How to Count Number of Digits in a Number in C++
- Method 1: Using a Loop
- Method 2: Using Logarithms
- Method 3: Converting to String
-
Use
std::string::erase
andstd::remove_if
Methods - Conclusion
- FAQ
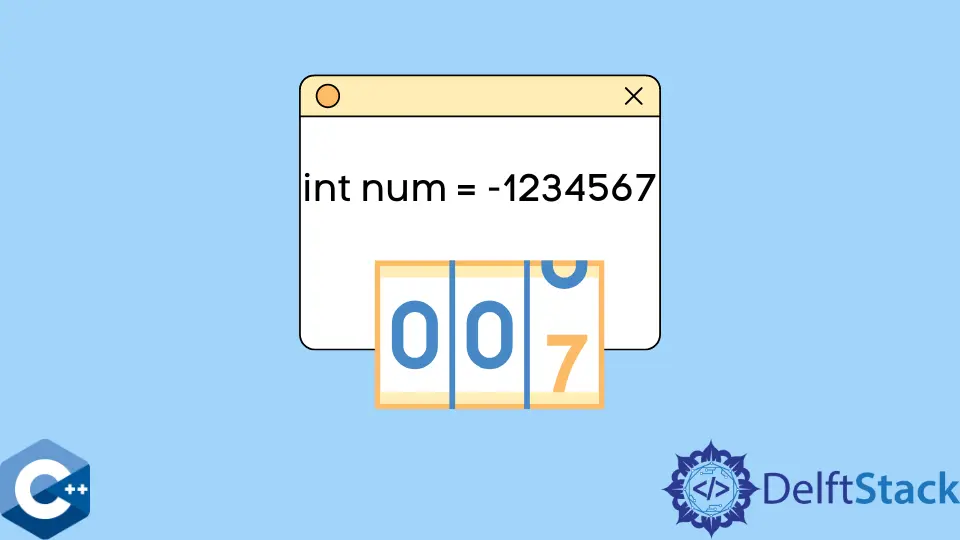
Counting the number of digits in a number is a fundamental task in programming, often used in various applications ranging from data validation to mathematical calculations. In C++, this can be accomplished in several ways, allowing developers to choose the method that best fits their needs. Whether you’re a beginner or an experienced programmer, understanding how to count the digits in a number can enhance your coding skills and improve your problem-solving abilities.
In this article, we will explore different methods to achieve this in C++, providing clear examples and explanations to help you grasp the concepts easily. Let’s dive in!
Method 1: Using a Loop
One of the most straightforward ways to count the number of digits in a number is by using a loop. This method involves repeatedly dividing the number by 10 until it becomes zero. Each division represents a digit, and we can increment a counter accordingly. Here’s how you can implement this in C++:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
int count = 0;
while (number != 0) {
number /= 10;
count++;
}
cout << "Number of digits: " << count << endl;
return 0;
}
Output:
Number of digits: 3
In this code snippet, we start by prompting the user to enter a number. We initialize a counter variable, count
, to zero. The while
loop continues to execute as long as the number is not zero. Inside the loop, we divide the number by 10, effectively removing the last digit, and increment the counter. Once the loop completes, we print the total count of digits. This method is efficient and easy to understand, making it ideal for beginners.
Method 2: Using Logarithms
Another elegant way to count the number of digits in a number is by using logarithms. The logarithm base 10 of a number gives us the power of 10 that is closest to that number, which directly correlates to the number of digits. Here’s how you can use this method in C++:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
int count = (number == 0) ? 1 : log10(abs(number)) + 1;
cout << "Number of digits: " << count << endl;
return 0;
}
Output:
Number of digits: 3
In this approach, we first check if the number is zero, as it has one digit. For any other number, we use log10
to find the logarithm base 10 of the absolute value of the number. Adding 1 gives us the total number of digits. This method is particularly efficient, as it runs in constant time, making it suitable for large numbers. However, it is important to handle negative numbers and zero properly, as shown in the code.
Method 3: Converting to String
Converting a number to a string is another effective method to count its digits. This approach leverages the string representation of the number, allowing us to simply measure the length of the string. Here’s how you can implement this in C++:
#include <iostream>
#include <string>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
string str = to_string(abs(number));
int count = str.length();
cout << "Number of digits: " << count << endl;
return 0;
}
Output:
Number of digits: 3
In this example, we first convert the number to its absolute value to avoid counting the negative sign as a digit. We then use to_string
to convert the number into a string. The length
function gives us the count of digits directly. This method is very intuitive and works well for most applications, especially when dealing with user input or displaying results. However, it may involve a slight overhead due to the conversion process.
Use std::string::erase
and std::remove_if
Methods
The previous example provides a fully sufficient solution for the above problem, but one can over-engineer the countDigits
using the std::string::erase
and std::remove_if
functions combination to remove any non-number symbols. Note also that this method may be the stepping stone to implement a function that can work with floating-point values. Mind though, the following sample code is not compatible with floating-point values.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
template <typename T>
size_t countDigits(T n) {
string tmp;
tmp = to_string(n);
tmp.erase(std::remove_if(tmp.begin(), tmp.end(), ispunct), tmp.end());
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
Output:
number of digits in 1234567 = 7
number of digits in -1234567 = 7
Conclusion
Counting the number of digits in a number in C++ can be achieved through various methods, each with its own advantages and use cases. Whether you prefer the simplicity of loops, the efficiency of logarithms, or the convenience of string manipulation, understanding these techniques will enhance your programming toolkit. As you practice these methods, you’ll become more adept at tackling similar challenges in your coding journey. So, go ahead and experiment with these approaches to see which one suits your style best!
FAQ
-
How do I count digits in a negative number?
You can use the absolute value of the number to count digits, as shown in the examples. -
Is there a method that works for floating-point numbers?
Yes, you can convert the number to a string and then count the digits, excluding the decimal point. -
Can I count digits in a very large number?
For extremely large numbers, consider using data types likelong long
or libraries designed for big integers. -
What if I want to count digits without using loops?
You can use logarithms or string conversion methods as alternatives to loops. -
Are there any built-in functions in C++ for this purpose?
C++ does not have a built-in function specifically for counting digits, but you can implement it using the methods discussed.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook