C#에서 함수 종료
-
break
문을 사용하여C#
에서 함수 종료 -
continue
문을 사용하여C#
에서 함수 종료 -
goto
문을 사용하여C#
에서 함수 종료 -
return
문을 사용하여C#
에서 함수 종료 -
throw
문을 사용하여C#
에서 함수 종료
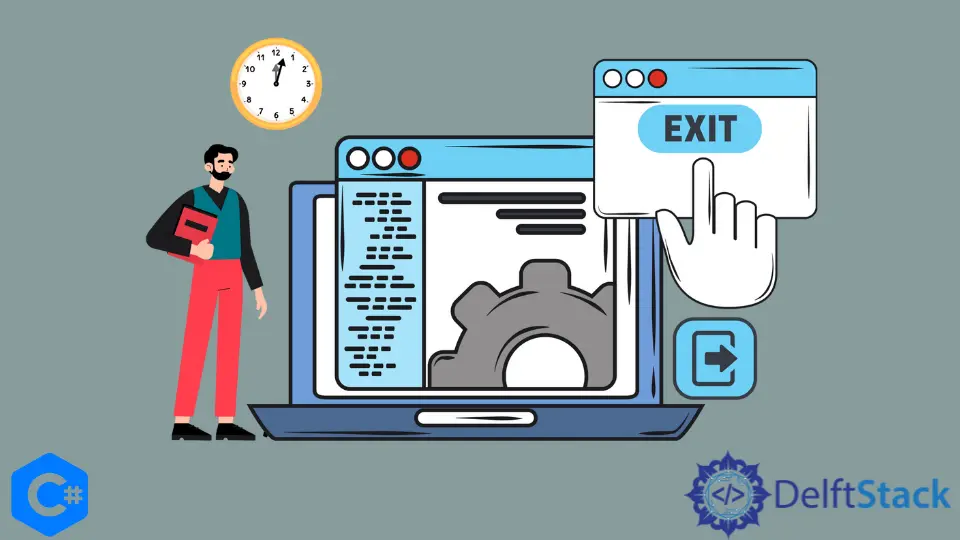
이 기사에서는 C#에서 함수를 종료하는 방법을 소개합니다.
점프 문은 일반적으로 프로그램 실행의 흐름을 제어하는 데 사용됩니다. 즉, 점프 문은 실행 중인 프로그램의 한 지점에서 다른 지점으로 무조건 제어를 전달합니다.
추가 논의는 이 참조를 통해 가능합니다.
다음은 Jump 문으로 분류된 C#의 5개 문입니다.
break
진술;continue
진술;goto
진술;return
진술;throw
진술.
break
문을 사용하여 C#
에서 함수 종료
break
문은 루프가 있는 위치에서 루프를 중지합니다. 그런 다음 사용 가능한 경우 제어는 종료된 명령문 다음에 오는 명령문으로 전달됩니다.
break
문이 중첩 루프에 있으면 break 문이 포함된 루프만 종료합니다.
예시:
// C# program to illustrate the
// use of break statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int number in Numbers) {
// print only the first 10 numbers
if (number > 10) {
break;
}
Console.Write($"{number} ");
}
}
}
출력:
1 2 3 4 5 6 7 8 9 10
continue
문을 사용하여 C#
에서 함수 종료
continue
문은 특정 조건이 참일 때 코드 블록의 실행을 건너뜁니다. break
문과 달리 continue
문은 제어를 루프의 시작 부분으로 전송합니다.
다음은 foreach
메서드를 사용하는 코드의 예입니다.
// C# program to illustrate the
// use of continue statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int oddNumber in Numbers) {
// print only the odd numbers 10 numbers
if (oddNumber % 2 == 0) {
continue;
}
Console.Write($"{oddNumber} ");
}
}
}
출력:
1 3 5 7 9 11 13 15 17 19
goto
문을 사용하여 C#
에서 함수 종료
goto
문을 사용하여 프로그램에서 레이블이 지정된 문으로 제어를 전송합니다. 레이블은 goto
문 앞에 있는 유효한 식별자여야 합니다.
즉, 레이블의 코드를 강제로 실행합니다.
아래 예에서 goto
문은 사례 5를 강제로 실행합니다.
// C# program to illustrate the
// use of goto statement
using System;
class Test {
// Main Method
static public void Main() {
int age = 18;
switch (age) {
case 5:
Console.WriteLine("5yrs is less than the recognized age for adulthood");
break;
case 10:
Console.WriteLine("Age 10 is still underage");
break;
case 18:
Console.WriteLine("18yrs! You are now an adult and old enough to drink");
// goto statement transfer
// the control to case 5
goto case 5; default:
Console.WriteLine("18yrs is the recognized age for adulthood");
break;
}
}
}
출력:
18yrs! You are now an adult and old enough to drink
5yrs is less than the recognized age for adulthood
return
문을 사용하여 C#
에서 함수 종료
return
문은 나타나는 함수 실행을 종료한 다음 사용 가능한 경우 호출 메서드의 결과에 대한 제어를 반환합니다. 그러나 함수에 값이 없으면 return
문은 표현식 없이 사용됩니다.
예시:
// C# program to illustrate the
// use of return statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int number in Numbers) {
// print only the first 10 numbers
if (number > 10) {
return;
}
return;
Console.Write($"{number} ");
}
}
}
출력:
No output
throw
문을 사용하여 C#
에서 함수 종료
예외는 오류가 발생했거나 프로그램 실행을 변경했음을 나타냅니다. throw
문은 new
키워드를 사용하여 유효한 Exception class
의 개체를 생성합니다.
모든 예외 클래스에는 Stacktrace 및 Message 속성이 있습니다.
유효한 예외는 Exception 클래스에서 파생되어야 합니다. 유효한 예외 클래스에는 ArgumentException
, InvalidOperationException
, NullReferenceException
및 IndexOutOfRangeException
이 포함됩니다.
추가 논의는 이 참조를 통해 가능합니다.
예시:
// C# program to illustrate the
// use of throw statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int number in Numbers) {
// using try catch block to
// handle the Exception
try {
// print only the first 10 numbers
if (number > 10) {
Console.WriteLine();
throw new NullReferenceException("Number is greater than 10");
}
Console.Write($"{number} ");
} catch (Exception exp) {
Console.WriteLine(exp.Message);
return;
}
}
}
}
출력:
1 2 3 4 5 6 7 8 9 10
Number is greater than 10