C#에서 현재 메서드 이름 가져오기
-
C#
에서MethodBase.GetCurrentMethod()
를 사용하여 현재 메서드 이름 얻기 -
C#
에서Reflection
을 사용하여 현재 메서드 이름 얻기 -
C#
에서StackTrace
클래스로 현재 메소드 이름 얻기 -
C#
에서nameof
연산자를 사용하여 현재 메서드 이름 얻기
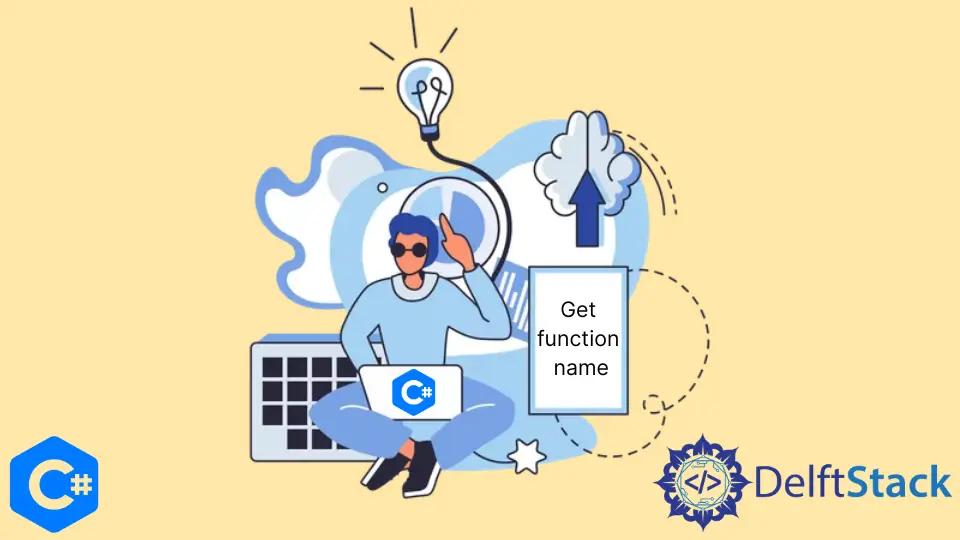
이 기사에서는 C#을 활용하여 실행 중인 현재 메서드의 이름을 가져오는 방법을 배웁니다. C#에는 현재 실행 중인 메서드의 이름을 가져오는 방법이 두 가지 이상 있습니다.
유사하게 작동하는 다음 절차는 아래에서 설명, 논의 및 수행됩니다.
C#
에서 MethodBase.GetCurrentMethod()
를 사용하여 현재 메서드 이름 얻기
현재 메서드를 나타내려면 MethodBase
개체를 활용해야 합니다.
실행 중인 메서드 내부에서 GetCurrentMethod()
를 사용하여 현재 메서드에 대한 정보를 얻을 수 있습니다. 현재 실행 중인 메서드를 반영하는 MethodBase
개체를 가져오기 위해 MethodBase.GetCurrentMethod()
함수를 사용할 수 있습니다.
먼저 코드에서 나중에 사용되는 기능에 액세스할 수 있도록 라이브러리를 가져옵니다.
using System;
using System.Diagnostics;
using System.Reflection;
그런 다음 Main
메서드 내에서 GetCurrentMethod()
함수를 사용하여 현재 메서드의 이름을 확인할 문자열 유형 변수를 초기화합니다. 그런 다음 콘솔에 함수 이름을 출력합니다.
string getName = MethodBase.GetCurrentMethod().Name;
Console.WriteLine("Current method is " + getName);
소스 코드:
using System;
using System.Diagnostics;
using System.Reflection;
class CurrentMethodName {
public static void Main() {
string getName = MethodBase.GetCurrentMethod().Name;
Console.WriteLine("Current method is " + getName);
}
}
출력:
Current method is Main
현재 실행 중인 메서드가 제네릭 유형에 정의된 경우(MethodBase.ContainsGenericParameters
가 true
를 반환함) GetCurrentMethod
에 의해 제공된 MethodInfo
는 제네릭 유형 정의에서 파생됩니다. 따라서 호출될 때 메서드에 전달된 매개 변수 유형을 나타내지 않습니다.
‘GetCurrentMethod’는 현재 실행 중인 메서드가 일반인 경우 일반 메서드 설명을 반환합니다. 제네릭 메서드가 제네릭 형식에 지정된 경우 제네릭 형식의 사양에서 MethodInfo
를 받습니다.
C#
에서 Reflection
을 사용하여 현재 메서드 이름 얻기
‘Reflection’은 시작하는 간단한 방법일 수 있습니다. reflection
네임스페이스는 메소드 정보를 가져오기 위해 GetCurrentMethod
를 노출하는 MethodBase
클래스를 제공합니다.
System.Reflection.MethodBase.GetCurrentMethod().Name;
다음은 Reflection
메서드의 전체 소스 코드입니다.
소스 코드:
using System;
class CurrentMethodName {
private static void Main(string[] args) {
var getName = System.Reflection.MethodBase.GetCurrentMethod().Name;
Console.WriteLine("Current method is " + getName);
}
}
출력:
Current method is Main
C#
에서 StackTrace
클래스로 현재 메소드 이름 얻기
메서드 이름을 가져오는 또 다른 옵션은 StackTrace
메서드와 스택 프레임을 사용하여 메서드 정보를 가져오는 것입니다. 메서드의 이름은 StackTrace
GetFrame()
함수가 반환한 프레임의 GetMethod()
속성을 통해 얻을 수 있습니다.
먼저 코드 내부의 StackTrace
클래스에 액세스하기 위해 라이브러리를 가져옵니다.
using System;
using System.Diagnostics;
stackTrace
라는 변수에 StackTrace
개체를 설정한 다음 Main()
함수에서 해당 개체를 사용합니다. StackTrace
를 통해 GetFrame
기능으로 프레임을 가져올 getFrame
변수를 만듭니다.
getName
이라는 변수를 만들고 GetMethod
클래스의 Name
특성을 사용하여 활성 메서드의 이름을 검색하는 책임을 변수에 할당합니다.
var stackTrace = new StackTrace();
var getFrame = stackTrace.GetFrame(0);
var getName = getFrame.GetMethod().Name;
소스 코드:
using System;
using System.Diagnostics;
class CurrentMethodName {
private static void Main(string[] args) {
var stackTrace = new StackTrace();
var getFrame = stackTrace.GetFrame(0);
var getName = getFrame.GetMethod().Name;
Console.WriteLine("Current method is " + getName);
}
}
출력:
Current method is Main
C#
에서 nameof
연산자를 사용하여 현재 메서드 이름 얻기
표현식에서 nameof
키워드를 사용하면 문자열 상수가 변수, 유형 또는 멤버의 이름으로 설정됩니다. nameof
표현식은 빌드 시에만 평가되기 때문에 런타임에 영향을 미치지 않습니다.
nameof
라는 식을 사용하여 인수 확인 코드를 유지 관리하기 쉽게 만들 수 있습니다.
이 접근 방식은 다음을 사용하지 않는 기본 제공 키워드를 기반으로 합니다.
reflection
네임스페이스를 첨부하는using
문,using System.Diagnostics;
를 첨부할 필요가 없습니다. 및사용 System.Reflection;
- 기존 코드를 반영하는 추가 클래스.
소스 코드:
using System;
class CurrentMethodName {
private static void Unique() {
string name = nameof(Unique);
Console.WriteLine("Current method is " + name);
}
public static void Main(string[] args) {
Unique();
}
}
출력:
Current method is Unique
nameof
연산자는 C# 버전 6 이상에서 사용할 수 있습니다.I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn