Nested Loops in C++
-
Use Nested
for
Loop to Initialize Two-Dimensional Matrix in C++ -
Use Nested
while
Loop to Initialize Two-Dimensional Matrix in C++ -
Use Nested
do...while
Loop to Initialize Two-Dimensional Matrix in C++
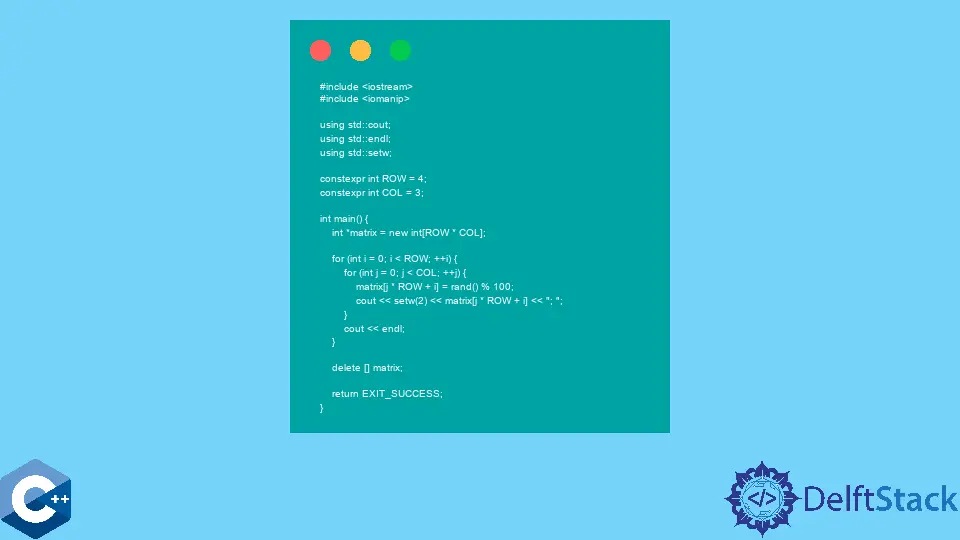
This article will describe how to utilize different nested loops in C++.
Use Nested for
Loop to Initialize Two-Dimensional Matrix in C++
Loops are known as control flow statements, which generally modify the program counter and force the executing CPU to move to a different(usually a non-sequential) instruction in the program. The program counter is a register in the CPU core that stores a running program’s next instruction.
Let’s think in terms of assembly language-level representation of the program, where each statement corresponds to a single machine instruction. The code executes sequentially, and the CPU fetches the following instruction unless instructed otherwise (loosely speaking, as contemporary CPU cores are massively optimized machines that can fetch multiple instructions and often from non-sequential locations in the program). So, we need to indicate in the assembly language with special instructions to transfer the execution to a different part of the program if we want to implement a loop.
Now, let’s consider a C++ language syntax and how to implement different control flow statements like loops.
One of the most common loop statements in programming languages is for
and while
loops. The for
loop has the header part that includes three-part statements and controls the execution of the loop body. Multiple for
loop statements can be nested inside each other, forming a useful construct for many programming tasks.
This article demonstrates an example of initializing a two-dimensional matrix using nested for
loops. Each nested loop level deals with a single row of the matrix, so we have two levels in this example. Notice that we also print the elements after the random integer is assigned to the given position. The latter step will be done separately in real-world scenarios, but this code sample is for educational purposes only.
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
constexpr int ROW = 4;
constexpr int COL = 3;
int main() {
int *matrix = new int[ROW * COL];
for (int i = 0; i < ROW; ++i) {
for (int j = 0; j < COL; ++j) {
matrix[j * ROW + i] = rand() % 100;
cout << setw(2) << matrix[j * ROW + i] << "; ";
}
cout << endl;
}
delete[] matrix;
return EXIT_SUCCESS;
}
Output:
83; 86; 77;
36; 93; 35;
86; 92; 14;
22; 62; 27;
Use Nested while
Loop to Initialize Two-Dimensional Matrix in C++
Alternatively, we can implement the previous code using nested while
loop statements. In this variant, we need to declare index variables i
and j
outside of the while
body and only initialize one of them in the same scope.
Note that the second variable y
- corresponding to the column position of the matrix is initialized to 0
each cycle of the outer while
loop. This ensures the inner loop is executed after processing the first row. We should also place incrementing statements for each index variable in respective loop scopes as the while
itself only has the conditional header which controls the iteration.
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
constexpr int ROW = 4;
constexpr int COL = 3;
int main() {
int *matrix = new int[ROW * COL];
int j, i = 0;
while (i < ROW) {
j = 0;
while (j < COL) {
matrix[j * ROW + i] = rand() % 100;
cout << setw(2) << matrix[j * ROW + i] << "; ";
j++;
}
i++;
cout << endl;
}
delete[] matrix;
return EXIT_SUCCESS;
}
Output:
83; 86; 77;
36; 93; 35;
86; 92; 14;
22; 62; 27;
Use Nested do...while
Loop to Initialize Two-Dimensional Matrix in C++
On the other hand, the C++ language also has the do...while
loop construct, which is suited for special cases of iteration, but we can rewrite the same code snippet using it.
The do...while
statement always executes the first iteration. So, this example will not suffer from this feature as we assume that the generated matrix has at least 1x1 dimensions.
The do...while
loop structure is almost the same as the while
example above, as these two have similar header format, which includes only conditional expression.
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
constexpr int ROW = 4;
constexpr int COL = 3;
int main() {
int *matrix = new int[ROW * COL];
int j, i = 0;
do {
j = 0;
do {
matrix[j * ROW + i] = rand() % 100;
cout << setw(2) << matrix[j * ROW + i] << "; ";
j++;
} while (j < COL);
i++;
cout << endl;
} while (i < ROW);
delete[] matrix;
return EXIT_SUCCESS;
}
Output:
83; 86; 77;
36; 93; 35;
86; 92; 14;
22; 62; 27;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook