C#에서 카운터 구현
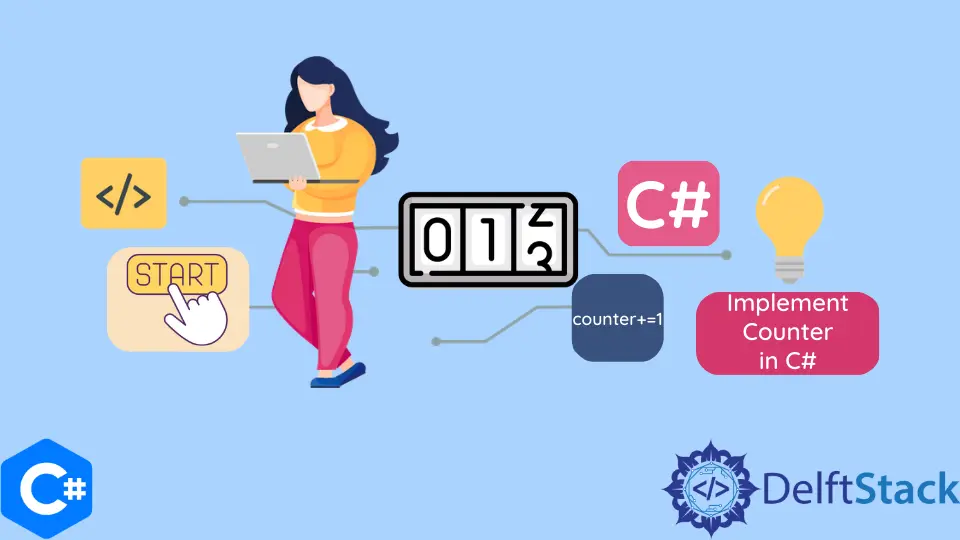
이 가이드에서는 두 개의 버튼을 사용하여 C#에서 카운터를 증가 및 감소시키는 방법을 볼 것입니다. 예를 들어 A와 B라는 두 개의 버튼이 있는 경우.
A와 B를 누르면 카운터가 증가하고 A와 B를 누르면 카운터가 감소해야 합니다. 이 가이드를 자세히 살펴보고 스스로 배우도록 합시다.
C#
에서 카운터 구현
이 문제에서는 두 개의 변수가 필요합니다. 먼저 카운터에 대한 변수가 필요하고 두 번째로 마지막으로 눌린 버튼을 확인하기 위한 변수가 필요합니다.
어떻게 하는지 봅시다. 처음에는 이벤트 대리자를 생성해야 하므로 버튼을 클릭할 때마다 특정 이벤트가 발생해야 합니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace counter {
class MyForm : Form {
// Window From Code
Button buttonA;
Button buttonB;
void InitializeComponent() {
buttonA.Clicked += Button_A_Click; // btn_Clicked Event.. delgates..
buttonB.Clicked += Button_B_Click;
}
}
}
위의 코드에서는 두 개의 버튼을 만들고 이벤트 대리자를 할당했습니다. Button_A_Click
기능은 buttonA
를 클릭할 때마다 실행됩니다.
다음으로 두 개의 변수가 필요합니다. 구경하다.
Button LastPressed = null; // Track which button was last pressed.
int counter = 0; // initializing Counter Value
LastPressed
는 마지막으로 눌린 버튼을 추적하고 카운터는 증가 및 감소를 추적합니다. 다음으로 필요한 것은 이 모든 것을 추적하는 두 개의 함수를 갖는 것입니다.
void Button_A_Click(object source, EventArgs e) {
if (LastPressed == buttonB) // verifying which button is being pressed.
{
// button B was pressed first, so decrement the counter
--counter; // decrementing...
// reset state for the next button press
LastPressed = null; // again button set to null for next tracking..
} else {
LastPressed = buttonA; // assging which button was pressed.
}
}
void Button_B_Click(object source, EventArgs e) {
if (LastPressed == buttonA) {
// buttonA was pressed 1st, so increment the counter
++counter;
// reset state for the next button press
LastPressed = null;
} else {
LastPressed = buttonB;
}
}
buttonA
를 클릭하면 프로그램이 LastPressed
의 값을 확인합니다. buttonB
이면 카운터가 감소합니다. 아무것도 없으면 buttonA
가 LastPressed
에 할당됩니다.
buttonB
도 마찬가지입니다. LastPressed
가 A이면 카운터가 증가합니다. 그렇지 않으면 buttonB
가 할당됩니다. 이것이 C#에서 카운터를 증가 및 감소시키는 프로그램을 만드는 방법입니다.
다음은 전체 코드입니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace counter {
class MyForm : Form {
// Window From Code
Button buttonA;
Button buttonB;
void InitializeComponent() {
buttonA.Clicked += Button_A_Click; // btnB_Clicked Event.. delgates..
buttonB.Clicked += Button_B_Click;
}
Button LastPressed = null; // Track which button was last pressed.
int counter = 0; // initializing Counter Value
void Button_A_Click(object source, EventArgs e) {
if (LastPressed == buttonB) // verifying which button is being pressed.
{
// button B was pressed first, so decrement the counter
--counter; // decrementing...
// reset state for the next button press
LastPressed = null; // again button set to null for next tracking..
} else {
LastPressed = buttonA; // assging which button was pressed.
}
}
void Button_B_Click(object source, EventArgs e) {
if (LastPressed == buttonA) {
// buttonA was pressed 1st, so increment the counter
++counter;
// reset state for the next button press
LastPressed = null;
} else {
LastPressed = buttonB;
}
}
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn