Void Pointer in C++
- What is a Void Pointer?
- How to Use Void Pointers in C++
- Advantages of Using Void Pointers
- Common Mistakes with Void Pointers
- Conclusion
- FAQ
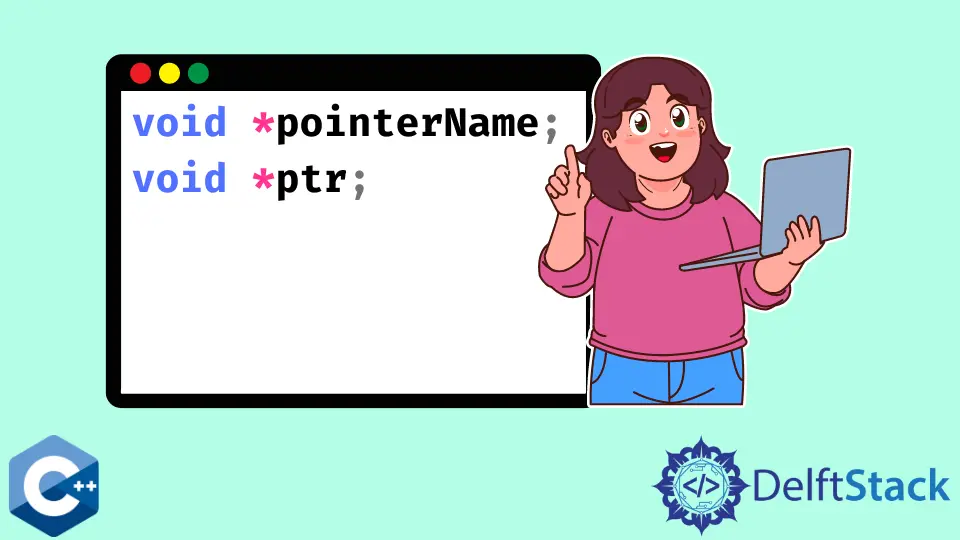
In the realm of C and C++, pointers are an essential concept that every programmer must grasp. Among these pointers, the void pointer stands out as a versatile tool. Unlike regular pointers that are tied to specific data types, a void pointer can point to any data type, making it a general-purpose pointer. This flexibility allows developers to write more generic and reusable code. Functions such as malloc()
and calloc()
return void pointers, which can then be typecast to the desired data type.
In this article, we will explore the concept of void pointers in C++, how they work, and why they are significant in programming.
What is a Void Pointer?
A void pointer is essentially a pointer that does not have a specific data type associated with it. This means that it can point to any data type, whether it’s an integer, a character, or even a user-defined structure. The syntax for declaring a void pointer is simple:
void* ptr;
Here, ptr
is a void pointer. The beauty of a void pointer lies in its ability to be typecast to any other pointer type as needed. For instance, if you have a void pointer and you know it points to an integer, you can cast it like this:
int* intPtr = (int*)ptr;
This typecasting is crucial when working with functions that return void pointers, as it allows you to manipulate the data correctly.
How to Use Void Pointers in C++
Using void pointers effectively requires understanding how to manipulate and typecast them. Let’s look at an example that demonstrates the use of a void pointer in a function that allocates memory dynamically.
#include <iostream>
#include <cstdlib>
void* allocateMemory(size_t size) {
return malloc(size);
}
int main() {
int* arr = (int*)allocateMemory(5 * sizeof(int));
for (int i = 0; i < 5; i++) {
arr[i] = i + 1;
}
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
free(arr);
return 0;
}
In this example, we define a function allocateMemory
that takes a size as an argument and returns a void pointer. Inside the main
function, we call this function to allocate memory for an array of integers. We then cast the void pointer returned by allocateMemory
to an integer pointer. This allows us to manipulate the allocated memory as an integer array. Finally, we print the values stored in the array and free the allocated memory.
Output:
1 2 3 4 5
This code snippet illustrates the power of void pointers in managing dynamic memory allocation. By using a void pointer, we can create a function that is not limited to a specific data type, enhancing code reusability.
Advantages of Using Void Pointers
Void pointers offer several advantages that make them a valuable asset in C and C++ programming:
-
Type Agnosticism: Since void pointers are not tied to a specific data type, they can be used to point to any type of data. This flexibility is especially useful in generic programming and when writing functions that can operate on different data types.
-
Memory Management: Functions like
malloc()
andcalloc()
return void pointers, which are crucial for dynamic memory management. This allows developers to allocate memory at runtime, adapting to the program’s needs. -
Functionality: Void pointers can be passed to functions that require pointers without needing to specify the data type. This can simplify function signatures and enhance code readability.
However, it is essential to remember that while void pointers provide flexibility, they require careful handling. Since they do not have type information, improper typecasting can lead to undefined behavior or runtime errors.
Common Mistakes with Void Pointers
While void pointers are powerful, they can also lead to common pitfalls if not used correctly. Here are some mistakes to avoid:
-
Improper Typecasting: One of the most frequent errors is failing to cast a void pointer to the correct type before dereferencing it. This can lead to incorrect memory access and potential crashes.
-
Memory Leaks: When using dynamic memory allocation with void pointers, it is crucial to free the allocated memory. Failing to do so can result in memory leaks, which can degrade performance over time.
-
Pointer Arithmetic: Performing pointer arithmetic on void pointers is not allowed because the compiler does not know the size of the data type being pointed to. Always ensure you cast the void pointer to the correct type before performing arithmetic operations.
By being aware of these common mistakes, developers can use void pointers more effectively and avoid potential issues in their programs.
Conclusion
Void pointers are a fundamental concept in C and C++ that provide flexibility and power in managing data types and memory allocation. By understanding how to use and manipulate void pointers, developers can write more generic and reusable code. While they offer significant advantages, it is essential to handle them with care to avoid common pitfalls. As you continue your journey in programming, mastering void pointers will undoubtedly enhance your coding skills and open up new possibilities in your projects.
FAQ
-
What is a void pointer in C++?
A void pointer is a pointer that does not have a specific data type associated with it, allowing it to point to any data type. -
How do you typecast a void pointer?
You can typecast a void pointer by using the desired data type in parentheses before the pointer, like this:int* intPtr = (int*)voidPtr;
. -
Why are void pointers useful?
Void pointers are useful because they allow for generic programming and dynamic memory management, enabling functions to operate on different data types. -
Can you perform pointer arithmetic on void pointers?
No, pointer arithmetic cannot be performed on void pointers because the compiler does not know the size of the data type being pointed to. -
How do you free memory allocated with a void pointer?
You can free memory allocated with a void pointer by passing it to thefree()
function, just like any other pointer.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn