Function Overloading VS Overriding in C++
- What is Function Overloading?
- What is Function Overriding?
- Key Differences Between Function Overloading and Overriding
- Conclusion
- FAQ
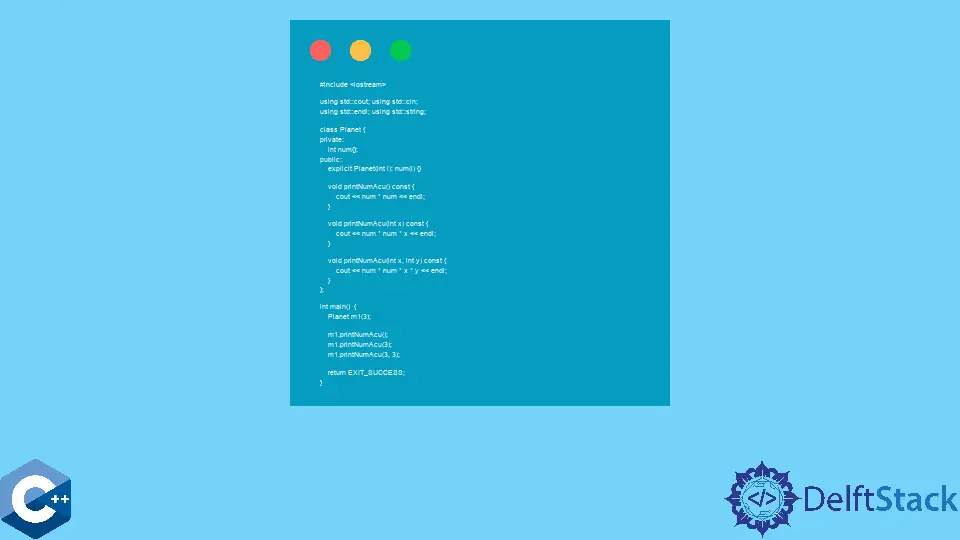
Understanding the differences between function overloading and function overriding is crucial for any C++ programmer. Both concepts are essential aspects of C++ that allow for more flexible and efficient code. While they may sound similar, they serve different purposes and are used in different contexts. Function overloading allows multiple functions with the same name but different parameters, enhancing readability and usability. On the other hand, function overriding is a feature that allows a derived class to provide a specific implementation of a function that is already defined in its base class.
In this article, we’ll explore both concepts in detail, providing clear examples and explanations to help you grasp their differences and applications.
What is Function Overloading?
Function overloading is a feature in C++ that allows you to define multiple functions with the same name but different parameter lists. This means that you can have several functions performing similar tasks but with different types or numbers of parameters. The compiler determines which function to execute based on the arguments passed during the function call.
Here’s a simple example of function overloading:
#include <iostream>
using namespace std;
class Math {
public:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
int add(int a, int b, int c) {
return a + b + c;
}
};
int main() {
Math math;
cout << math.add(5, 10) << endl;
cout << math.add(5.5, 10.5) << endl;
cout << math.add(5, 10, 15) << endl;
return 0;
}
Output:
15
16
30
In this example, the Math
class has three add
methods, each with different parameters. The first function adds two integers, the second adds two doubles, and the third adds three integers. The appropriate function is called based on the arguments provided, demonstrating how function overloading improves code clarity and usability.
What is Function Overriding?
Function overriding occurs when a derived class provides a specific implementation of a function that is already defined in its base class. This allows the derived class to inherit the base class’s properties while customizing the behavior of specific functions. Function overriding is essential for achieving polymorphism in C++.
Here’s an illustrative example of function overriding:
#include <iostream>
using namespace std;
class Animal {
public:
virtual void sound() {
cout << "Some generic animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Woof!" << endl;
}
};
class Cat : public Animal {
public:
void sound() override {
cout << "Meow!" << endl;
}
};
int main() {
Animal* animal1 = new Dog();
Animal* animal2 = new Cat();
animal1->sound();
animal2->sound();
delete animal1;
delete animal2;
return 0;
}
Output:
Woof!
Meow!
In this code, the Animal
class has a virtual function called sound()
. The Dog
and Cat
classes override this function to provide their specific implementations. When we call sound()
on pointers to Animal
, the appropriate function for Dog
or Cat
is executed, demonstrating polymorphism and the power of function overriding in C++.
Key Differences Between Function Overloading and Overriding
Although both function overloading and overriding allow for flexibility in how functions are defined and used, they have distinct differences:
-
Definition: Overloading allows multiple functions with the same name but different parameters, while overriding allows a derived class to provide a new implementation of a base class function.
-
Polymorphism: Overloading is a compile-time polymorphism, meaning the resolution happens at compile time. In contrast, overriding is a runtime polymorphism, resolved during program execution.
-
Inheritance: Overloading can occur within a single class, while overriding requires inheritance, as it involves a base class and a derived class.
-
Virtual Functions: Overriding is closely associated with virtual functions, which allow derived classes to redefine base class methods. Overloading does not involve virtual functions.
Understanding these differences is crucial for writing efficient and maintainable C++ code, as they dictate how functions can be structured and utilized in your programs.
Conclusion
In conclusion, both function overloading and function overriding are vital features in C++ that enhance the language’s flexibility and power. Function overloading allows for multiple functions with the same name but different parameters, improving code readability and usability. Function overriding enables derived classes to customize the behavior of inherited functions, supporting polymorphism. By mastering these concepts, C++ programmers can write cleaner, more efficient code that is easier to maintain and understand.
FAQ
-
What is function overloading in C++?
Function overloading allows multiple functions with the same name but different parameter lists to coexist in the same scope. -
What is function overriding in C++?
Function overriding allows a derived class to provide a specific implementation of a function that is already defined in its base class. -
How does function overloading differ from function overriding?
Overloading occurs within the same class and is resolved at compile time, while overriding involves a base class and a derived class and is resolved at runtime. -
Can we overload constructors in C++?
Yes, constructors can be overloaded in C++, allowing multiple constructors with different parameter lists in the same class.
- Is it necessary to use the
virtual
keyword for function overriding?
Yes, thevirtual
keyword must be used in the base class to allow derived classes to override the function.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook