Range-Based for Loop in C++
-
Use Range-Based
for
Loop to Print Elements ofstd::map
in C++ -
Use Range-Based
for
Loop to Print Members ofstruct
in C++
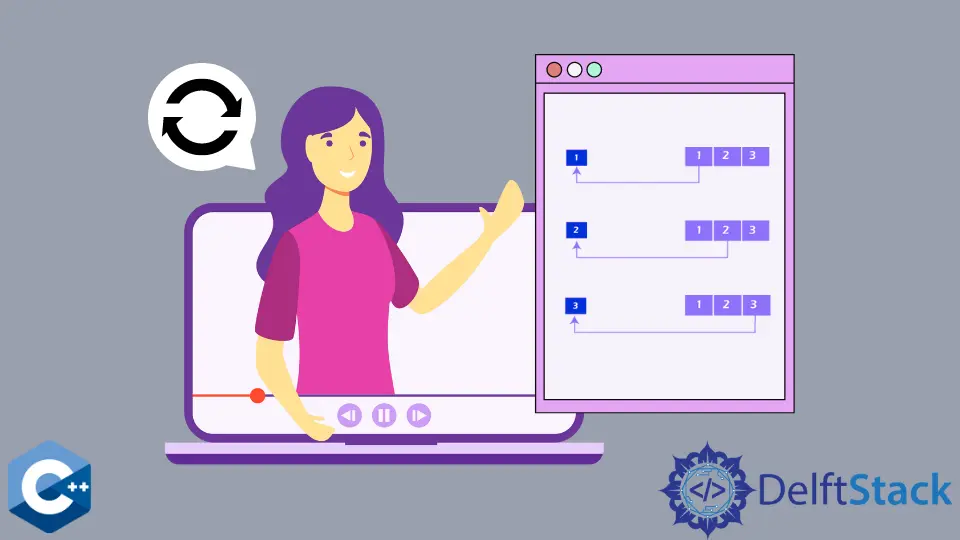
This article will demonstrate multiple methods about how to use a range-based for
loop in C++.
Use Range-Based for
Loop to Print Elements of std::map
in C++
The range-based for
loop is a more readable equivalent of the regular for
loop. It can be used to traverse the array or any other object that has begin
and end
member functions. Note that we use the auto
keyword and the reference to the element to access it. In this case, the item
refers to the single element of std::map
, which happens to be of type std::pair
. Thus, accessing the key-value pairs requires special dot notation to access them using first
/second
keywords.
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
map<int, string> fruit_map = {{
1,
"Apple",
},
{
2,
"Banana",
},
{
3,
"Mango",
},
{
4,
"Cocoa",
}};
for (auto &item : fruit_map) {
cout << "[" << item.first << "," << item.second << "]\n";
}
return EXIT_SUCCESS;
}
Output:
[1,Apple]
[2,Banana]
[3,Mango]
[4,Cocoa]
Alternatively, a range-based loop can access elements using structured binding notation and make the code block more concise. In the following example, we demonstrate such binding usage for accessing std::map
pairs.
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
map<int, string> fruit_map = {{
1,
"Apple",
},
{
2,
"Banana",
},
{
3,
"Mango",
},
{
4,
"Cocoa",
}};
for (const auto& [key, val] : fruit_map) {
cout << "[" << key << "," << val << "]\n";
}
return EXIT_SUCCESS;
}
Output:
[1,Apple]
[2,Banana]
[3,Mango]
[4,Cocoa]
Use Range-Based for
Loop to Print Members of struct
in C++
Structured binding can be extremely useful when the traversed elements represent the relatively bigger structures with multiple data members. As shown in the next example code, these members are declared as an identifier list and then referenced directly without the struct.member
notation. Note that most of the STL containers are traversable using the range-based for
loop.
#include <iostream>
#include <list>
using std::cin;
using std::cout;
using std::endl;
using std::list;
using std::string;
struct Person {
string name;
string surname;
int age;
};
int main() {
list<Person> persons = {{"T", "M", 11},
{"R", "S", 23},
{"J", "R", 43},
{"A", "C", 60},
{"D", "C", 97}};
for (const auto& [n, s, age] : persons) {
cout << n << "." << s << " - " << age << " years old" << endl;
}
return EXIT_SUCCESS;
}
Output:
T.M - 11 years old
R.S - 23 years old
J.R - 43 years old
A.C - 60 years old
D.C - 97 years old
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook