Function Pointer to Member Function in C++
- Implement Function Pointer to Member Function in C++
- Affix a Pointer to a Member Function in C++
- Call a Pointer to Member Function Without Using the Pointer Symbol in C++
- Give a Method From the Same Class As Function Pointer in C++
- Function Pointer to Member Function Using String Command in C++
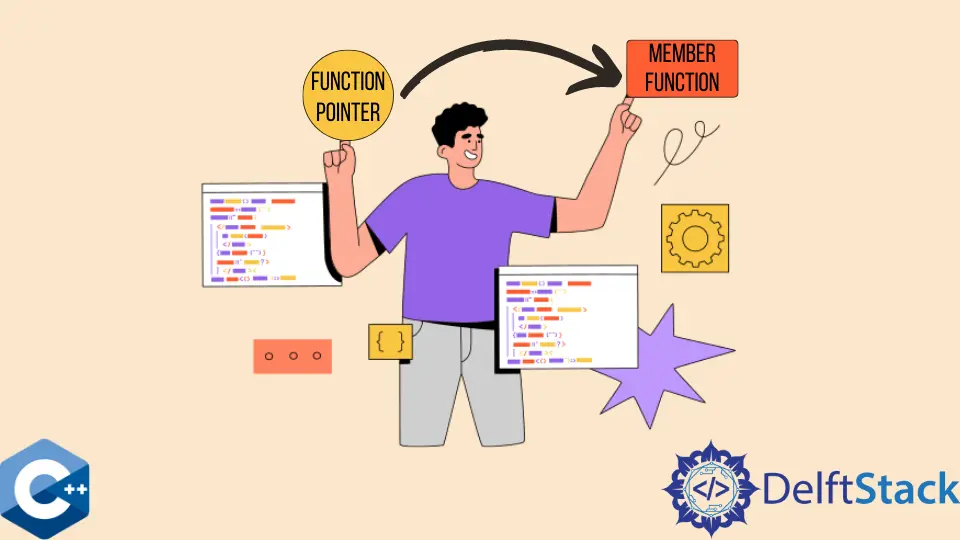
When a variable is created in programming languages, the machine must store its value in computer memory. The machine allots some memory to this variable, whether empty or not.
The location is often required instead of a variable’s values. During that time, these values can be fetched through pointers.
Additionally, pointers in C++ can also be used to point to a piece of code, such as a function, compared to referencing a memory address. These are called Function pointers, increasing the efficiency of code.
This article’s examples will clearly show how function pointers can be employed inside a class to make pointers point to a member function in C++.
Implement Function Pointer to Member Function in C++
There are two ways through which this can be done.
- A function pointer is created that points to a member function.
- Calling a method using just the name of the function pointer.
Affix a Pointer to a Member Function in C++
The following steps are required to use a pointer function.
- A parameterized function with a return type - void.
- Type Casting the pointer variable.
- Allocating the pointer variable to a method.
- A method call that uses the pointer to call the parameterized function inside the main function.
The standard stdio
package is imported for input/output functions inside the program. A function display
is declared with a parameter var1
, with an integer datatype.
void display(int var1) { printf("The variable holds value = %d\n", var1); }
Inside the display
method, var1
is printed.
The variable ptr_method
has a datatype void inside the main function. It must be noted that void can be used as a datatype in c++, but it acts as a placeholder and does not genuinely represent a type of data.
The declared pointer variable is then provided with an integer parameter as:
void (*ptr_method)(int)
The ptr_method
has an integer parameter. This will pass a value to the member method while calling ptr_method
.
Because using any other datatype results in an incorrect conversion error with the compiler, the pointer function ptr_method
has been given a void data type.
The function pointer gets assigned to the function declared at the program’s start using the syntax below. Use the following syntax to make the pointer point towards the function display
.
ptr_method = &display;
Finally, the pointer function is called with the integer parameter provided as "65"
.
Code:
#include <stdio.h>
// This method has a parameter int and a return type which is void
void display(int var1) { printf("The variable holds value = %d\n", var1); }
int main() {
void (*ptr_method)(int);
ptr_method = &display;
(*ptr_method)(65);
return 0;
}
Output:
The variable holds value = 65
Call a Pointer to Member Function Without Using the Pointer Symbol in C++
The program used in this example is the same as the above one except for the function call. Here, the pointer variable is called using the variable’s name, excluding the asterisk sign(*
).
The program works as intended because when a function pointer is pointed towards a method, the memory address of the method stored inside it can be accessed using both the pointer and the variable form. For instance, when the function pointer ptr_method
is defined, the function display
assignment is accomplished by utilizing the variable name directly rather than adding a &
in front of the variable name as in the previous example.
At the function call, the function pointer ptr_method
is called directly without the pointer symbol (*
) and passed the value 54
.
void (*ptr_method)(int);
ptr_method = display;
ptr_method(54);
Code:
#include <stdio.h>
void display(int var1) { printf("The variable holds value = %d\n", var1); }
int main() {
void (*ptr_method)(int);
ptr_method = display;
ptr_method(54);
return 0;
}
Output:
The variable holds value = 54
Give a Method From the Same Class As Function Pointer in C++
The program used in this example is similar to the other two programs. But here, the pointer is a method, whereas the work was handled by simple pointer variables in the other two programs.
A constructor class assgnPtr
is created with two public members - a char method foo and another char method which is a function pointer ptr
. The two methods are shown in the syntax below, and it is clear from the last line of the syntax that it points to the class assgnPtr
for the function pointer.
public:
char foo();
char (assgnPtr::*ptr)();
After the public methods are declared, function foo()
is provided a return f
. This implies that when the function pointer is called, the program calls the function foo
and prints whatever is returned.
A variable named var1
is declared inside the main method. This variable functions as a class object.
It is because function pointers which are member functions have the drawback of being inaccessible when used without an object from the same class. The member function is assigned to the pointer variable in the following syntax.
int main() {
assgnPtr var1;
var1.ptr = &assgnPtr::foo;
The pointer ptr
is used along with the object class var1
, and it is assigned method foo
. As a constructor, the method has to be assigned using the ::
symbol, indicating that the function is the member method of the class assgnPrt
.
Lastly, the result needs to be printed.
printf("%c\n", (var1.*(var1.ptr))());
It can be troubling to readers as it is very complicated to understand. So it needs to be broken down into bits to understand clearly.
var1.ptr
is a pointer to the member function of class assgnPtr
. But when *(var1.ptr
) is used, it turns into a pointer reference while printing *(var1.ptr
) cannot be used directly as ptr
is not in scope.
For that reason, it needs to be bound with the class object var1
as var1.*(var1.ptr)
.
Lastly, (var1.*(var1.ptr))()
invokes the method with no arguments. The print statement prints the value returned when the method is called using the function pointer.
Full Source Code:
#include <iostream>
class assgnPtr {
public:
char foo();
char (assgnPtr::*ptr)();
};
char assgnPtr::foo() { return 'f'; }
int main() {
assgnPtr var1;
var1.ptr = &assgnPtr::foo;
printf("%c\n", (var1.*(var1.ptr))());
}
Output:
f
Function Pointer to Member Function Using String Command in C++
In this example, the program uses string commands to assign function pointers. The core concept is similar, except the member method uses a relational operator to check and assign the pointer.
The program uses two import functions, iostream
for i/o
and string
for string commands. A constructor class assgnPtr2
is created.
There are two private members, display
and pass_value
and a public member, func_ptr
, which will be the function pointer.
The private member pass_value
has three parameters - variables x and y of datatype string, and a function prototype foo
. The function prototype states that the parameter foo
will be a pointer to a function.
Method display
will print a statement when the pointer is assigned. Method func_ptr
calls method pass_value
and passes the values of x
& y
as a parameter, and attaches method display
using the &
symbol as an argument for the function prototype.
Lastly, inside method pass_value
, the values of x and y which were passed are compared using the relational operator ==
. An if statement assigns the pointer foo
to the method if both the values of x and y are matched.
Inside the main function, a class object var
is created. Using this object, the method func_ptr
is called.
Full Source Code:
#include <iostream>
#include <string>
class assgnPtr2 {
public:
void func_ptr();
private:
void display();
void pass_value(std::string x, std::string y, void (assgnPtr2::*foo)());
};
void assgnPtr2::display() { std::cout << "Pointer Assigned\n"; }
void assgnPtr2::func_ptr() { pass_value("h", "h", &assgnPtr2::display); }
void assgnPtr2::pass_value(std::string x, std::string y,
void (assgnPtr2::*foo)()) {
if (x == y) {
(this->*foo)();
}
}
int main() {
assgnPtr2 var;
var.func_ptr();
return 0;
}
Output:
Pointer Assigned