Character Input in While Loop Using C++
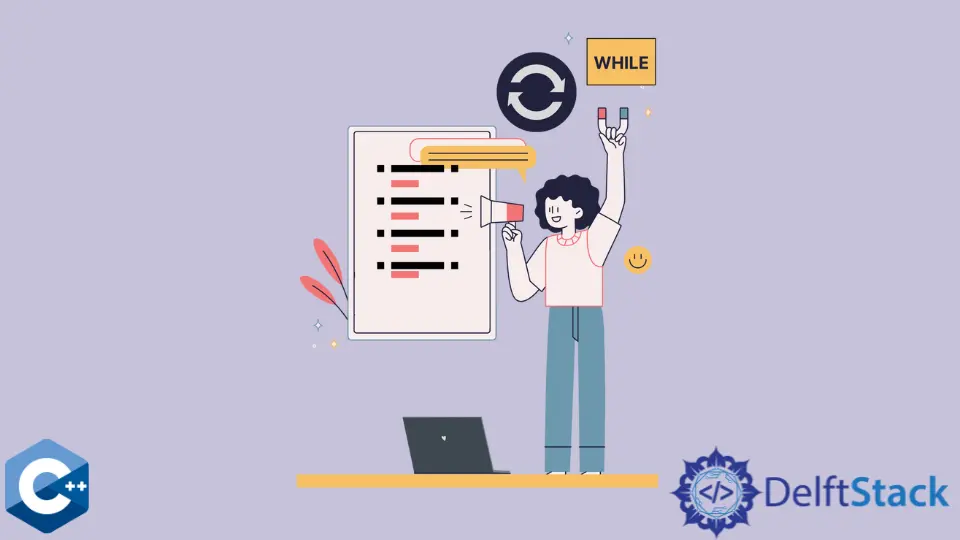
This trivial guide will discuss handing inputs in C++. Moreover, we will look into the issue of tackling invalid inputs by the user. Lastly, we will discuss how the input can be taken from the user using the while
loop.
Firstly, one should know the procedure to take input data from the user in C++. The iostream
library in C++ provides us with the function cin
. It will take the input from the user and store the value in the variable that is used.
Code Example:
int x;
cin >> x;
In this code, x
is an integer
variable declared at line no 1, and input is taken at line no 2. cin
will prompt the user to enter the data and store that data in the variable x
. This value must be an integer
value; otherwise, the value will be partially saved in x
or will not save.
Use the while
Loop With the cin
Function in C++
Consider another example where we want to take multiple inputs until the user enters some invalid data. If some invalid data is entered, the input should be closed.
Code:
int main() {
int a;
;
while (cin >> a) {
cout << "You entered the correct input value" << endl;
}
cout << "Sorry, you have entered wrong type of data." << endl;
}
Output:
The user is prompted to enter a value, and we entered the value 2
, which is an int
value. So, the loop continues to iterate, and again user is prompted.
After three attempts, we entered some characters (i.e., abc
) that are not of the int
type. This causes the standard input stream (associated with cin
) to destroy, and the expression cin >> a
returns false
.
Therefore, the loop breaks and control is shifted to the line outside the loop. We can restrict some invalid data from being entered in this way.
Another way to achieve the same task is shown in this next example.
Code:
int main() {
int a;
;
while (cin) {
cin >> a;
cout << "You entered the correct input value" << endl;
}
cout << "Sorry, you have entered a wrong type of data." << endl;
}
We should know that cin
has four states, i.e. good
, bad
, fail
, and EOF
. cin
is in its good
state when running the code above.
We have used cin
as a loop condition. When the code starts executing, cin
will be in a good
state and return true
.
Hence, the while
loop will execute and prompt the user to input a value for a
.
Output:
As soon as the incorrect data is entered, the loop doesn’t break immediately, but after one iteration.
Once the standard input stream associated with cin
goes into an error state
, no further inputs can be taken until the state is not reset to good state
. We can clear invalid state of cin
using cin.clear()
function and cin.ignore(numeric_limits<streamsize>::max(), '\n')
function can be used to clear invalid input from the stream.
This will enable us to retake the inputs from the user without breaking the execution.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn