How to Erase String in C++
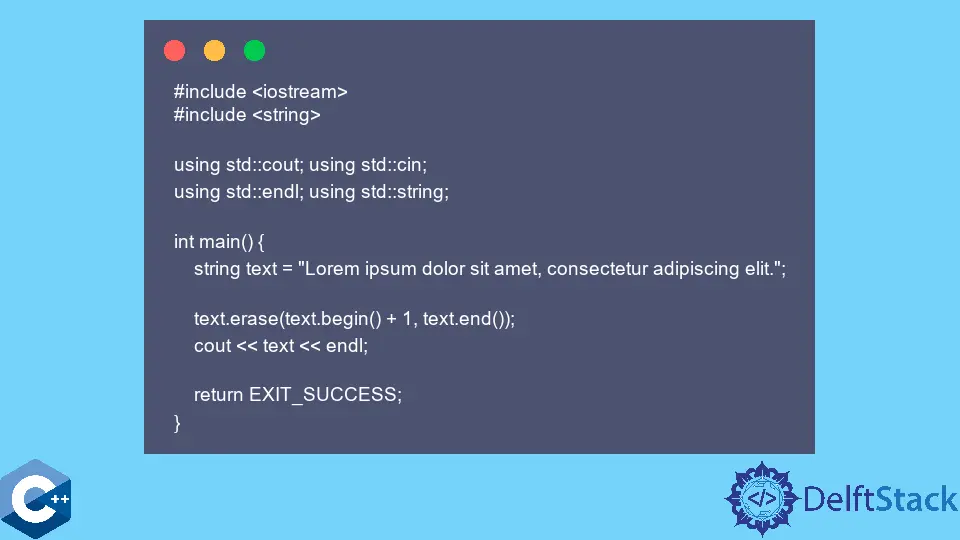
This article will demonstrate how to use the std::string::erase
function to remove a character from a string in C++.
Use std::string::erase
Function to Remove Specified Characters in the String
erase
is a std::string
member function that can be utilized to remove the given characters from the string. It has three overloads, each of which we are going to discuss in the following examples.
The first overload accepts two arguments of size_type
type denoting the index
and the count
. This version will try to erase the count
number of characters starting at the position index
, but in some scenarios, there may be fewer characters left after the given index, so the function is said to remove min(count, size() - index)
characters. The first overload of erase
returns the *this
value.
Both of these parameters have default values, and when we pass a single argument value to the function, the result can be a bit counterintuitive. In fact, the given value is interpreted as the index
parameter, and the count
is assumed to be std::npos
. Consequently, this results in erasing the rest of the string after the given position.
The following code snippet shows similar scenarios - one using find
member functions and the other one - by explicitly passing the index value.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
text.erase(0, 6);
cout << text << endl;
text.erase(text.find('c'));
cout << text << endl;
text.erase(2);
cout << text << endl;
return EXIT_SUCCESS;
}
Output:
ipsum dolor sit amet, consectetur adipiscing elit.
ipsum dolor sit amet,
ip
The second overload of the function accepts a single parameter representing the character’s position to be erased. This argument should be the corresponding iterator type; otherwise, it will invoke the first overload. We can utilize this version of the function together with the std::find
algorithm to remove the first occurrence of the given character from the string. Otherwise, we can pass the character’s relative position using begin
or end
iterators.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
text.erase(std::find(text.begin(), text.end(), ','));
cout << text << endl;
text.erase(text.end() - 5);
cout << text << endl;
return EXIT_SUCCESS;
}
Output:
Lorem ipsum dolor sit amet consectetur adipiscing elit.
Lorem ipsum dolor sit amet consectetur adipiscing lit.
Finally, we have the third overload that takes two iterators as parameters and removes all characters in the corresponding range. The following code sample shows how we can remove all characters in the string except the initial one.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
text.erase(text.begin() + 1, text.end());
cout << text << endl;
return EXIT_SUCCESS;
}
Output:
L
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++