How to Shuffle Vector in C++
-
Use the
shuffle
Algorithm to Shuffle Vector Elements -
Use the
random_shuffle
Algorithm to Shuffle Vector Elements
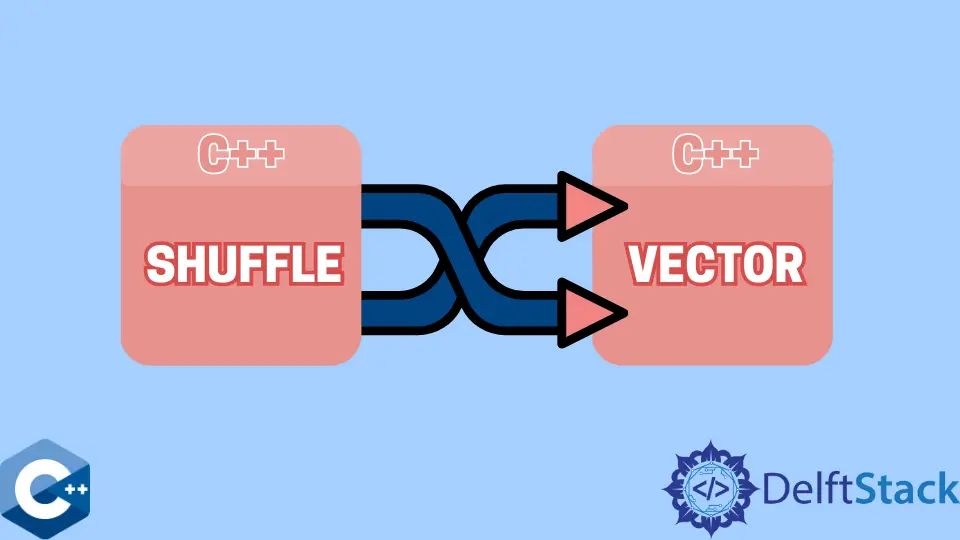
This article will demonstrate multiple methods about how to shuffle vector elements in C++.
Use the shuffle
Algorithm to Shuffle Vector Elements
std::shuffle
is part of the C++ <algorithm>
library and implements the random permutation feature, which can be applied to the elements of the given range. The function takes range iterators as the first two arguments and the random number generator as the third one. A random number generator is a function object. Contemporary C++ recommends using the standard library utilities of random number generation. std::random_device
should be utilized for non-deterministic number generation.
Finally, the chosen random number engine object must be created and passed to the shuffle
algorithm to generate a random permutation of the range. Note that we print a vector of integers before and after the shuffling has been done.
#include <algorithm>
#include <iostream>
#include <random>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::shuffle;
using std::string;
using std::vector;
template <typename T>
void printVectorElements(vector<T> &vec) {
for (auto i = 0; i < vec.size(); ++i) {
cout << vec.at(i) << "; ";
}
cout << endl;
}
int main() {
vector<int> i_vec1 = {12, 32, 43, 53, 23, 65, 84};
cout << "i_vec1 : ";
printVectorElements(i_vec1);
std::random_device rd;
std::default_random_engine rng(rd());
shuffle(i_vec1.begin(), i_vec1.end(), rng);
cout << "i_vec1 (shuffled): ";
printVectorElements(i_vec1);
cout << endl;
return EXIT_SUCCESS;
}
Output:
i_vec1 : 12; 32; 43; 53; 23; 65; 84;
i_vec1 (shuffled): 53; 32; 84; 23; 12; 43; 65;
As an alternative to the previous method, one can implement the same subroutine using std::begin
and std::end
objects to pass the range iterators to the shuffle
function. The following example could be a more generic version for specific coding scenarios.
#include <algorithm>
#include <iostream>
#include <random>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::shuffle;
using std::string;
using std::vector;
template <typename T>
void printVectorElements(vector<T> &vec) {
for (auto i = 0; i < vec.size(); ++i) {
cout << vec.at(i) << "; ";
}
cout << endl;
}
int main() {
vector<int> i_vec1 = {12, 32, 43, 53, 23, 65, 84};
cout << "i_vec1 : ";
printVectorElements(i_vec1);
std::random_device rd;
std::default_random_engine rng(rd());
shuffle(std::begin(i_vec1), std::end(i_vec1), rng);
cout << "i_vec1 (shuffled): ";
printVectorElements(i_vec1);
cout << endl;
return EXIT_SUCCESS;
}
Output:
i_vec1 : 12; 32; 43; 53; 23; 65; 84;
i_vec1 (shuffled): 43; 23; 32; 65; 53; 12; 84;
Use the random_shuffle
Algorithm to Shuffle Vector Elements
std::random_shuffle
is another utility algorithm from the C++ standard library. The older version of the std::shuffle
has been depreciated for the newest C++ standards. Although it can be utilized in more legacy coding environments where older C++ versions are available.
random_shuffle
can take a user-provided random number generator, but since the older versions of C++ lacked the random library facilities, one may provide only range iterators to the function. In the latter case, random_shuffle
utilizes an implementation-defined random number generator, which sometimes happens to be the std::rand
function call.
#include <algorithm>
#include <iostream>
#include <random>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::shuffle;
using std::string;
using std::vector;
template <typename T>
void printVectorElements(vector<T> &vec) {
for (auto i = 0; i < vec.size(); ++i) {
cout << vec.at(i) << "; ";
}
cout << endl;
}
int main() {
vector<int> i_vec1 = {12, 32, 43, 53, 23, 65, 84};
cout << "i_vec1 : ";
printVectorElements(i_vec1);
std::random_shuffle(i_vec1.begin(), i_vec1.end());
cout << "i_vec1 (shuffled): ";
printVectorElements(i_vec1);
cout << endl;
return EXIT_SUCCESS;
}
Output:
i_vec1 : 12; 32; 43; 53; 23; 65; 84;
i_vec1 (shuffled): 23; 53; 32; 84; 12; 65; 43;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook