Increment and Decrement Counter in C#
- Create and Design a Windows Forms Application Using Visual Studio
- Implement an Increment and Decrement Counter in C#
- Conclusion
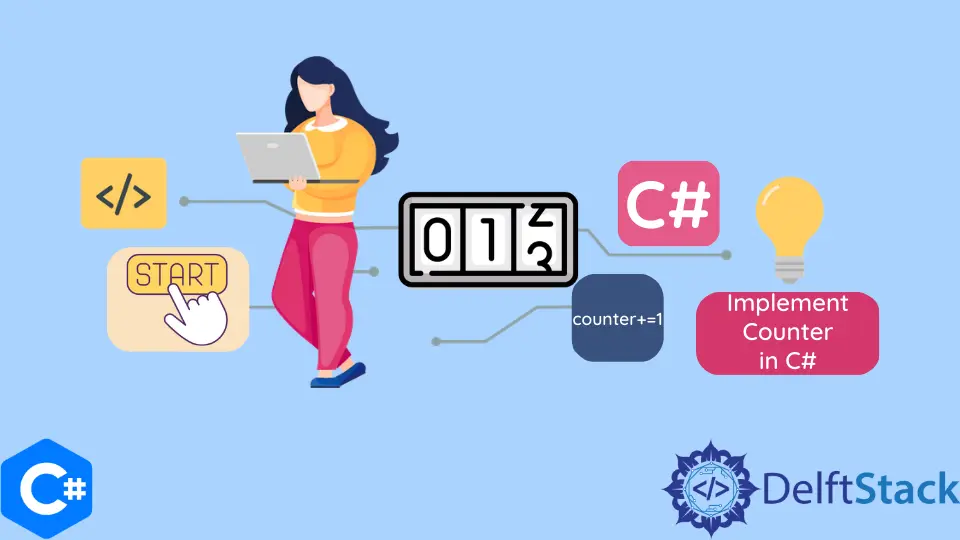
Counters are a common feature in many applications, allowing users to easily track and manipulate numeric values.
This tutorial guides you in creating an increment and decrement counter in C# using Windows Forms, a graphical user interface (GUI) framework for building Windows applications.
By the end of this tutorial, you’ll have a working counter application with two buttons—one for incrementing and the other for decrementing the count.
Create and Design a Windows Forms Application Using Visual Studio
Before we start, ensure you have Visual Studio or another C# development environment installed on your computer.
-
Launch Visual Studio.
-
Go to
File
>New
>Project
. -
In the
Create a new project
dialog, selectWindows Forms App (.NET Framework)
. -
Choose a name for your project and specify the location where you want to save it.
-
Click the
Create
button to create the project. -
Visual Studio will generate a new Windows Forms project with a default form (
Form1
).
We will design the user interface (UI) for our counter application. We’ll add two buttons—one for incrementing and the other for decrementing the count—and a label to display the current count.
-
Open
Form1.cs
by double-clicking it in theSolution Explorer
. -
In the form designer, add two buttons and one label to your form.
-
Rename the buttons to something more descriptive. Right-click on each button, select
Properties
, and change theName
property toincrementButton
for the increment button anddecrementButton
for the decrement button. -
Change the buttons’ text to
Increment
andDecrement
by modifying theText
property in theProperties
window. -
Change the initial text of the label to
0
to represent the initial count.
The form should look like the form below.
Implement an Increment and Decrement Counter in C#
Now that we’ve designed our UI, let’s add the functionality to our buttons. We’ll write the C# code to increment and decrement the count when these buttons are clicked.
Double-click the Increment
button to create a click event handler. This will automatically generate a method in your code.
Inside the incrementButton_Click
method, write the following code to increment the count.
private void incrementButton_Click(object sender, EventArgs e) {
// Increment the count
int currentCount = int.Parse(countLabel.Text);
currentCount++;
countLabel.Text = currentCount.ToString();
}
Double-click the Decrement
button to create a click event handler. In the decrementButton_Click
method, write the following code to decrement the count.
private void decrementButton_Click(object sender, EventArgs e) {
// Decrement the count if it's greater than 0
int currentCount = int.Parse(countLabel.Text);
if (currentCount > 0) {
currentCount--;
countLabel.Text = currentCount.ToString();
}
}
The above code converts the text from the label into an integer, increments or decrements it, and then updates the label with the new count.
Run the application, and the form will appear. You can click the Increment
and Decrement
buttons to see the count change accordingly.
Increment:
Decrement:
Conclusion
This tutorial taught you how to create a simple counter application in C# with a graphical user interface using Windows Forms. You designed the user interface with buttons and a label and implemented the logic for incrementing and decrementing a counter variable based on button clicks.
This project serves as a basic introduction to GUI development in C# and can be extended and customized to meet more advanced requirements in your future projects.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn