How to Swap Two Numbers Using Pointers in C++
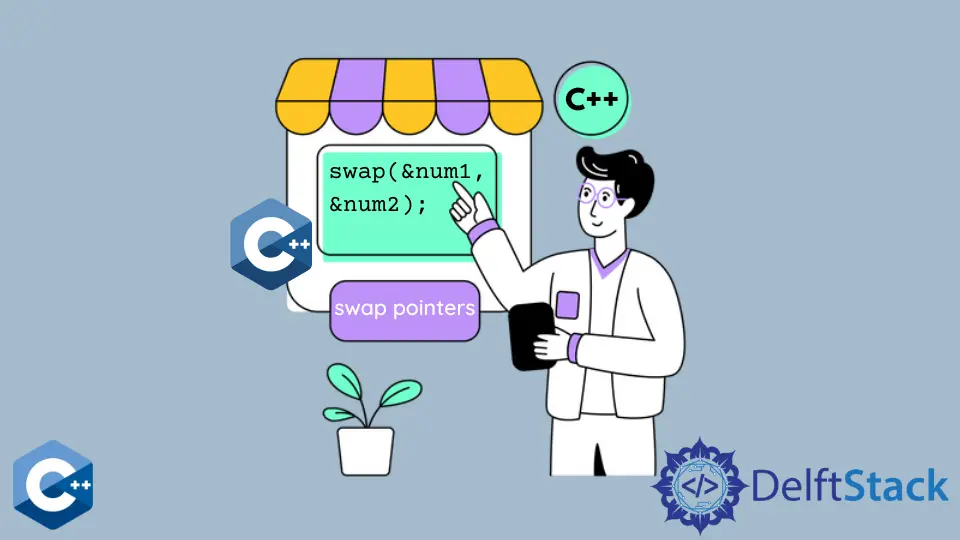
Swapping two numbers is a common operation in programming, and there are various ways to achieve it. One interesting approach is to use pointers in C++.
Pointers provide a way to manipulate the memory directly, allowing us to swap values efficiently without the need for a temporary variable. In this article, we will explore how to swap two numbers using pointers in C++.
Pointers in C++
Before diving into swapping numbers, let’s have a quick refresher on pointers. A pointer is a variable that holds the memory address of another variable.
This ability to reference memory locations directly enables more efficient manipulation of data, making pointers a crucial aspect of low-level programming.
In C++, you declare a pointer using the asterisk (*
) symbol. Let’s consider an example where we have an integer variable and a pointer to that integer:
int number = 42;
int *ptr = &number;
In this example, ptr
is a pointer to an integer, and &number
represents the address of the number
variable. The *ptr
notation is used to access the value stored at the memory location pointed to by ptr
.
std::cout << "Value of number: " << number << "\n";
std::cout << "Address of number: " << &number << "\n";
std::cout << "Value using pointer: " << *ptr << "\n";
std::cout << "Address stored in pointer: " << ptr << "\n";
This code snippet demonstrates how to output the value and address of the number
variable directly and through the pointer ptr
.
Another important aspect is pointer arithmetic, which allows you to manipulate pointers by adding or subtracting integers. This can be particularly useful when working with arrays or traversing memory blocks.
For example:
int numbers[] = {1, 2, 3, 4, 5};
int *ptr = numbers; // Pointer points to the first element of the array
// Accessing array elements using pointer arithmetic
std::cout << "Element 1: " << *ptr << "\n";
std::cout << "Element 2: " << *(ptr + 1) << "\n";
std::cout << "Element 3: " << *(ptr + 2) << "\n";
In this snippet, ptr
is initially set to the address of the first element of the numbers
array. Using pointer arithmetic, we can access different elements of the array by incrementing the pointer.
Understanding pointers is crucial for more advanced programming tasks, and it lays the foundation for various operations, including dynamic memory allocation, function pointers, and the topic at hand—swapping variables efficiently using pointers.
In the next section, we will delve into the process of swapping two numbers using pointers in C++.
Swap Numbers Using Pointers in C++
Utilizing pointers in C++ provides an efficient way to swap two numbers without the need for a temporary variable. Swapping with pointers involves manipulating the values indirectly by exchanging the data stored at their memory addresses.
Let’s explore the steps involved in swapping two numbers using pointers.
-
Begin by declaring two integer variables that you want to swap:
int a = 5; int b = 10;
Here, `a` and `b` are the two numbers you wish to interchange.
-
Next, declare two pointer variables and assign them the addresses of the variables to be swapped:
int *ptrA = &a; int *ptrB = &b;
Now, `ptrA` holds the address of `a`, and `ptrB` holds the address of `b`.
-
Utilize pointer dereferencing to access and swap the values stored at the memory locations:
// Swapping values using pointers int temp = *ptrA; *ptrA = *ptrB; *ptrB = temp;
In these lines, a temporary variable `temp` is employed to store the value pointed to by `ptrA`. Subsequently, the value pointed to by `ptrA` is updated with the value pointed to by `ptrB`, and vice versa.
Combining all the steps, the complete code for swapping two numbers using pointers looks like this:
#include <iostream>
int main() {
int a = 5;
int b = 10;
std::cout << "Before swapping:\n";
std::cout << "a = " << a << "\n";
std::cout << "b = " << b << "\n";
int *ptrA = &a;
int *ptrB = &b;
int temp = *ptrA;
*ptrA = *ptrB;
*ptrB = temp;
std::cout << "After swapping:\n";
std::cout << "a = " << a << "\n";
std::cout << "b = " << b << "\n";
return 0;
}
Output:
Before swapping:
a = 5
b = 10
After swapping:
a = 10
b = 5
Let’s enhance the concept by encapsulating the swapping logic into a function. This promotes code reusability and makes the program more modular.
-
First, we declare a function called
swap
that takes two integer pointers as parameters and swaps the values they point to:void swap(int *num1, int *num2) { int temp = *num1; *num1 = *num2; *num2 = temp; }
The function uses a temporary variable, `temp`, to facilitate the swapping without the need for an additional variable.
-
In the
main
function, we declare two integer variables,num1
andnum2
. User input is then obtained for these numbers:int num1, num2; std::cout << "\nEnter the first number : "; std::cin >> num1; std::cout << "\nEnter the Second number : "; std::cin >> num2;
-
We call the
swap
function, passing the addresses ofnum1
andnum2
as arguments:swap(&num1, &num2);
After the swap, the program displays the swapped values:
```c++
std::cout << "\nAfter Swapping, we have observed the following changes";
std::cout << "\nFirst number : " << num1;
std::cout << "\nSecond number: " << num2;
```
Here’s the complete code example:
#include <iostream>
using namespace std;
void swap(int *num1, int *num2) {
int temp;
temp = *num1;
*num1 = *num2;
*num2 = temp;
}
int main() {
int num1, num2;
cout << "Enter the first number : ";
cin >> num1;
cout << "\nEnter the Second number : ";
cin >> num2;
swap(&num1, &num2);
cout << "\nAfter Swapping, we have observed the following changes";
cout << "\nFirst number : " << num1;
cout << "\nSecond number: " << num2;
}
Output:
Enter the first number : 34
Enter the Second number : 43
After Swapping, we have observed the following changes
First number : 43
Second number: 34
Click here to see the code’s live demo.
Conclusion
Understanding pointers in C++ is essential for efficient memory management and manipulation. The examples provided demonstrate the process of swapping numbers using pointers, showcasing the elegance and power of pointers in direct memory manipulation.
As you continue to learn pointers, you’ll find their applications extend beyond simple swapping operations, contributing to more advanced programming tasks. By incorporating pointers into your programming toolkit, you enhance your ability to write concise, performant, and modular code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook