How to Use Smart Pointers in C++
-
Use
std::shared_ptr
for Multiple Pointers to Refer to the Same Object in C++ -
Use
std::unique_ptr
for a Pointer to Own the Given Object in C++
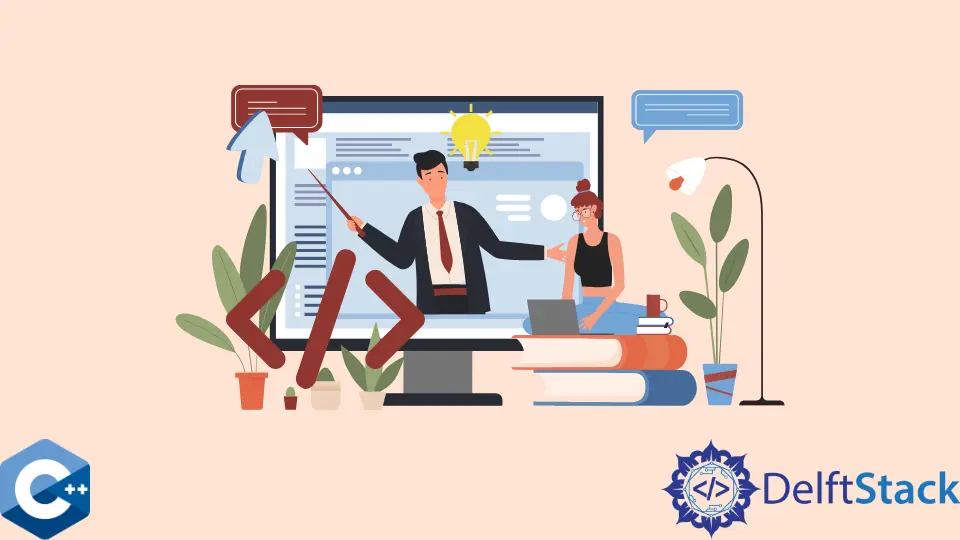
This article will demonstrate multiple methods of how to use smart pointers in C++.
Use std::shared_ptr
for Multiple Pointers to Refer to the Same Object in C++
Since the manual management of dynamic memory happens to be quite hard for real-world projects and often is the main cause of the bugs, C++ provides the concept of smart pointers as a separate library. Smart pointers generally act as regular pointers and provide extra features, the most prominent of which is automatically freeing the pointed objects. There are two core types of smart pointers: shared_ptr
and unique_ptr
.
The following example code demonstrates the usage of the shared_ptr
type. Note that shared_ptr
is usually used when the pointed object should be referenced by other shared pointers. Thus, shared_ptr
internally has the associated counter, which denotes the number of pointers that point to the same object. shared_ptr::unique
member function can be used to determine if the pointer is only one referencing the current object. The function return type is boolean, and the true
value confirms the object’s exclusive ownership. reset
built-in function replaces the current object with the object passed as the first parameter. The shared object is deleted when the last shared_ptr
pointing to the object goes out of scope.
#include <iostream>
#include <memory>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
std::shared_ptr<string> p(new string("Arbitrary string"));
std::shared_ptr<string> p2(p);
cout << "p : " << p << endl;
cout << "p2: " << p2 << endl;
if (!p.unique()) {
p.reset(new string(*p));
}
cout << "p : " << p << endl;
*p += " modified";
cout << "*p : " << *p << endl;
cout << "*p2 : " << *p2 << endl;
return EXIT_SUCCESS;
}
Output:
p : 0x2272c20
p2: 0x2272c20
p : 0x2273ca0
*p : Arbitrary string modified
*p2 : Arbitrary string
Use std::unique_ptr
for a Pointer to Own the Given Object in C++
Alternatively, C++ provides the unique_ptr
type that is the sole owner of the object. No other unique_ptr
pointer can reference it. unique_ptr
does not support ordinary copy and assignment operations. Still, the object ownership can be transferred between two unique_ptr
pointers using the built-in functions: release
and reset
. The release
function call makes the unique_ptr
pointer null. Once the unique_ptr
is released, then the reset
function can be called to assign new object ownership to it.
#include <iostream>
#include <memory>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
std::unique_ptr<string> p1(new string("Arbitrary string 2"));
std::unique_ptr<string> p3(p1.release());
cout << "*p3 : " << *p3 << endl;
cout << "p3 : " << p3 << endl;
cout << "p1 : " << p1 << endl;
std::unique_ptr<string> p4(new string("Arbitrary string 3"));
p3.reset(p4.release());
cout << "*p3 : " << *p3 << endl;
cout << "p3 : " << p3 << endl;
cout << "p4 : " << p4 << endl;
return EXIT_SUCCESS;
}
Output:
*p3 : Arbitrary string 2
p3 : 0x7d4c20
p1 : 0
*p3 : Arbitrary string 3
p3 : 0x7d5c80
p4 : 0
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook