How to Pass Pointer by Reference in C++
- The Significance of Passing Pointers by Reference
- Passing a Pointer by Reference Using Pointers
- Passing Pointers by Reference with Smart Pointers
- Passing Pointers by Reference in C++ through Returning a Pointer
- Conclusion
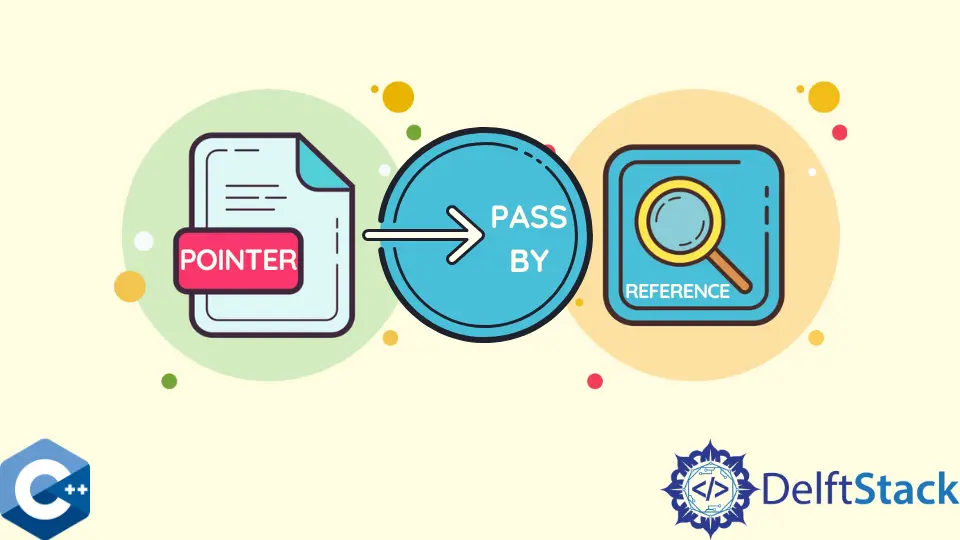
This article will demonstrate multiple methods on how to pass a pointer by reference in C++.
The Significance of Passing Pointers by Reference
Understanding the significance of passing pointers by reference is crucial in C++ programming for several reasons. Firstly, it allows functions to modify the original pointer, facilitating dynamic changes to memory addresses.
This is particularly valuable when dealing with dynamic memory allocation or scenarios where a function needs to reassign the pointer to a new memory location.
Moreover, passing pointers by reference enhances code clarity and readability. It ensures that modifications made to the pointer within a function persist outside its scope, making the code more predictable and easier to maintain.
Additionally, this technique is essential for efficient memory management. By passing pointers by reference, developers can prevent memory leaks and improve the overall stability of their programs.
It empowers programmers to have better control over dynamic memory, leading to more robust and reliable C++ applications.
Passing a Pointer by Reference Using Pointers
In the realm of C++ programming, the ability to efficiently manage and manipulate pointers is a crucial skill. Pointers provide a way to directly access and modify memory, offering flexibility and control over dynamic data structures.
One powerful technique often employed is passing pointers by reference, allowing functions to modify the original pointer itself. In this article, we will delve into the method of passing pointers by reference using pointers, exploring their significance, and providing a detailed walkthrough of a working example.
Code Example:
#include <iostream>
void modifyPointer(int*& ptr) {
// Allocate memory for an integer and assign the address to the pointer
ptr = new int(42);
}
int main() {
// Declare a pointer and initialize it to nullptr
int* myPointer = nullptr;
// Call the function to modify the pointer
modifyPointer(myPointer);
// Access the value through the modified pointer
std::cout << "Modified Pointer Value: " << *myPointer << std::endl;
// Deallocate the memory to prevent memory leaks
delete myPointer;
return 0;
}
The function modifyPointer
takes a reference to a pointer (int*& ptr
) as its parameter, ensuring that any changes made to the pointer inside the function persist outside the function scope. Within the function, memory is allocated for an integer using new int(42)
, and the address of this memory is assigned to the pointer ptr
.
The value 42
is used for demonstration purposes and can be replaced with any desired value. In the main
function, a pointer myPointer
is declared and initialized to nullptr
to avoid using uninitialized pointers.
The modifyPointer
function is then called, passing myPointer
as an argument, which modifies the original pointer by assigning it a new memory location. The value stored at the modified memory location is accessed using *myPointer
and printed to the console.
In order to prevent memory leaks, the allocated memory is deallocated using delete myPointer
.
Output:
This output demonstrates that the pointer myPointer
has been successfully modified within the function, showcasing the effectiveness of passing pointers by reference using the pointer manipulation method.
In conclusion, passing pointers by reference using pointers in C++ is a powerful technique that enhances the control and flexibility of dynamic memory management. This method allows functions to directly manipulate the original pointer, providing a seamless way to update memory addresses.
By mastering this concept, programmers can unlock new possibilities for efficient and dynamic memory utilization in their C++ applications.
Passing Pointers by Reference with Smart Pointers
Smart pointers, introduced in C++11, are intelligent constructs that not only hold the memory address but also manage the memory automatically. Unlike raw pointers, which require manual memory deallocation, smart pointers handle memory release automatically when it’s no longer needed, leading to safer and more reliable code.
The concept of passing pointers by reference using std::shared_ptr
or std::unique_ptr
is an advanced technique that not only allows for dynamic memory manipulation but also ensures automatic memory deallocation, mitigating the risks of memory leaks.
Passing pointers by reference using std::shared_ptr
or std::unique_ptr
builds upon this foundation, allowing functions to modify the smart pointer itself. This method enhances code clarity, reduces the chances of memory leaks, and provides a more robust approach to dynamic memory allocation and deallocation.
Code Example:
#include <iostream>
#include <memory>
void modifySharedPtr(std::shared_ptr<int>& ptr) {
// Allocate memory for an integer and assign the address to the shared_ptr
ptr = std::make_shared<int>(42);
}
int main() {
// Declare a shared_ptr and initialize it to nullptr
std::shared_ptr<int> mySharedPtr = nullptr;
// Call the function to modify the shared_ptr
modifySharedPtr(mySharedPtr);
// Access the value through the modified shared_ptr
std::cout << "Modified Shared Pointer Value: " << *mySharedPtr << std::endl;
// No need to manually deallocate memory; shared_ptr handles it automatically
return 0;
}
The function modifySharedPtr
plays a key role in passing a reference to a shared pointer (std::shared_ptr<int>& ptr
). This reference ensures that any alterations made to the shared pointer within the function persist beyond its scope.
Inside the function, memory allocation occurs for an integer using std::make_shared<int>(42)
, and the shared pointer ptr
is assigned the address of this memory. The value 42
is used for illustration and can be substituted with any desired value.
In the main
function, a shared pointer mySharedPtr
is declared and initialized to nullptr
. This initialization is automatic for smart pointers.
Upon calling the modifySharedPtr
function with mySharedPtr
as an argument, the original shared pointer is modified, pointing to a new memory location. Accessing the modified shared pointer is facilitated by using *mySharedPtr
to retrieve the value stored at the updated memory location, which is then printed to the console.
Unlike raw pointers, there is no need to explicitly free memory, as the shared pointer automatically manages memory cleanup when it goes out of scope. This streamlined approach enhances code safety and efficiency, making dynamic memory management in C++ more intuitive and robust.
Output:
This output demonstrates that the shared pointer mySharedPtr
has been successfully modified within the function, showcasing the effectiveness of passing smart pointers by reference using the std::shared_ptr
method. The automatic memory deallocation ensures a safer and more efficient approach to dynamic memory management.
Passing Pointers by Reference in C++ through Returning a Pointer
One powerful technique for managing memory and passing pointers by reference involves returning a pointer from a function. This approach not only allows functions to modify the original pointer but also provides a seamless way to dynamically allocate memory.
Passing pointers by reference through returning a pointer is a versatile technique that enables functions to alter the original pointer passed to them. By returning a pointer from a function, developers can dynamically allocate memory, modify the original pointer, and achieve a more flexible and controlled approach to memory management.
This method is especially valuable when dealing with scenarios where the function needs to allocate memory and update the caller’s pointer.
Code Example:
#include <iostream>
int* allocateAndModifyPointer() {
// Allocate memory for an integer
int* ptr = new int(42);
// Modify the value at the allocated memory
*ptr = 99;
// Return the pointer
return ptr;
}
int main() {
// Declare a pointer and initialize it to nullptr
int* myPointer = nullptr;
// Call the function to allocate and modify the pointer
myPointer = allocateAndModifyPointer();
// Access the value through the modified pointer
std::cout << "Modified Pointer Value: " << *myPointer << std::endl;
// Deallocate the memory to prevent memory leaks
delete myPointer;
return 0;
}
In the function allocateAndModifyPointer
, dynamic memory is allocated for an integer, initially set to 42
, and later modified to 99
. The function then returns the pointer to the dynamically allocated memory.
Moving to the main
function, a pointer myPointer
is declared and initialized to nullptr
to ensure it’s not pointing to any specific memory address initially. Upon calling the allocateAndModifyPointer
function, the returned pointer modifies the original pointer, directing it to the dynamically allocated memory.
The modified pointer is then used to access and print the value stored at the updated memory location. In order to prevent memory leaks, the allocated memory is deallocated using delete
.
This concise process showcases the effectiveness of returning a pointer to pass pointers by reference in C++, providing a flexible and controlled approach to dynamic memory management. The output, in this case, will be Modified Pointer Value: 99
.
Output:
This output demonstrates that the pointer myPointer
has been successfully modified within the function allocateAndModifyPointer
. Returning a pointer from the function has allowed us to dynamically allocate and manipulate memory, showcasing the effectiveness of passing pointers by reference through returning a pointer in C++.
Conclusion
Understanding the significance of passing pointers by reference in C++ is crucial for effective memory management and dynamic programming. This technique empowers developers to modify original pointers, facilitating flexibility in memory allocation and manipulation.
Throughout the article, we explored various methods, each offering unique advantages. Whether using the traditional pointer reference approach or leveraging smart pointers, these methods contribute to safer and more efficient C++ programming, emphasizing the importance of mastering pointer manipulation for dynamic and reliable applications.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook