C# でカウンターを実装する
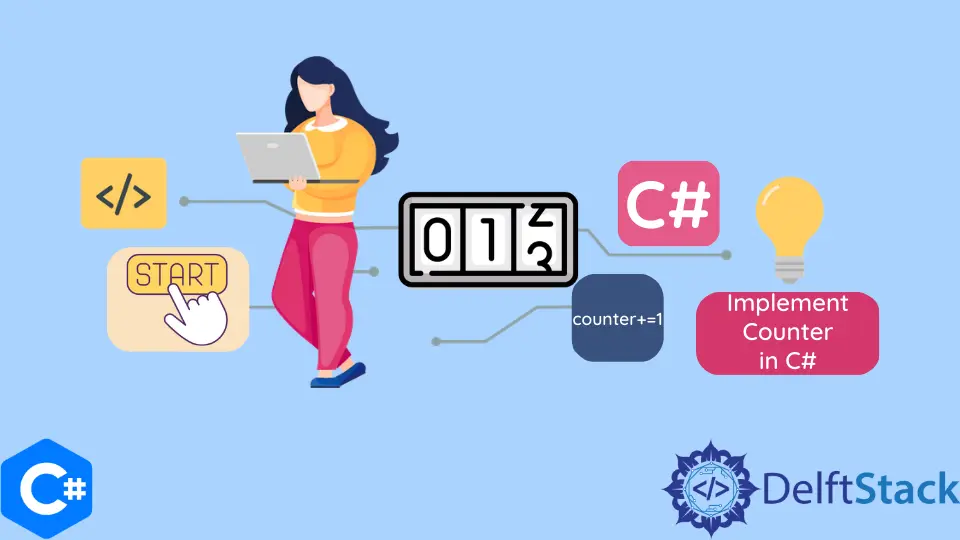
このガイドでは、2つのボタンを使用して C# でインクリメントおよびデクリメントカウンターを作成する方法を説明します。たとえば、A と B の 2つのボタンがあるとします。
A と B を押すとカウンターがインクリメントされ、A と B を押すとそれぞれカウンターがデクリメントされます。このガイドに飛び込んで、自分自身を学びましょう。
C#
でカウンターを実装する
この問題では、2つの変数が必要です。まず、カウンター用の変数が必要です。次に、最後に押されたボタンをチェックするための変数が必要です。
それをどのように行うか見てみましょう。最初に、イベントデリゲートを作成する必要があるため、ボタンがクリックされるたびに特定のイベントが発生する必要があります。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace counter {
class MyForm : Form {
// Window From Code
Button buttonA;
Button buttonB;
void InitializeComponent() {
buttonA.Clicked += Button_A_Click; // btn_Clicked Event.. delgates..
buttonB.Clicked += Button_B_Click;
}
}
}
上記のコードでは、2つのボタンを作成し、イベントデリゲートを割り当てました。関数 Button_A_Click
は、buttonA
がクリックされるたびに実行されます。
次に、2つの変数が必要です。見てください。
Button LastPressed = null; // Track which button was last pressed.
int counter = 0; // initializing Counter Value
LastPressed
は最後に押されたボタンを追跡し、カウンターはインクリメントとデクリメントを追跡します。次に必要なのは、これらすべてを追跡する 2つの関数を用意することです。
void Button_A_Click(object source, EventArgs e) {
if (LastPressed == buttonB) // verifying which button is being pressed.
{
// button B was pressed first, so decrement the counter
--counter; // decrementing...
// reset state for the next button press
LastPressed = null; // again button set to null for next tracking..
} else {
LastPressed = buttonA; // assging which button was pressed.
}
}
void Button_B_Click(object source, EventArgs e) {
if (LastPressed == buttonA) {
// buttonA was pressed 1st, so increment the counter
++counter;
// reset state for the next button press
LastPressed = null;
} else {
LastPressed = buttonB;
}
}
buttonA
がクリックされると、プログラムは LastPressed
の値をチェックします。buttonB
の場合、カウンターはデクリメントします。何もない場合は、buttonA
が LastPressed
に割り当てられます。
同じことが buttonB
にも当てはまります。LastPressed
が A の場合、カウンターがインクリメントされます。それ以外の場合は、buttonB
が割り当てられます。これが、C# でカウンターをインクリメントおよびデクリメントするプログラムを作成する方法です。
以下は完全なコードです。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace counter {
class MyForm : Form {
// Window From Code
Button buttonA;
Button buttonB;
void InitializeComponent() {
buttonA.Clicked += Button_A_Click; // btnB_Clicked Event.. delgates..
buttonB.Clicked += Button_B_Click;
}
Button LastPressed = null; // Track which button was last pressed.
int counter = 0; // initializing Counter Value
void Button_A_Click(object source, EventArgs e) {
if (LastPressed == buttonB) // verifying which button is being pressed.
{
// button B was pressed first, so decrement the counter
--counter; // decrementing...
// reset state for the next button press
LastPressed = null; // again button set to null for next tracking..
} else {
LastPressed = buttonA; // assging which button was pressed.
}
}
void Button_B_Click(object source, EventArgs e) {
if (LastPressed == buttonA) {
// buttonA was pressed 1st, so increment the counter
++counter;
// reset state for the next button press
LastPressed = null;
} else {
LastPressed = buttonB;
}
}
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn