Dangling Pointers in C++
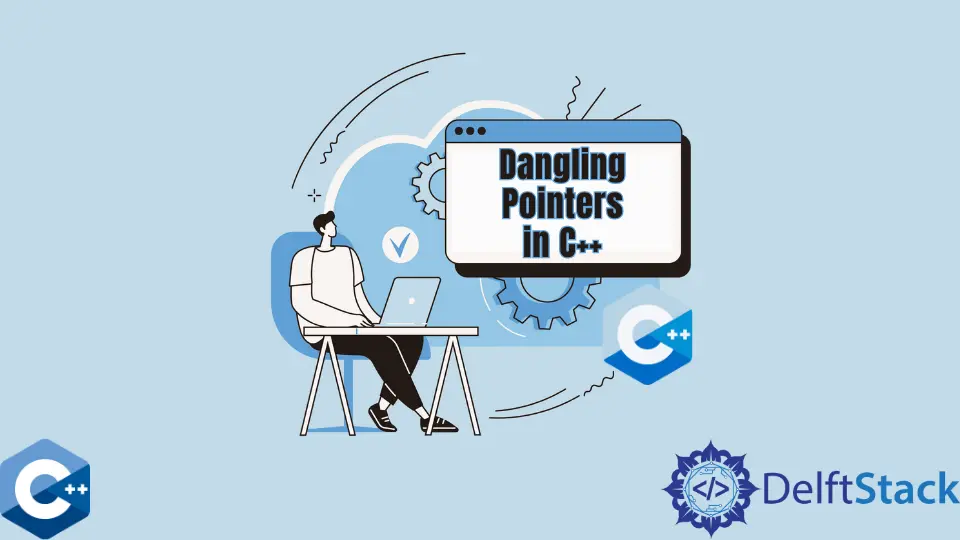
Understanding dangling pointers is crucial for any C++ developer. A dangling pointer is a pointer that references a memory location of an object that has already been deleted or deallocated. This situation can lead to unpredictable behavior, memory leaks, or program crashes. When you delete an object in C++, the pointer still holds the address of that memory location, which can lead to serious issues if you try to access it.
In this article, we will delve deeper into what dangling pointers are, how they occur, and the best practices to avoid them. By the end, you will have a solid grasp of this important concept in C++ programming.
What Causes Dangling Pointers?
Dangling pointers usually arise from the following situations:
- Memory Deallocation: When you delete an object using the
delete
operator, the pointer that pointed to that object becomes a dangling pointer. - Scope Exit: Local variables go out of scope when the function exits. If a pointer points to a local variable, it becomes dangling once the function returns.
- Pointer Reassignment: If a pointer is assigned to another pointer that has been deleted, the original pointer becomes dangling.
Understanding these causes helps in preventing dangling pointers in your code.
How to Avoid Dangling Pointers
1. Set Pointers to Null After Deletion
One of the simplest techniques to avoid dangling pointers is to set pointers to nullptr
after deleting the objects they point to. This way, even if you try to access the pointer later, it won’t point to a garbage value.
#include <iostream>
int main() {
int* ptr = new int(42);
std::cout << "Value before deletion: " << *ptr << std::endl;
delete ptr;
ptr = nullptr; // Set pointer to nullptr to avoid dangling pointer
if (ptr) {
std::cout << "Value after deletion: " << *ptr << std::endl;
} else {
std::cout << "Pointer is now null." << std::endl;
}
return 0;
}
Output:
Value before deletion: 42
Pointer is now null.
Setting the pointer to nullptr
ensures that it no longer points to the deleted memory. This can help prevent accidental dereferencing of a dangling pointer, which could lead to undefined behavior in your program.
2. Use Smart Pointers
Modern C++ provides smart pointers like std::unique_ptr
and std::shared_ptr
. These automatically manage memory and help prevent dangling pointers. When the smart pointer goes out of scope, it automatically deletes the memory it points to.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr(new int(42));
std::cout << "Value before deletion: " << *ptr << std::endl;
ptr.reset(); // Automatically deletes the memory
if (!ptr) {
std::cout << "Pointer is now null." << std::endl;
}
return 0;
}
Output:
Value before deletion: 42
Pointer is now null.
By using std::unique_ptr
, you can ensure that the memory is properly managed, and you won’t have to worry about manually deleting it. This significantly reduces the risk of creating dangling pointers in your code.
3. Avoid Returning Pointers to Local Variables
Returning a pointer to a local variable can also lead to dangling pointers. Once the function exits, the local variable is destroyed, and the pointer becomes invalid. Instead, consider returning a pointer to dynamically allocated memory or using smart pointers.
#include <iostream>
int* createValue() {
int* ptr = new int(42);
return ptr; // Returning pointer to dynamically allocated memory
}
int main() {
int* ptr = createValue();
std::cout << "Value: " << *ptr << std::endl;
delete ptr; // Don't forget to delete the memory
return 0;
}
Output:
Value: 42
In this example, the pointer returned from createValue
points to dynamically allocated memory, which remains valid even after the function exits. However, remember to delete the memory to avoid memory leaks.
Conclusion
Dangling pointers are a common pitfall in C++ programming that can lead to serious issues such as crashes and undefined behavior. By understanding their causes and employing strategies like setting pointers to nullptr
, using smart pointers, and avoiding returning pointers to local variables, you can significantly reduce the risk of encountering dangling pointers in your applications. Always remember that proper memory management is key to writing robust and reliable C++ code.
FAQ
-
What is a dangling pointer?
A dangling pointer is a pointer that references a memory location that has been deleted or deallocated. -
How can I avoid dangling pointers in C++?
You can avoid dangling pointers by setting pointers to nullptr after deletion, using smart pointers, and avoiding returning pointers to local variables. -
What are smart pointers?
Smart pointers are objects that manage memory automatically, preventing memory leaks and dangling pointers. Examples include std::unique_ptr and std::shared_ptr.
-
What happens if I dereference a dangling pointer?
Dereferencing a dangling pointer can lead to undefined behavior, crashes, or memory corruption. -
Can dangling pointers cause memory leaks?
Yes, dangling pointers can contribute to memory leaks if the memory they point to is not properly managed or deleted.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook