How to Sort Vector in C++
-
Use the
std::sort
Algorithm to Sort Vector Elements -
Use the
std::sort
Function With Lambda Expression to Sort Vector ofstruct
-
Use the
std::sort
Function With Custom Function to Sort Vector ofstruct
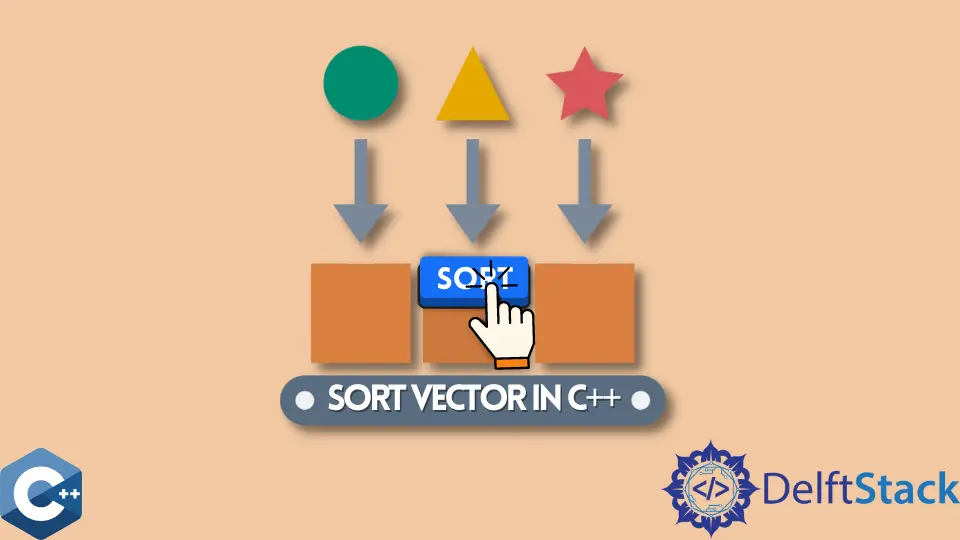
This article will demonstrate multiple methods of how to sort a vector in C++.
Use the std::sort
Algorithm to Sort Vector Elements
The std::sort
function implements a generic algorithm to work with different objects and sorts the given elements in the range using the comparator function passed as the third argument. Note that the function can be used without the third argument, in which case the elements get sorted using operator<
. The following example code demonstrates such a scenario, where the element is of type string, which has operator<
member function and can be sorted with default comparator.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<string> vec1 = {"highway", "song", "world", "death",
"mom", "historian", "menu", "woman"};
printVector(vec1);
sort(vec1.begin(), vec1.end());
printVector(vec1);
return EXIT_SUCCESS;
}
Output:
highway, song, world, death, mom, historian, menu, woman,
death, highway, historian, menu, mom, song, woman, world,
Use the std::sort
Function With Lambda Expression to Sort Vector of struct
Alternatively, a custom comparator function object can be constructed with a lambda expression to sort the user-defined structures. In this case, we have a struct cpu
with different data members, and two sort
calls are constructed by passing the function objects that compare value
or property1
members, respectively.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
struct cpu {
string property1;
string property2;
string property3;
int value;
} typedef cpu;
void printVector(vector<cpu> &vec) {
for (const auto &item : vec) {
cout << item.property1 << " : " << item.property2 << " : " << item.property3
<< " : " << item.value << endl;
}
cout << endl;
}
int main() {
vector<cpu> vec3 = {{"WMP", "GR", "33", 2023},
{"TPS", "US", "31", 2020},
{"EOM", "GB", "36", 2021},
{"AAW", "GE", "39", 2024}};
printVector(vec3);
sort(vec3.begin(), vec3.end(),
[](cpu &x, cpu &y) { return x.value < y.value; });
sort(vec3.begin(), vec3.end(),
[](cpu &x, cpu &y) { return x.property1 < y.property1; });
printVector(vec3);
return EXIT_SUCCESS;
}
Use the std::sort
Function With Custom Function to Sort Vector of struct
Notice that the previous method is quite inflexible to be utilized in larger codebases and can make code rather bulky if the comparison function is complicated. Another solution would be to implement comparison functions as struct
members and declare them as static
to be accessible through the scope operator. Also, these functions must return a bool
value and only have two parameters.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
struct cpu {
string property1;
string property2;
string property3;
int value;
public:
static bool compareCpusByValue(cpu &a, cpu &b) { return a.value < b.value; }
static bool compareCpusByProperty1(cpu &a, cpu &b) {
return a.property1 < b.property1;
}
} typedef cpu;
void printVector(vector<cpu> &vec) {
for (const auto &item : vec) {
cout << item.property1 << " : " << item.property2 << " : " << item.property3
<< " : " << item.value << endl;
}
cout << endl;
}
int main() {
vector<cpu> vec3 = {{"WMP", "GR", "33", 2023},
{"TPS", "US", "31", 2020},
{"EOM", "GB", "36", 2021},
{"AAW", "GE", "39", 2024}};
printVector(vec3);
sort(vec3.begin(), vec3.end(), cpu::compareCpusByProperty1);
sort(vec3.begin(), vec3.end(), cpu::compareCpusByValue);
printVector(vec3);
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook