C++ 中的多重继承
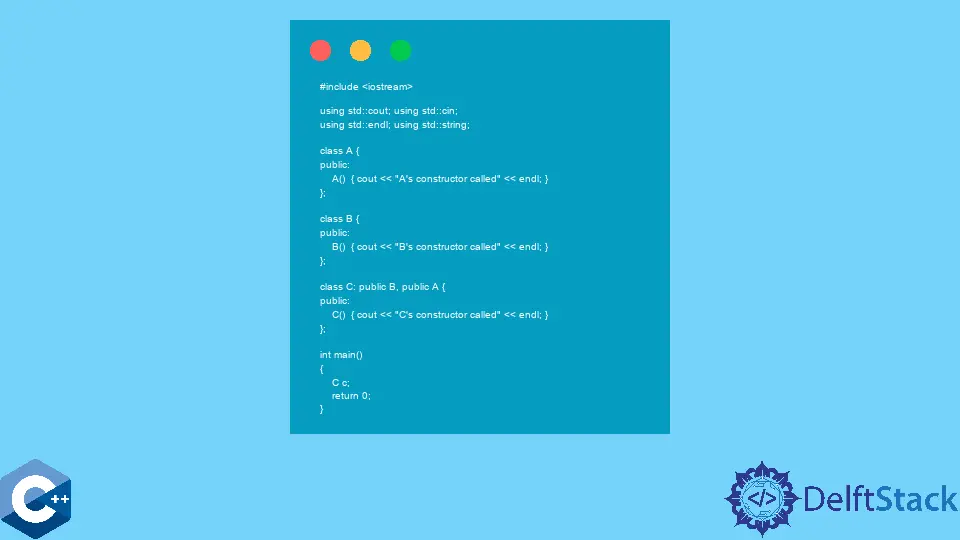
本文将演示有关如何在 C++ 中使用多重继承的多种方法。
使用多重继承将两个给定类的多个属性应用于另一个类
C++ 中的类可以具有多个继承,这提供了从多个直接基类派生一个类的可能性。
这意味着如果没有仔细实现这些类,则可能会出现特殊的异常行为。例如,考虑以下代码段代码,其中 C
类是从 B
和 A
类派生的。它们都有默认的构造函数,该构造函数输出特殊的字符串。虽然,当我们在 mainc
函数中声明 C
类型的对象时,三个构造函数会打印输出。请注意,构造函数以与继承相同的顺序被调用。另一方面,析构函数的调用顺序相反。
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class A {
public:
A() { cout << "A's constructor called" << endl; }
};
class B {
public:
B() { cout << "B's constructor called" << endl; }
};
class C : public B, public A {
public:
C() { cout << "C's constructor called" << endl; }
};
int main() {
C c;
return 0;
}
输出:
Bs constructor called
As constructor called
Cs constructor called
使用 virtual
关键字修复基类的多个副本
请注意,以下程序输出 Planet
类构造函数的两个实例,分别是 Mars
和 Earth
构造函数,最后是 Rock
类构造函数。同样,当对象超出范围时,Planet
类的析构函数将被调用两次。请注意,可以通过在 Mars
和 Earth
类中添加 virtual
关键字来解决此问题。当一个类具有多个基类时,有可能派生
类将从其两个或多个基类继承具有相同名称的成员。
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class Planet {
public:
Planet(int x) { cout << "Planet::Planet(int ) called" << endl; }
};
class Mars : public Planet {
public:
Mars(int x) : Planet(x) { cout << "Mars::Mars(int ) called" << endl; }
};
class Earth : public Planet {
public:
Earth(int x) : Planet(x) { cout << "Earth::Earth(int ) called" << endl; }
};
class Rock : public Mars, public Earth {
public:
Rock(int x) : Earth(x), Mars(x) { cout << "Rock::Rock(int ) called" << endl; }
};
int main() { Rock tmp(30); }
输出:
Planet::Planet(int ) called
Mars::Mars(int ) called
Planet::Planet(int ) called
Earth::Earth(int ) called
Rock::Rock(int ) called
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu