How to Initialize Vector of Structs in C++
- Initialize a Vector of Structs in C++ Using Initializer List Constructor
- Initialize a Vector of Structs in C++ Using the Range Constructor
- Initialize a Vector of Structs in C++ Using the Custom Constructor
-
Initialize a Vector of Structs in C++ Using
push_back
-
Initialize a Vector of Structs in C++ Using
emplace_back
- Conclusion
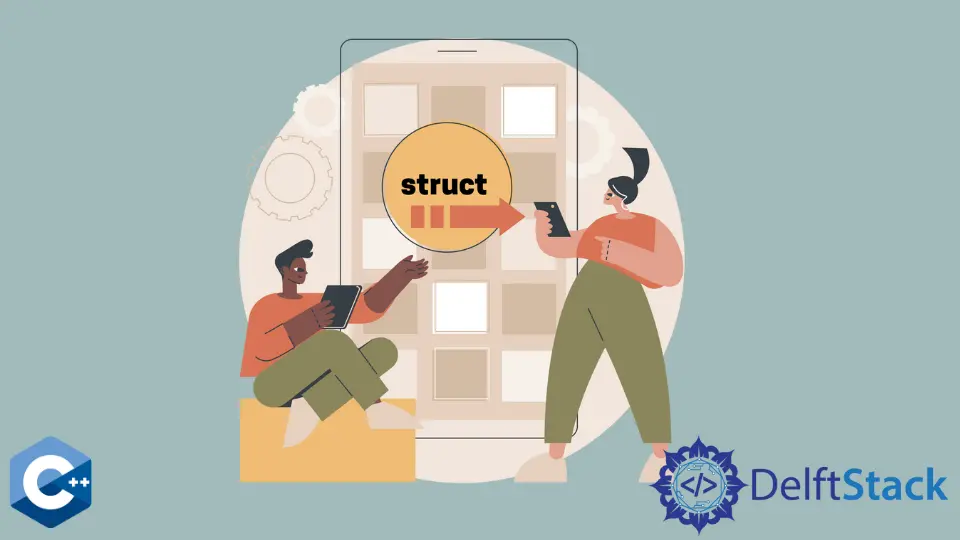
In C++ programming, working with structured data often involves the use of structs, allowing developers to organize related information within a single composite type. When it comes to managing collections of these structs, such as in a vector, the initialization process is a significant step in setting the foundation for effective data manipulation.
This article delves into the various methods available for initializing a vector of structs in C++. Whether you’re dealing with a fixed set of values, copying from an existing vector, or dynamically constructing instances during runtime, these initialization methods will allow you to write cleaner, more efficient code.
Initialize a Vector of Structs in C++ Using Initializer List Constructor
One concise way to initialize a vector of structs is by utilizing the initializer list constructor. This feature, introduced in C++11, allows you to initialize the elements of a vector directly with a list of values, simplifying the code and making it more readable.
The syntax for initializing a vector of structs using the initializer list constructor involves enclosing a list of comma-separated elements inside curly braces {}
. Each element corresponds to a struct instance, and the structs themselves are enclosed in an additional set of curly braces.
Here’s a general representation:
std::vector<StructType> vectorName = {
{value1, value2, ...}, {value3, value4, ...},
// Additional struct instances as needed
};
Here, StructType
represents the type of your struct, and vectorName
is the name you assign to your vector.
Let’s consider a scenario where we have a struct named Person
with attributes name
, surname
, and age
. We’ll initialize a vector of Person
structs using the initializer list constructor.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Person {
string name;
string surname;
int age;
};
int main() {
vector<Person> peopleVector = {{"John", "Cooper", 32},
{"Theo", "Parrot", 23},
{"Aun", "Chao", 43},
{"Vivien", "Bardot", 67}};
for (const auto &person : peopleVector) {
cout << "Name: " << person.name << endl
<< "Surname: " << person.surname << endl
<< "Age: " << person.age << endl;
cout << endl;
}
return EXIT_SUCCESS;
}
In the above code, firstly, we include necessary header files for input and output operations (<iostream>
), string manipulation (<string>
), and vector operations (<vector>
). We also use the using
directive to simplify our code, bringing the cout
, endl
, string
, and vector
into the current scope.
Next, we define a custom struct named Person
. This struct encapsulates information about an individual, having three attributes: name
and surname
as strings and age
as an integer.
Moving on to the main
function, we initialize a vector of Person
structs named peopleVector
using the initializer list constructor. This constructor allows us to populate the vector directly with instances of the Person
struct.
In this case, we provide four instances, each represented by a set of curly braces. Each set contains values for the name
, surname
, and age
attributes of a person.
Subsequently, we iterate over each element in peopleVector
using a range-based for
loop. The loop variable person
is a reference to each element in the vector, allowing us to access the attributes of the Person
struct.
Inside the loop, we use cout
statements to display the name
, surname
, and age
of each person on separate lines.
Output:
This output corresponds to the initialized vector of Person
structs, demonstrating the successful use of the initializer list constructor to initialize a vector in C++.
Initialize a Vector of Structs in C++ Using the Range Constructor
Another effective method for initializing a vector of structs in C++ is by employing the range constructor. This constructor allows us to create a new vector and initialize it with the elements from an existing range, such as another vector.
The syntax for using the range constructor to initialize a vector of structs is as follows:
std::vector<StructType> newVector(existingVector.begin(), existingVector.end());
Here, StructType
represents the type of your struct, and existingVector
is the vector from which you want to copy elements.
Let’s consider the same scenario with the Person
struct. This time, we’ll initialize a new vector (parr3
) using the range constructor and the contents of an existing vector (parr1
).
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Person {
string name;
string surname;
int age;
};
int main() {
// Initialize an existing vector of Person structs
vector<Person> parr1 = {{"John", "Cooper", 32},
{"Theo", "Parrot", 23},
{"Kim", "Colbert", 53},
{"Aun", "Chao", 43}};
// Initialize a new vector using the range constructor
vector<Person> parr3(parr1.begin(), parr1.end());
for (const auto &person : parr3) {
cout << "Name: " << person.name << endl
<< "Surname: " << person.surname << endl
<< "Age: " << person.age << endl;
cout << endl;
}
return EXIT_SUCCESS;
}
In this code snippet, we begin by including the necessary C++ standard library headers and defining the Person
struct, just as in the previous example.
Next, we define a custom struct named Person
, capturing details about individuals such as their name
and surname
as strings and their age
as an integer.
Moving on to the main
function, we initialize an existing vector of Person
structs named parr1
using the initializer list constructor. This constructor allows us to directly populate the vector with instances of the Person
struct, each specified within a set of curly braces.
The new vector parr3
is then initialized using the range constructor. This constructor takes two iterators, parr1.begin()
and parr1.end()
, specifying the range of elements to copy from parr1
to parr3
.
Essentially, it duplicates the content of the existing vector into the new one.
Output:
This output demonstrates the successful use of the range constructor to initialize a new vector (parr3
) with the elements from an existing vector (parr1
) containing Person
structs.
Initialize a Vector of Structs in C++ Using the Custom Constructor
Another effective method for initializing a vector of structs is by employing a custom constructor provided by the vector class. This constructor enables the initialization of a vector with a specified number of identical struct instances.
The syntax for using the custom constructor to initialize a vector of structs is as follows:
std::vector<StructType> newVector(numInstances, defaultValue);
Here, StructType
represents the type of your struct, numInstances
is the desired number of struct instances in the vector, and defaultValue
is an instance of the struct used as a template.
Let’s continue with our Person
struct example. This time, we’ll initialize a vector (parr4
) using the custom constructor, creating three identical instances of the Person
struct.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Person {
string name;
string surname;
int age;
};
constexpr int NUM = 3;
int main() {
// Initialize a vector using the custom constructor
vector<Person> parr4(NUM, {"John", "Cooper", 32});
for (const auto &person : parr4) {
cout << "Name: " << person.name << endl
<< "Surname: " << person.surname << endl
<< "Age: " << person.age << endl;
cout << endl;
}
return EXIT_SUCCESS;
}
In this C++ code, we begin by including the necessary standard library headers for input and output operations (<iostream>
), string manipulation (<string>
), and vector operations (<vector>
). Additionally, we use the using
directive to make our code more concise, bringing cout
, endl
, string
, and vector
into the current scope.
Next, we define a custom struct named Person
. This struct encapsulates information about an individual, with attributes including name
and surname
as strings and age
as an integer.
In the main
function, we set a constant expression NUM
to the value 3
. This will be used as the desired number of instances when initializing the vector.
The vector parr4
is then initialized using the custom constructor. This constructor takes two parameters: the desired number of instances (NUM
) and a default instance of the Person
struct represented by the values {"John", "Cooper", 32}
.
This effectively creates a vector containing three identical instances of the Person
struct.
Output:
This output illustrates the successful use of the custom constructor to initialize a vector (parr4
) with three identical instances of the Person
struct.
Initialize a Vector of Structs in C++ Using push_back
Initializing a vector of structs using the push_back
method provides a dynamic approach, allowing elements to be added to the vector one by one.
This method is particularly useful when the size of the vector is not known in advance or when elements are generated dynamically during program execution. Unlike initializer lists or range constructors, push_back
lets you incrementally add elements to the vector.
The push_back
method is a member function of the std::vector
class. Its syntax is as follows:
myVector.push_back({value1, value2, ...});
Here, myVector
is the vector to which we want to add elements, and the values enclosed in curly braces represent the attributes of the struct.
Consider the Person
struct example. In this code snippet, we initialize an empty vector (peopleVector
) and use push_back
to add individual instances of the Person
struct.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Person {
string name;
string surname;
int age;
};
int main() {
vector<Person> peopleVector;
// Use push_back to add instances to the vector
peopleVector.push_back({"John", "Cooper", 32});
peopleVector.push_back({"Theo", "Parrot", 23});
peopleVector.push_back({"Aun", "Chao", 43});
peopleVector.push_back({"Vivien", "Bardot", 67});
for (const auto &person : peopleVector) {
cout << "Name: " << person.name << endl
<< "Surname: " << person.surname << endl
<< "Age: " << person.age << endl;
cout << endl;
}
return EXIT_SUCCESS;
}
In this code, we start by including the necessary standard library headers and defining the Person
struct. The main
function initializes an empty vector of Person
structs named peopleVector
.
Moving on to the main
function, we initialize an empty vector of Person
structs named peopleVector
. This vector is initially devoid of any elements.
Subsequently, we use the push_back
method to dynamically add instances of the Person
struct to the vector. Each call to push_back
includes a set of values enclosed in curly braces, representing the name
, surname
, and age
attributes of a person.
The push_back
method appends these instances to the end of the vector, allowing us to incrementally build the vector.
Output:
This output demonstrates the successful use of push_back
to dynamically initialize a vector (peopleVector
) with instances of the Person
struct.
Initialize a Vector of Structs in C++ Using emplace_back
The emplace_back
method offers another efficient way to initialize a vector of structs. It allows for the in-place construction of objects directly within the vector, reducing unnecessary copies and providing better performance compared to push_back
.
The emplace_back
method is a member function of the std::vector
class. Its syntax is similar to the constructor of the object being inserted:
myVector.emplace_back(arg1, arg2, ...);
Here, myVector
is the vector to which we want to add elements, and arg1
, arg2
, etc., are the arguments required by the constructor of the struct.
Consider the Person
struct example. In this code snippet, we initialize an empty vector (peopleVector
) and use emplace_back
to construct instances of the Person
struct directly within the vector.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Person {
string name;
string surname;
int age;
Person(const char* n, const char* s, int a) : name(n), surname(s), age(a) {}
};
int main() {
vector<Person> peopleVector;
// Use emplace_back to construct instances in the vector
peopleVector.emplace_back("John", "Cooper", 32);
peopleVector.emplace_back("Theo", "Parrot", 23);
peopleVector.emplace_back("Aun", "Chao", 43);
peopleVector.emplace_back("Vivien", "Bardot", 67);
for (const auto& person : peopleVector) {
cout << "Name: " << person.name << endl
<< "Surname: " << person.surname << endl
<< "Age: " << person.age << endl;
cout << endl;
}
return EXIT_SUCCESS;
}
Beginning with the necessary standard library, including the definition of the Person
struct, the main
function initializes an empty vector of Person
structs named peopleVector
. This vector is created to store instances of the Person
struct.
The primary focus of this example is the utilization of the emplace_back
method. Instead of using push_back
to add instances to the vector, emplace_back
allows us to construct instances of the Person
struct directly within the vector.
Each call to emplace_back
takes arguments corresponding to the parameters of the Person
struct’s constructor, and it constructs instances in place, optimizing performance by avoiding unnecessary copies.
Output:
This output illustrates the successful use of emplace_back
to efficiently initialize a vector (peopleVector
) with instances of the Person
struct. The method’s in-place construction results in improved performance and reduced memory overhead compared to traditional methods like push_back
.
Conclusion
Initializing a vector of structs in C++ can be done in various ways, depending on the version of C++ you are using and your specific requirements.
The initializer list constructor proves effective when struct instances are known at compile time, providing a concise and readable syntax for initialization. On the other hand, the range constructor is ideal when populating a new vector with the content of an existing one, facilitating efficient data transfer.
For scenarios requiring a vector with a fixed number of identical instances, the custom constructor provides a straightforward solution, simplifying the initialization process. Additionally, dynamic situations can be accommodated using push_back
or emplace_back
, with the latter offering enhanced performance by constructing elements in place.
Ultimately, the choice of initialization method depends on the specific requirements of your application, emphasizing readability, efficiency, and flexibility.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook