Implementar un contador en C#
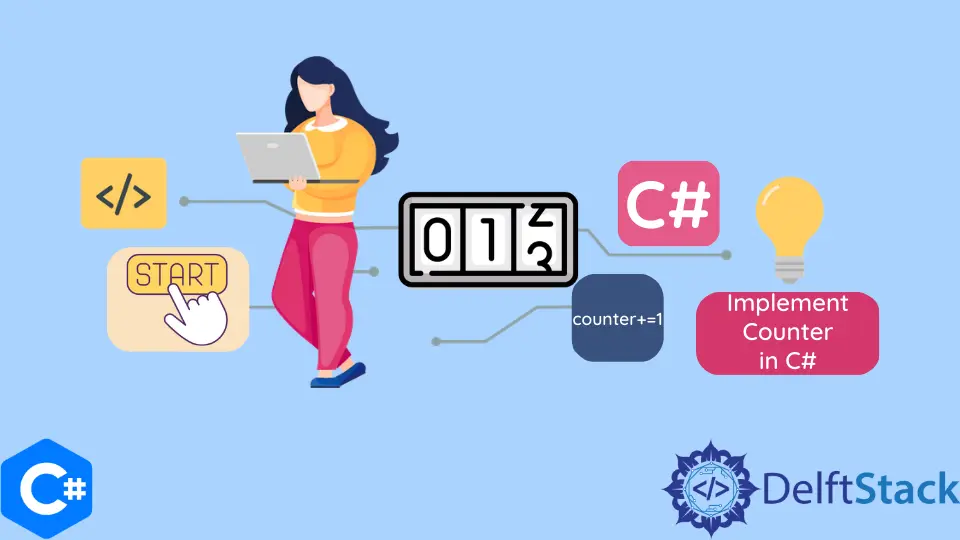
En esta guía, veremos cómo hacer un contador de incrementos y decrementos en C# usando dos botones. Por ejemplo, si hay dos botones, A y B.
Presionar A y B debería incrementar el contador, y presionar A y B debería disminuir el contador, respectivamente. Sumerjámonos en esta guía y aprendamos nosotros mismos.
Implementar el contador en C#
En este problema, requerimos dos variables. Primero, necesitamos una variable para el contador y, segundo, necesitamos una para verificar el último botón presionado.
Vamos a ver cómo vamos a hacer eso. Al principio, necesitaremos crear delegados de eventos, por lo que debería ocurrir un determinado evento cada vez que se haga clic en un botón.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace counter {
class MyForm : Form {
// Window From Code
Button buttonA;
Button buttonB;
void InitializeComponent() {
buttonA.Clicked += Button_A_Click; // btn_Clicked Event.. delgates..
buttonB.Clicked += Button_B_Click;
}
}
}
En el código anterior, creamos dos botones y asignamos delegados de eventos. La función Button_A_Click
se ejecutará cada vez que se haga clic en un buttonA
.
A continuación, necesitamos tener dos variables. Echar un vistazo.
Button LastPressed = null; // Track which button was last pressed.
int counter = 0; // initializing Counter Value
El LastPressed
rastreará qué botón se presionó por última vez, y el contador rastreará el incremento y la disminución. Lo siguiente que necesitamos es tener dos funciones rastreando todo esto.
void Button_A_Click(object source, EventArgs e) {
if (LastPressed == buttonB) // verifying which button is being pressed.
{
// button B was pressed first, so decrement the counter
--counter; // decrementing...
// reset state for the next button press
LastPressed = null; // again button set to null for next tracking..
} else {
LastPressed = buttonA; // assging which button was pressed.
}
}
void Button_B_Click(object source, EventArgs e) {
if (LastPressed == buttonA) {
// buttonA was pressed 1st, so increment the counter
++counter;
// reset state for the next button press
LastPressed = null;
} else {
LastPressed = buttonB;
}
}
Si se hace clic en buttonA
, el programa verificará el valor en LastPressed
. Si es botónB
, el contador decrementará; si no es nada, buttonA
se asignará a LastPressed
.
Lo mismo ocurre con botónB
; si el LastPressed
fuera A, incrementaría el contador; de lo contrario, se asignará buttonB
. Así es como se crea un programa para incrementar y disminuir un contador en C#.
El siguiente es el código completo.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace counter {
class MyForm : Form {
// Window From Code
Button buttonA;
Button buttonB;
void InitializeComponent() {
buttonA.Clicked += Button_A_Click; // btnB_Clicked Event.. delgates..
buttonB.Clicked += Button_B_Click;
}
Button LastPressed = null; // Track which button was last pressed.
int counter = 0; // initializing Counter Value
void Button_A_Click(object source, EventArgs e) {
if (LastPressed == buttonB) // verifying which button is being pressed.
{
// button B was pressed first, so decrement the counter
--counter; // decrementing...
// reset state for the next button press
LastPressed = null; // again button set to null for next tracking..
} else {
LastPressed = buttonA; // assging which button was pressed.
}
}
void Button_B_Click(object source, EventArgs e) {
if (LastPressed == buttonA) {
// buttonA was pressed 1st, so increment the counter
++counter;
// reset state for the next button press
LastPressed = null;
} else {
LastPressed = buttonB;
}
}
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn