How to Remove Punctuation From a String in C++
-
Use
std::erase
andstd::remove_if
Functions to Remove Punctuation From a String in C++ - Use a Custom Function to Remove Punctuation From a String in C++
- Use the Iterative Method to Remove Punctuation From a String in C++
- Conclusion
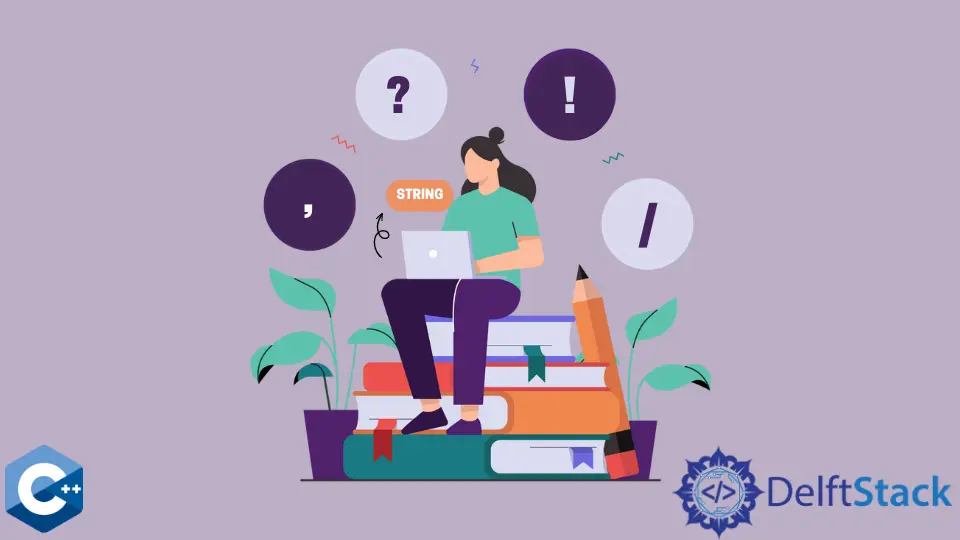
This article will explain several methods to remove punctuation from a string in C++. Each method showcases distinct techniques to achieve the same goal, providing flexibility and adaptability in handling strings with punctuation.
Use std::erase
and std::remove_if
Functions to Remove Punctuation From a String in C++
The std::remove_if
is part of the STL algorithms library, and it can remove all elements for which the given condition evaluates true. The condition should return the bool
value for each element in the range.
In this case, we pass the ispunct
function to check for the punctuation symbols. Notice that std::remove_if
returns a pass-the-end iterator for the new range of values, so we are chaining a call to it with the erase
method that results in the parsed string.
This solution works similarly to the erase-remove
idiom.
#include <algorithm>
#include <cctype>
#include <cstdlib>
#include <iostream>
#include <string>
int main() {
std::string text =
"Lorem ipsum, dolor sit! amet, consectetur? adipiscing elit. Ut "
"porttitor.";
std::cout << text << std::endl;
text.erase(std::remove_if(text.begin(), text.end(), ::ispunct), text.end());
std::cout << text << std::endl;
return EXIT_SUCCESS;
}
Output:
Lorem ipsum, dolor sit! amet, consectetur? adipiscing elit. Ut porttitor.
Lorem ipsum dolor sit amet consectetur adipiscing elit Ut porttitor
In this C++ code, we have a program that processes a given string to remove punctuation characters. We start by defining a string variable named text
containing a sample sentence with various punctuation marks.
We use the std::cout
statement to print the original text to the console. The main processing occurs in the next part, where we utilize the std::remove_if
algorithm along with the ::ispunct
function from the <cctype>
header, and this combination effectively removes all punctuation characters from the string.
The erase
function is then used to eliminate the positions in the string where punctuation characters were removed. Finally, we print the modified text without punctuation to the console using another std::cout
statement.
The EXIT_SUCCESS
constant from the <cstdlib>
header is returned to indicate successful execution. When we run this program, the output will consist of two lines: the original text and the text without punctuation, demonstrating the successful removal of punctuation characters from the given string.
Use a Custom Function to Remove Punctuation From a String in C++
Alternatively, one can move the previous method to a separate function, creating a local copy of the given string and operating on it while returning the parsed value to the caller code. This function can be utilized to extend the functionality to support different character groups or even pass the custom predicate function for the third parameter of the remove_if
algorithm.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
string removePunctuation(const string& s) {
string tmp(s);
tmp.erase(std::remove_if(tmp.begin(), tmp.end(), ::ispunct), tmp.end());
return tmp;
}
int main() {
string text =
"Lorem ipsum, dolor sit! amet, consectetur? adipiscing elit. Ut "
"porttitor.";
cout << "Original Text: " << text << endl;
string parsed_text = removePunctuation(text);
cout << "Text without Punctuation: " << parsed_text << endl;
return EXIT_SUCCESS;
}
Output:
Original Text: Lorem ipsum, dolor sit! amet, consectetur? adipiscing elit. Ut porttitor.
Text without Punctuation: Lorem ipsum dolor sit amet consectetur adipiscing elit Ut porttitor
In this C++ code, we begin by including the necessary headers and defining the main function. We also declare a utility function called removePunctuation
that takes a constant reference to a string and returns the string’s modified version with all punctuation characters removed.
Inside the removePunctuation
function, we create a temporary string tmp
initialized with the input string and then use the std::remove_if
algorithm along with ::ispunct
to erase punctuation characters from tmp
. The main function initializes a string variable named text
with a sample sentence containing various punctuation marks.
We print the original text to the console using std::cout
. Next, we call the removePunctuation
function to obtain a new string without punctuation, assigning it to the variable parsed_text
.
Finally, we print the modified text without punctuation to the console, labeling it as Text without Punctuation:
. The program returns EXIT_SUCCESS
to indicate successful execution.
When we run this program, the output consists of two lines: the original text and the modified text without punctuation, demonstrating the successful removal of punctuation characters from the given string.
Note: Ensure that your C++ compiler supports the C++11 standard or later, as the code uses features introduced in C++11.
Use the Iterative Method to Remove Punctuation From a String in C++
Another alternative is to implement a separate function that iterates through every character of the string. The function takes the string by reference and utilizes the for
loop to traverse the string.
In each iteration, ispunct
is called to check if the character is a punctuation symbol or not. Note that the len
variable is assigned the string’s size on each matching condition because the original string object gets modified by the erase
function, and the loop needs to renew the count.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void removeSpaces2(string& s) {
for (int i = 0, len = s.size(); i < len; i++) {
if (ispunct(s[i])) {
s.erase(i--, 1);
len = s.size();
}
}
}
int main() {
string text =
"Lorem ipsum, dolor sit! amet, consectetur? adipiscing elit. Ut "
"porttitor.";
cout << text << endl;
removeSpaces2(text);
cout << text << endl;
return EXIT_SUCCESS;
}
Output:
Lorem ipsum, dolor sit! amet, consectetur? adipiscing elit. Ut porttitor.
Lorem ipsum dolor sit amet consectetur adipiscing elit Ut porttitor
In this C++ code, we first include the necessary headers and declare the main function. We also declare a utility function called removeSpaces2
, which takes a reference to a string and removes punctuation characters from it.
Inside the function, we use a loop to iterate through the characters of the string. If a punctuation character is encountered, we erase it from the string, and we decrement the loop index (i
) to recheck the current position, and this ensures that consecutive punctuation characters are handled correctly.
The main function initializes a string variable named text
with a sample sentence containing various punctuation marks. We print the original text to the console using std::cout
.
Next, we call the removeSpaces2
function to modify the string in place by removing punctuation characters. Finally, we print the modified text to the console.
When we run this program, the output consists of two lines: the original text and the modified text without punctuation, demonstrating the successful removal of punctuation characters from the given string.
Conclusion
In conclusion, we’ve explored multiple approaches to remove punctuation from strings in C++. Whether leveraging the power of STL algorithms or implementing custom functions for more specific needs, these methods offer diverse solutions for different programming scenarios.
The provided code snippets demonstrate the effectiveness of each technique, producing modified strings without punctuation. By understanding and employing these methods, developers can choose the approach that best fits their requirements, contributing to cleaner and more readable code.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++