How to Initialize Static Variables in C++ Class
- Understanding Static Variables
- Initializing Static Variables in C++ Class
- Best Practices for Static Variable Initialization
- Conclusion
- FAQ
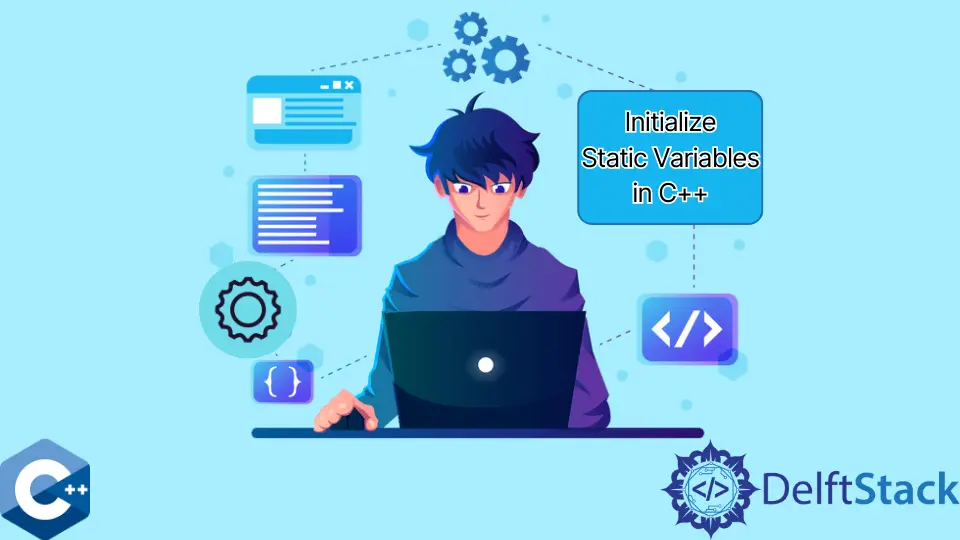
The initialization of static variables in a C++ class is a fundamental concept that every programmer should grasp. Static variables are shared across all instances of a class, meaning they retain their value even when no instances of the class exist. This characteristic makes them particularly useful for counting instances or maintaining state information that should be accessible across different objects.
In this article, we will explore how to properly initialize static variables within a C++ class, including best practices and common pitfalls to avoid. By the end, you’ll have a solid understanding of how to manage static variables effectively in your C++ applications.
Understanding Static Variables
Static variables in C++ are declared using the static
keyword. They are unique to the class rather than individual objects. This means that all instances of the class share the same static variable. When you initialize a static variable, it is done outside the class definition, typically in a source file. This is crucial for memory management and ensuring that the variable maintains its value throughout the program’s lifecycle.
Here’s a simple example to illustrate this:
#include <iostream>
class Counter {
public:
static int count;
Counter() {
count++;
}
};
int Counter::count = 0;
int main() {
Counter c1;
Counter c2;
std::cout << "Count: " << Counter::count << std::endl;
return 0;
}
In this example, we define a static variable count
within the Counter
class. Each time a new Counter
object is created, count
is incremented. The static variable is initialized to zero outside the class definition.
Output:
Count: 2
This demonstrates that the static variable retains its value across multiple instances of the class.
Initializing Static Variables in C++ Class
When you declare a static variable in a class, you must define it outside the class. This definition allocates storage for the variable and allows you to initialize it. Here’s a more detailed breakdown of how to do this correctly:
- Declaration in the Class: You declare the static variable within the class definition.
- Definition Outside the Class: You then define and initialize the static variable outside the class.
Let’s see an example:
#include <iostream>
class MyClass {
public:
static int staticVar;
MyClass() {
staticVar++;
}
};
// Definition and initialization of static variable
int MyClass::staticVar = 10;
int main() {
MyClass obj1;
MyClass obj2;
std::cout << "Static Variable: " << MyClass::staticVar << std::endl;
return 0;
}
In this code, staticVar
is initialized to 10
. Each time an object of MyClass
is created, staticVar
is incremented by 1.
Output:
Static Variable: 12
This shows that the static variable is shared among all instances of MyClass
.
Best Practices for Static Variable Initialization
When working with static variables, it’s essential to follow best practices to avoid common pitfalls. Here are some tips to keep in mind:
- Initialize Once: Always initialize your static variables only once outside the class definition. This prevents multiple definitions and ensures consistent behavior.
- Use Meaningful Names: Choose descriptive names for static variables to clarify their purpose.
- Thread Safety: If your application is multi-threaded, consider thread safety when accessing static variables. You may need to use mutexes or other synchronization mechanisms to prevent race conditions.
- Memory Management: Be aware that static variables persist for the lifetime of the program. Ensure that they are used judiciously to avoid unnecessary memory consumption.
Here’s an example illustrating these best practices:
#include <iostream>
#include <mutex>
class ThreadSafeCounter {
public:
static int count;
static std::mutex mtx;
ThreadSafeCounter() {
std::lock_guard<std::mutex> lock(mtx);
count++;
}
};
int ThreadSafeCounter::count = 0;
std::mutex ThreadSafeCounter::mtx;
int main() {
ThreadSafeCounter t1;
ThreadSafeCounter t2;
std::cout << "Thread-Safe Count: " << ThreadSafeCounter::count << std::endl;
return 0;
}
In this example, we use a mutex to ensure that the static variable count
is updated safely in a multi-threaded environment.
Output:
Thread-Safe Count: 2
Following these best practices will help you manage static variables effectively and avoid potential issues.
Conclusion
In conclusion, initializing static variables in a C++ class is a straightforward yet crucial aspect of programming in C++. By understanding how to declare, define, and manage these variables, you can create more efficient and organized code. Remember to follow best practices to ensure that your static variables serve their purpose without causing unintended side effects. With the right approach, static variables can be powerful tools in your C++ programming arsenal.
FAQ
-
What is a static variable in C++?
A static variable in C++ is a variable that retains its value across multiple instances of a class and is shared among all instances. -
How do I declare a static variable in a class?
You declare a static variable in a class using thestatic
keyword within the class definition. -
Can I initialize a static variable inside the class?
No, you must define and initialize a static variable outside the class definition. -
What happens if I don’t initialize a static variable?
If you do not initialize a static variable, it will have a default value of zero for integral types or null for pointers. -
Are static variables thread-safe?
No, static variables are not inherently thread-safe. You should use synchronization techniques like mutexes when accessing them in a multi-threaded environment.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook