How to Convert String to Int Array in C++
-
Use
std::getline
andstd::stoi
Functions to Convertstring
toint
Array in C++ -
Use
std::string::find
andstd::stoi
Functions to Convertstring
toint
Array in C++ -
Use
std::copy
andstd::remove_if
Functions to Convertstring
toint
Array in C++
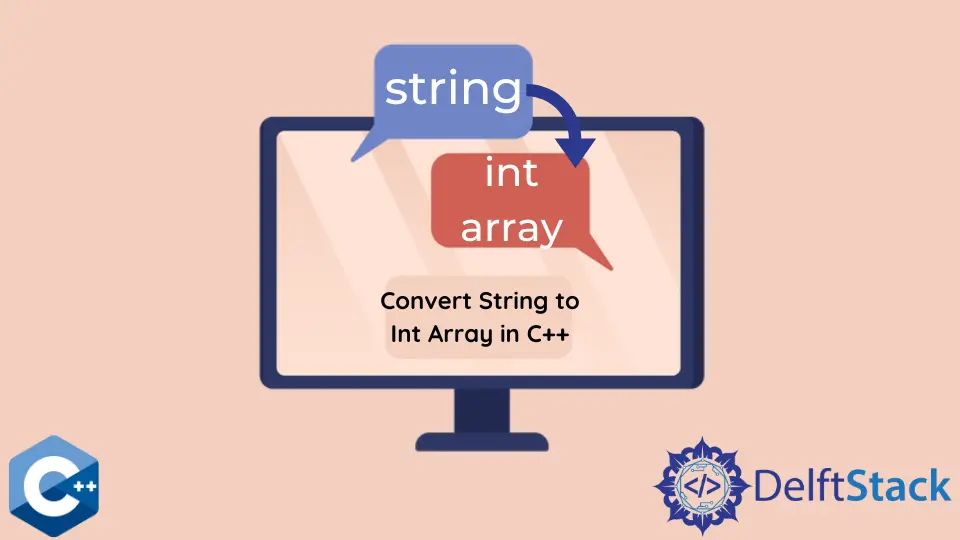
This article will demonstrate multiple methods about how to convert string to int array in C++.
Use std::getline
and std::stoi
Functions to Convert string
to int
Array in C++
std::stoi
is used to convert string values to a signed integer, and it takes one mandatory argument of type std::string
. Optionally, the function can take 2 additional arguments, the first of which can be used to store the index of the last unconverted character. The third argument can optionally specify the number base of the input. Note that we assume the comma-separated numbers as the input string like .csv
file. Thus, we use the getline
function to parse each number and then pass the value to the stoi
. We also store each number in the std::vector
container by calling the push_back
method on every iteration.
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<int> numbers;
int num;
stringstream text_stream(text);
string item;
while (std::getline(text_stream, item, ',')) {
numbers.push_back(stoi(item));
}
for (auto &n : numbers) {
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
Output:
125
44
24
5543
111
Use std::string::find
and std::stoi
Functions to Convert string
to int
Array in C++
Alternatively, we can utilize a find
built-in method of the std::string
class to retrieve the position of the comma delimiter and then call the substr
function to get the number. Then, the substr
result is passed to the stoi
which itself is chained to push_back
method to store the number into the vector
. Note that there’s the line after the while
loop which is necessary to extract the last number in the sequence.
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<int> numbers;
size_t pos = 0;
while ((pos = text.find(',')) != string::npos) {
numbers.push_back(stoi(text.substr(0, pos)));
text.erase(0, pos + 1);
}
numbers.push_back(stoi(text.substr(0, pos)));
for (auto &n : numbers) {
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
Output:
125
44
24
5543
111
Use std::copy
and std::remove_if
Functions to Convert string
to int
Array in C++
Another method to extract integers is to use std::copy
algorithm in conjunction with std::istream_iterator
and std::back_inserter
. This solution stores the string values into a vector
and outputs them to the cout
stream, but one can easily add std::stoi
function to convert each element to int
value. Mind though, that the following example code only stores the numbers as string values.
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<string> nums;
std::istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::back_inserter(nums));
for (auto &n : nums) {
n.erase(std::remove_if(n.begin(), n.end(), ispunct), n.end());
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
Output:
125
44
24
5543
111
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++