How to Get Class Name in C++
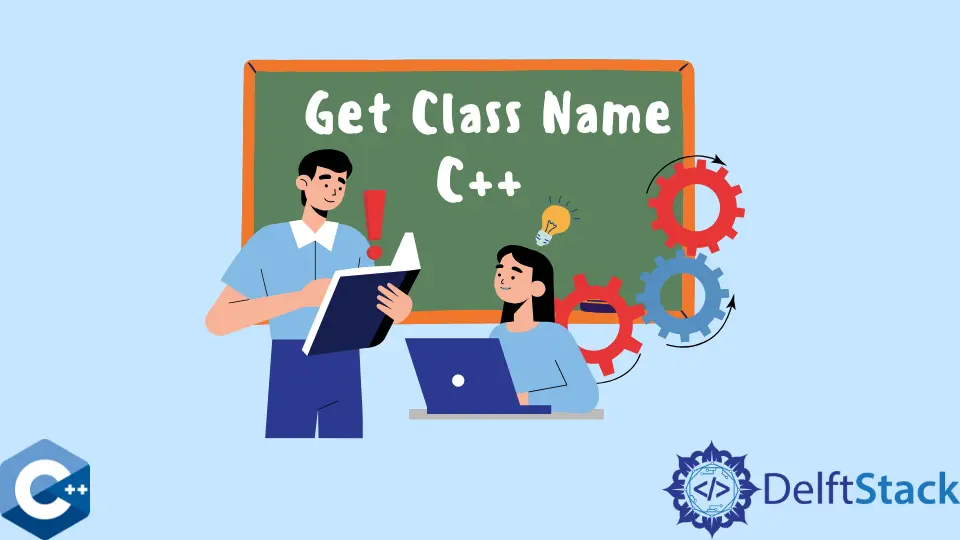
In the world of C++, knowing how to retrieve a class name can be quite beneficial, especially for debugging, logging, or even reflection purposes.
This article aims to guide you through the various methods to get the class name using the C++ programming language. Whether you’re a novice or an experienced developer, understanding how to access class names can enhance your coding efficiency and improve your overall programming skills. So, let’s dive into the methods that will help you achieve this in C++!
Using typeid Operator
One of the most straightforward ways to get the class name in C++ is by using the typeid
operator. This operator provides runtime type information about an object. When you apply it to an object, it returns a type_info
object that contains the class name as a string.
Here is a simple example to illustrate this method:
#include <iostream>
#include <typeinfo>
class MyClass {
};
int main() {
MyClass obj;
std::cout << "Class name: " << typeid(obj).name() << std::endl;
return 0;
}
Output:
Class name: 7MyClass
In this example, we define a class named MyClass
and create an object of that class. By using typeid(obj).name()
, we retrieve the name of the class. The output may not be in a human-readable format depending on the compiler, as it often includes mangled names. Nonetheless, this method effectively provides the class name at runtime.
Using Class Name in a Function
Another effective technique is to create a member function within the class that returns the class name. This approach is particularly useful for logging or debugging purposes, as it encapsulates the logic within the class itself.
Here’s how you can implement this:
#include <iostream>
#include <string>
class MyClass {
public:
std::string getClassName() {
return "MyClass";
}
};
int main() {
MyClass obj;
std::cout << "Class name: " << obj.getClassName() << std::endl;
return 0;
}
Output:
Class name: MyClass
In this code, we define a member function getClassName()
that simply returns the name of the class as a string. When we call this function on the object obj
, it provides a clear and readable output. This method is particularly beneficial when you want to ensure that the class name remains consistent and easy to manage.
Using Macros for Class Name
If you want to automate the process of getting the class name, you can use preprocessor macros. This method allows you to define a macro that retrieves the class name without the need for additional member functions or typeid.
Here’s an example of how to do this:
#include <iostream>
#define CLASS_NAME(name) #name
class MyClass {
public:
void printClassName() {
std::cout << "Class name: " << CLASS_NAME(MyClass) << std::endl;
}
};
int main() {
MyClass obj;
obj.printClassName();
return 0;
}
Output:
Class name: MyClass
In this example, we define a macro CLASS_NAME
that converts the class name into a string. Inside the class MyClass
, we use this macro in the printClassName()
method. This technique is particularly useful when you want to avoid hardcoding class names and keep your code clean and maintainable.
Conclusion
In summary, retrieving class names in C++ can be accomplished using various methods, including the typeid
operator, member functions, and preprocessor macros. Each approach has its advantages, depending on your specific use case and coding style. By incorporating these techniques into your programming toolkit, you can enhance your debugging capabilities and improve code readability. Understanding how to get class names will undoubtedly make you a more proficient C++ developer.
FAQ
-
How can I get the class name of an object in C++?
You can use thetypeid
operator or define a member function that returns the class name as a string. -
Is the output of typeid always human-readable?
No, the output oftypeid
can sometimes be a mangled name, depending on the compiler. -
Can I use macros to get class names in C++?
Yes, you can define a macro that converts the class name into a string, which can be useful for automation. -
What is the benefit of using member functions to get class names?
Member functions provide a clear and consistent way to retrieve class names, improving code readability and maintainability. -
Are there any performance implications of using typeid?
Generally, usingtypeid
has minimal performance overhead, but it’s best used judiciously in performance-critical applications.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn