Multiple Inheritance in C++
- Understanding Multiple Inheritance
- The Diamond Problem
- Virtual Inheritance
- Best Practices for Using Multiple Inheritance
- Conclusion
- FAQ
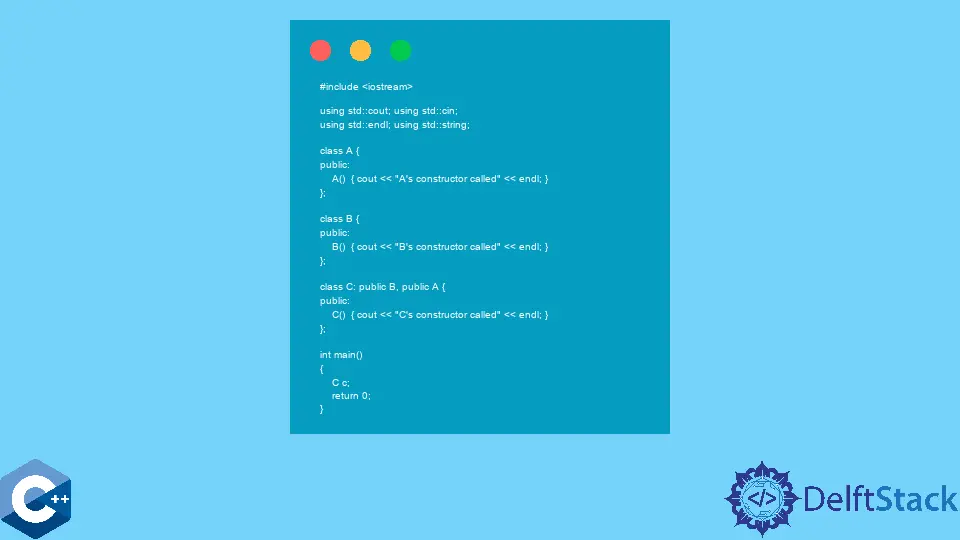
Multiple inheritance is a powerful feature in C++ that allows a class to inherit from more than one base class. This capability can lead to more versatile and complex class designs, enabling developers to create robust applications. However, while multiple inheritance can enhance the functionality of your programs, it also introduces complexity, such as the potential for ambiguity and the diamond problem.
In this article, we will explore how to effectively use multiple inheritance in C++, providing clear examples and explanations to help you understand this concept. Whether you’re a beginner or an experienced programmer, this guide will equip you with the knowledge you need to implement multiple inheritance in your C++ projects.
Understanding Multiple Inheritance
In C++, multiple inheritance allows a derived class to inherit from two or more base classes. This is different from single inheritance, where a class can only inherit from one base class. The syntax for multiple inheritance is straightforward. You simply specify multiple base classes in the class declaration, separated by commas.
Here’s a simple example:
#include <iostream>
using namespace std;
class Base1 {
public:
void displayBase1() {
cout << "Base1 function called." << endl;
}
};
class Base2 {
public:
void displayBase2() {
cout << "Base2 function called." << endl;
}
};
class Derived : public Base1, public Base2 {
};
int main() {
Derived obj;
obj.displayBase1();
obj.displayBase2();
return 0;
}
Output:
Base1 function called.
Base2 function called.
In this example, Derived
inherits from both Base1
and Base2
. When we create an object of Derived
, we can call methods from both base classes. This demonstrates the core concept of multiple inheritance.
The Diamond Problem
While multiple inheritance can be beneficial, it can also lead to complications, particularly the diamond problem. This occurs when two base classes inherit from the same parent class, and a derived class inherits from both of these base classes. This can create ambiguity when trying to access members of the common base class.
Consider the following example:
#include <iostream>
using namespace std;
class A {
public:
void show() {
cout << "Class A" << endl;
}
};
class B : public A {
};
class C : public A {
};
class D : public B, public C {
};
int main() {
D obj;
obj.B::show();
obj.C::show();
return 0;
}
Output:
Class A
Class A
In this case, D
inherits from both B
and C
, which both inherit from A
. When we call show()
from an object of D
, we must specify which base class’s method to use, as both B
and C
have access to A
. This is how we resolve the ambiguity introduced by the diamond problem.
Virtual Inheritance
To address the diamond problem, C++ provides a feature called virtual inheritance. By declaring the base class as virtual, you ensure that only one instance of the base class is created, regardless of how many times it is inherited.
Here’s how to implement virtual inheritance:
#include <iostream>
using namespace std;
class A {
public:
void show() {
cout << "Class A" << endl;
}
};
class B : virtual public A {
};
class C : virtual public A {
};
class D : public B, public C {
};
int main() {
D obj;
obj.show();
return 0;
}
Output:
Class A
In this example, A
is virtually inherited by both B
and C
. When we create an object of D
and call show()
, it directly accesses the single instance of A
. This eliminates the ambiguity and provides a clear path to the base class’s methods.
Best Practices for Using Multiple Inheritance
While multiple inheritance can be advantageous, it’s essential to follow best practices to avoid common pitfalls. Here are some tips:
- Use When Necessary: Only use multiple inheritance when it adds clear value to your design. If a single inheritance structure suffices, prefer that.
- Keep Hierarchies Simple: Complex hierarchies can lead to confusion. Aim for simplicity in your class structures.
- Document Your Code: Given the complexity that can arise with multiple inheritance, thorough documentation is crucial. Clearly explain the relationships between classes.
- Prefer Composition Over Inheritance: In many cases, composition can achieve the same results without the complications of multiple inheritance.
By adhering to these best practices, you can leverage multiple inheritance effectively while minimizing potential issues.
Conclusion
Multiple inheritance in C++ is a powerful feature that enables developers to create versatile and complex class structures. However, it comes with challenges, such as the diamond problem and potential ambiguity. By understanding these concepts and implementing best practices, you can harness the power of multiple inheritance while maintaining clarity in your code. Whether you are building simple applications or complex systems, mastering multiple inheritance will enhance your C++ programming skills.
FAQ
-
What is multiple inheritance in C++?
Multiple inheritance allows a derived class to inherit from two or more base classes, providing more functionality and flexibility. -
What is the diamond problem?
The diamond problem occurs when two base classes inherit from the same parent class, creating ambiguity in the derived class regarding which base class’s member to access. -
How can virtual inheritance solve the diamond problem?
Virtual inheritance ensures that only one instance of a base class is created, eliminating ambiguity when accessing members of that base class. -
Should I always use multiple inheritance?
No, use multiple inheritance only when it adds clear value to your design. In many cases, single inheritance or composition may be more appropriate. -
How can I avoid issues with multiple inheritance?
Follow best practices such as keeping hierarchies simple, documenting your code, and preferring composition over inheritance when possible.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook