Wrapper Class in C++
- Understanding Wrapper Classes in C++
- Why Are Wrapper Classes Used in C++
- Create a Wrapper Class in C++
- Conclusion
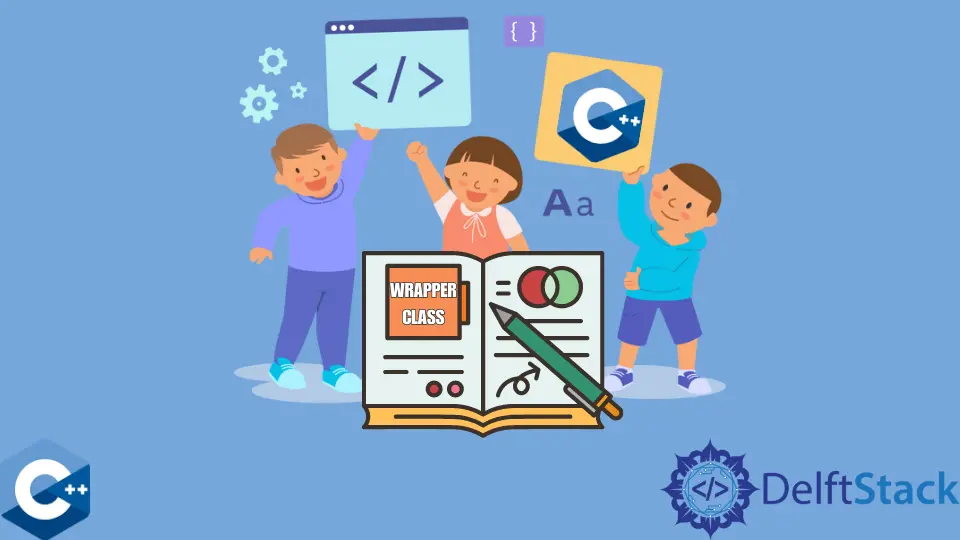
In C++, a “wrapper class” is a term often used to describe a class that “wraps” or encapsulates a primitive data type, providing additional functionality, abstraction, or customization options. Unlike languages like Java or C#, C++ does not have native wrapper classes in the same sense. However, developers can create their wrapper classes to simulate similar behavior and advantages.
Understanding Wrapper Classes in C++
A wrapper class is a special type of class that wraps around a managed resource. Let us see an example to understand it better.
Example code:
class int_wrapper {
public:
int_wrapper(int value = 0) : myPtr(new int(value)) {}
~int_wrapper() { delete myPtr; }
private:
int* myPtr;
};
Now the above class wraps a pointer to an int
. All the resources should be wrapped in some manner for cleanliness (a destructor does that automatically) and correctness.
This pattern is often called Resource-Acquisition Is Initialization (RAII). So, the idea is to bind a destructor for cleanliness.
For example, we wrap many functionalities of C language to get an object-oriented interface instead of a procedural one.
Suppose we have a function fun()
:
void fun() { cout << "hero"; }
A wrapper class will look like below:
class temp {
void fun() { cout << "hero"; }
};
Purpose:
- Abstraction: Wrapper classes abstract the complexities of dealing directly with primitive data types by providing a more object-oriented interface.
- Enhanced Functionality: They can include additional methods, behaviors, or attributes that aren’t available with primitive types.
- Customization: Developers can implement custom logic or restrictions on how the data is manipulated or accessed.
Example Scenario:
Imagine needing a more sophisticated integer type with additional functionalities like checking for prime numbers or handling conversions specific to your application. A wrapper class could encapsulate an integer and provide these extra functionalities.
Why Are Wrapper Classes Used in C++
Let’s see an example where wrapper classes are needed:
Assume a company uses different types of cameras. Now, every camera manufacturer will have different functions to make the camera work.
Now, if a developer wants to build an application or add extra functions to these cameras, one way would be to build and write separate codes for each camera type or else write one wrapper class with extra functions that will wrap around the existing code cameras.
Create a Wrapper Class in C++
Structure:
A basic wrapper class typically consists of:
- Private Data: Encapsulated primitive data type.
- Public Methods: Accessors, mutators, and additional functionalities.
Example Code:
class IntWrapper {
private:
int value;
public:
// Constructor
IntWrapper(int val) : value(val) {}
// Accessor
int getValue() const { return value; }
// Mutator
void setValue(int val) { value = val; }
// Additional functionality
bool isPrime() const {
if (value <= 1) return false;
for (int i = 2; i * i <= value; ++i) {
if (value % i == 0) return false;
}
return true;
}
};
The code defines an IntWrapper
class, acting as a wrapper around an integer primitive type. It encapsulates an integer value within a private member variable value
.
The class provides a constructor that initializes this value upon object creation. Public methods are included for accessing (getValue()
) and modifying (setValue()
) the encapsulated integer.
Additionally, the class offers an isPrime()
method to determine whether the stored integer is a prime number. This method employs a basic prime checking algorithm, returning true
if the value is greater than 1 and has no divisors other than 1 and itself, otherwise returning false
.
Overall, this IntWrapper
class serves to encapsulate an integer while offering functionalities to manipulate and query properties of the encapsulated value.
Usage (main method):
int main() {
IntWrapper myInt(10);
// Using the wrapper class
std::cout << "Value: " << myInt.getValue() << std::endl;
std::cout << "Is Prime? " << (myInt.isPrime() ? "Yes" : "No") << std::endl;
myInt.setValue(7);
std::cout << "New Value: " << myInt.getValue() << std::endl;
std::cout << "Is Prime? " << (myInt.isPrime() ? "Yes" : "No") << std::endl;
return 0;
}
Output:
Value: 10
Is Prime? No
New Value: 7
Is Prime? Yes
This code snippet demonstrates the usage of a custom wrapper class IntWrapper
in C++. It starts by creating an instance myInt
of the IntWrapper
class initialized with an integer value of 10.
The code then utilizes the functionalities provided by the wrapper class, first displaying the stored value and determining whether it is a prime number using the getValue()
and isPrime()
methods, respectively. After modifying the stored value to 7 using the setValue()
method, the code displays the updated value and checks again if it is a prime number.
This example showcases how the wrapper class encapsulates an integer value and offers additional functionalities, allowing manipulation and assessment of the encapsulated data in a controlled and customized manner.
Advantages of Wrapper Classes in C++:
- Encapsulation: Data hiding ensures the internal representation is hidden from the outside world, promoting better code maintenance and reusability.
- Customization: Additional functionalities can be tailored to specific needs, improving code readability and modularity.
- Abstraction: Abstracting the primitive data types helps in managing complexity and potential errors.
Limitations and Considerations:
- Performance Overhead: Wrapper classes may introduce overhead due to function calls and additional logic compared to direct use of primitive types.
- Design Complexity: Introducing wrapper classes for all primitive types can lead to complex code if not carefully managed.
- Applicability: Using wrapper classes is advisable when specific functionality or abstraction is necessary. For simple use cases, direct primitive types may be more efficient.
Conclusion
While C++ doesn’t have built-in wrapper classes like some other languages, developers can create their wrapper classes to encapsulate primitive types, offering enhanced functionality, abstraction, and customization. Wrapper classes can simplify code, improve maintainability, and provide additional features tailored to specific application requirements.
However, they should be used judiciously, considering trade-offs in performance and complexity. Ultimately, wrapper classes empower C++ programmers to abstract and manipulate primitive data types in a more object-oriented manner, enhancing the language’s flexibility and usability in various applications.