The continue Statement in C++
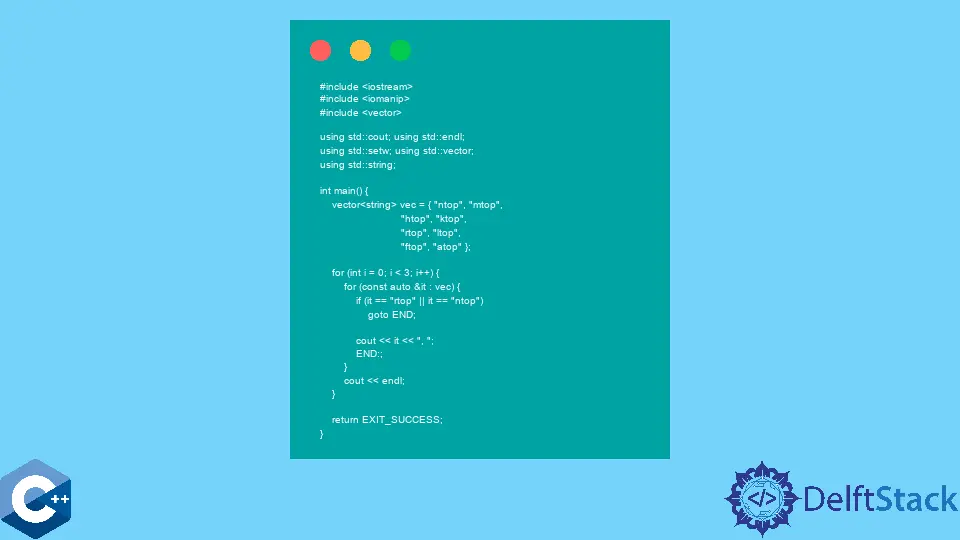
This article will explain how to utilize the continue
statement in C++.
Use the continue
Statement to Skip the Remaining Portion of the Loop Body
The continue
statement is utilized in conjunction with iteration statements to manipulate the loop-dependent block execution. Namely, once the continue
statement is reached in the loop, the following statements are skipped over, and the control moves to the condition evaluation step. If the condition is true, the loop starts from a new iteration cycle as usual.
Note that continue
can only be included in a code block enclosed by at least one of the loop statements: for
, while
, do...while
, or range-based for
. Suppose we have multiple nested loops, and the continue
statement is included in the inner one. The skipping behavior affects only the inner loop while the outer one behaves as usual.
In the following example, we demonstrate two for
loops, the inner of which iterates through the vector
of strings. Notice that the continue
statement is specified at the beginning of the loop body, and it essentially controls if the following statement gets executed. Thus, the string values with rtop
and ntop
are not displayed on output, and the outer loop executes all of its cycles.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
int main() {
vector<string> vec = {"ntop", "mtop", "htop", "ktop",
"rtop", "ltop", "ftop", "atop"};
for (int i = 0; i < 3; i++) {
for (const auto &it : vec) {
if (it == "rtop" || it == "ntop") continue;
cout << it << ", ";
}
cout << endl;
}
return EXIT_SUCCESS;
}
Output:
mtop, htop, ktop, ltop, ftop, atop,
mtop, htop, ktop, ltop, ftop, atop,
mtop, htop, ktop, ltop, ftop, atop,
Alternatively, we can implement the same behavior of the previous code snippet using the goto
statement instead of continue
. Remember that goto
acts as an unconditional jump to the given line in the program, and it should not be used to jump over variable initialization statements. In this case, the empty statement can be marked with the END
label so that goto
will move the execution to the given label.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
int main() {
vector<string> vec = {"ntop", "mtop", "htop", "ktop",
"rtop", "ltop", "ftop", "atop"};
for (int i = 0; i < 3; i++) {
for (const auto &it : vec) {
if (it == "rtop" || it == "ntop") goto END;
cout << it << ", ";
END:;
}
cout << endl;
}
return EXIT_SUCCESS;
}
The continue
statement can be considered bad practice by some coding guidelines, making the code a bit harder to read. The same recommendation is often given to excessive usage of goto
statements. Still, one can utilize these constructs when the given problem can internalize the readability cost and offer easier implementation using these statements. The next code example shows the basic usage of the continue
statement in a while
loop.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
int main() {
vector<string> vec = {"ntop", "mtop", "htop", "ktop",
"rtop", "ltop", "ftop", "atop"};
while (!vec.empty()) {
if (vec.back() == "atop") {
vec.pop_back();
continue;
}
cout << vec.back() << ", ";
vec.pop_back();
}
cout << "\nsize = " << vec.size() << endl;
return EXIT_SUCCESS;
}
Output:
ftop, ltop, rtop, ktop, htop, mtop, ntop,
size = 0
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook