How to Return a Pointer in C++
-
Use the
std::string::data
Function to Return Pointer From Function in C++ -
Use
&variable
Address-Of Notation to Return Pointer From Function in C++
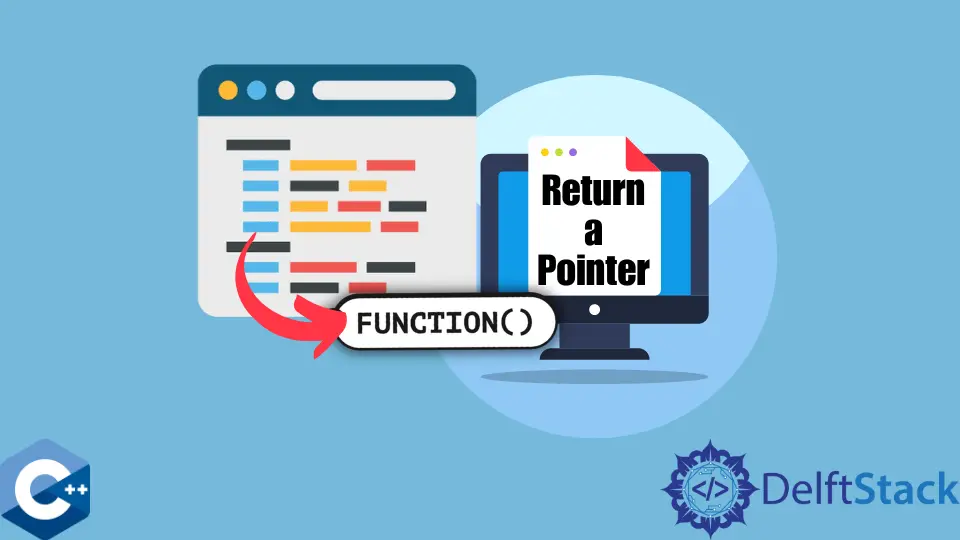
This article will explain several methods of how to return a pointer from a function in C++.
Use the std::string::data
Function to Return Pointer From Function in C++
Function return types generally fall into three categories: value, reference, or pointer. All of them have their optimal use cases in which
most of the performance is reached. Generally, returning pointers from functions are more common in the C language, as C++ provides a more eloquent concept - a reference for passing and returning using functions without copying the objects. Although there are scenarios that may utilize pointers better, so we demonstrate some examples of how to return pointer values for different data structures.
In the following example code, we implement a function that takes a reference to std::string
and returns the char*
, which is the underlying data type. Note that, std::string
class is used to contain the char
sequence stored contiguously. We can retrieve a pointer to the first character in the sequence using the data()
built-in function and pass after the return
statement. Finally, we can operate on the char
array using the returned pointer as needed.
#include <algorithm>
#include <iostream>
#include <iterator>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
char *reverseString(string &s) {
reverse(s.begin(), s.end());
return s.data();
}
int main() {
string str = "This string shall be reversed";
cout << str << endl;
cout << reverseString(str) << endl;
return EXIT_SUCCESS;
}
Output:
This string shall be reversed
desrever eb llahs gnirts sihT
Use &variable
Address-Of Notation to Return Pointer From Function in C++
&
address-of operator has its roots in back in C language and C++ uses it with the same semantics - to take the memory address of the object following it. Note though, it has slightly different behavior when the operator&
is overloaded (see the page). In this case, we demonstrate a function that takes an array as one of its arguments and returns the pointer to the same array. Since the pointer to the array object is the same as the pointer to the first element of the array, we can use the following notation - &arr[0]
to return the given array’s address.
#include <algorithm>
#include <iostream>
#include <iterator>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int *subtructArray(int arr[], size_t size, int subtrahend) {
for (size_t i = 0; i < size; ++i) {
arr[i] -= subtrahend;
}
return &arr[0];
}
int main() {
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int num = 3;
auto arr_size = sizeof c_array / sizeof c_array[0];
int *ptr = subtructArray(c_array, arr_size, num);
cout << "c_array = [ ";
for (int i = 0; i < arr_size; ++i) {
cout << ptr[i] << ", ";
}
cout << "\b\b ]" << endl;
return EXIT_SUCCESS;
}
Output:
c_array = [ -2, -1, 0, 1, 2, 3, 4, 5, 6, 7 ]
Alternatively, one can rewrite the previous code using just the variable name that the array is referred to in the function block. Note that, even though the function takes the int arr[]
as a parameter, the compiler does not copy the array underneath but rather passes the pointer to it. We can just pass the arr
name to the return
statement, and the pointer will be returned.
#include <algorithm>
#include <iostream>
#include <iterator>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int *subtructArray(int arr[], size_t size, int subtrahend) {
for (size_t i = 0; i < size; ++i) {
arr[i] -= subtrahend;
}
return arr;
}
int main() {
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int num = 3;
auto arr_size = sizeof c_array / sizeof c_array[0];
int *ptr = subtructArray(c_array, arr_size, num);
cout << "c_array = [ ";
for (int i = 0; i < arr_size; ++i) {
cout << ptr[i] << ", ";
}
cout << "\b\b ]" << endl;
return EXIT_SUCCESS;
}
Output:
c_array = [ -2, -1, 0, 1, 2, 3, 4, 5, 6, 7 ]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook