Pointer to an Array in C++
- Use Pointer to An Array to Swap Elements in Different Arrays in C++
- Use Array Reference to Pass 2D Array to a Function in C++
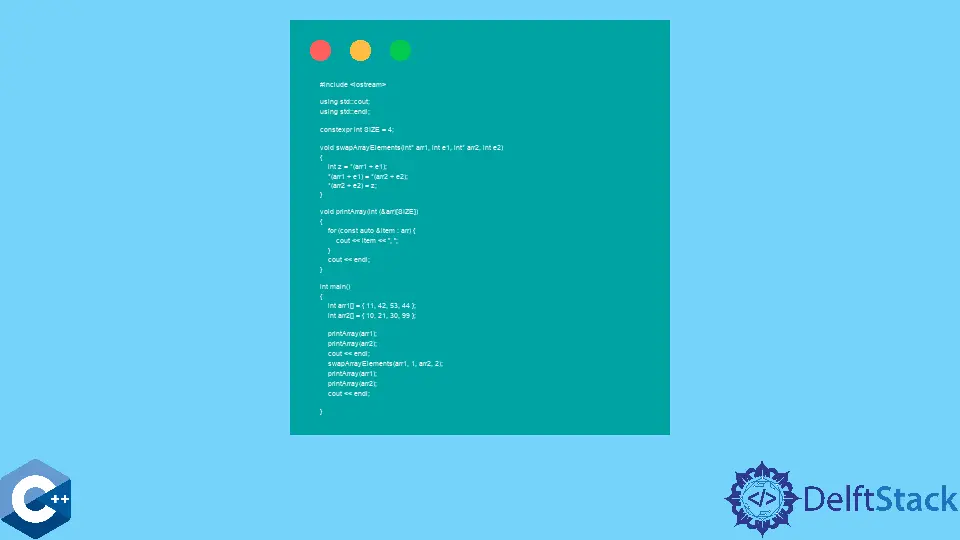
This article will demonstrate multiple methods about how to use a pointer to an array in C++.
Use Pointer to An Array to Swap Elements in Different Arrays in C++
The pointer is one of the core elements of low-level programming. Even though C++ tries to substitute some of their use cases with references, pointers are still only built-in data types that can be utilized to handle memory directly. Note that, C-style array is essentially a pointer to the starting element and since it has a fixed size compiler automatically handles accesses with []
notation internally. In the following example code, we implement a function that swaps two elements from different integer arrays. Notice that the function prototype takes two int*
pointers to denote the elements which need to be swapped. The pointer makes it possible to access the given elements’ memory location directly instead of merely modifying the local instances of the elements.
#include <iostream>
using std::cout;
using std::endl;
constexpr int SIZE = 4;
void swapArrayElements(int* e1, int* e2) {
int z = *e1;
*e1 = *e2;
*e2 = z;
}
void printArray(int (&arr)[SIZE]) {
for (const auto& item : arr) {
cout << item << ", ";
}
cout << endl;
}
int main() {
int arr1[] = {11, 42, 53, 44};
int arr2[] = {10, 21, 30, 99};
printArray(arr1);
printArray(arr2);
cout << endl;
swapArrayElements(&arr1[0], &arr2[3]);
printArray(arr1);
printArray(arr2);
cout << endl;
return EXIT_SUCCESS;
}
Output:
11, 42, 53, 44,
10, 21, 30, 99,
99, 42, 53, 44,
10, 21, 30, 11,
Alternatively, we can pass array pointers and element positions separately. This method is not inherently better than the previous, but it’s here to demonstrate the different language notations by which a pointer to an array can be utilized. In this case, two function arguments are added to specify the position of the elements that need to be swapped. Meanwhile, the element access is done using the so-called pointer arithmetic
, which can have a quite cumbersome notation. Note that incrementing the pointer to the array by an integer value is equal to incrementing the pointer to an element type, which moves the pointer value by the sizeof
bytes of the object type.
#include <iostream>
using std::cout;
using std::endl;
constexpr int SIZE = 4;
void swapArrayElements(int* arr1, int e1, int* arr2, int e2) {
int z = *(arr1 + e1);
*(arr1 + e1) = *(arr2 + e2);
*(arr2 + e2) = z;
}
void printArray(int (&arr)[SIZE]) {
for (const auto& item : arr) {
cout << item << ", ";
}
cout << endl;
}
int main() {
int arr1[] = {11, 42, 53, 44};
int arr2[] = {10, 21, 30, 99};
printArray(arr1);
printArray(arr2);
cout << endl;
swapArrayElements(arr1, 1, arr2, 2);
printArray(arr1);
printArray(arr2);
cout << endl;
}
Output:
99, 42, 53, 44,
10, 21, 30, 11,
99, 30, 53, 44,
10, 21, 42, 11,
Use Array Reference to Pass 2D Array to a Function in C++
Passing the two-dimensional C-style arrays can get quite ugly, so it’s preferable to use reference notation instead. Mind though, the following example functions would be suitable for predefined length-sized arrays so that the function prototype includes the size value for each dimension. On the upside, this gives a possibility to utilize a range-based for
loop for element traversal.
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
constexpr int SIZE = 4;
void MultiplyArrayByTwo(int (&arr)[SIZE][SIZE]) {
for (auto& i : arr) {
for (int& j : i) j *= 2;
}
}
void printArray(int (&arr)[SIZE][SIZE]) {
for (auto& i : arr) {
cout << " [ ";
for (int j : i) {
cout << setw(2) << j << ", ";
}
cout << "]" << endl;
}
}
int main() {
int array_2d[SIZE][SIZE] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
printArray(array_2d);
MultiplyArrayByTwo(array_2d);
cout << endl;
printArray(array_2d);
return EXIT_SUCCESS;
}
Output:
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook