How to Implement Inorder Traversal for Binary Search Tree in C++
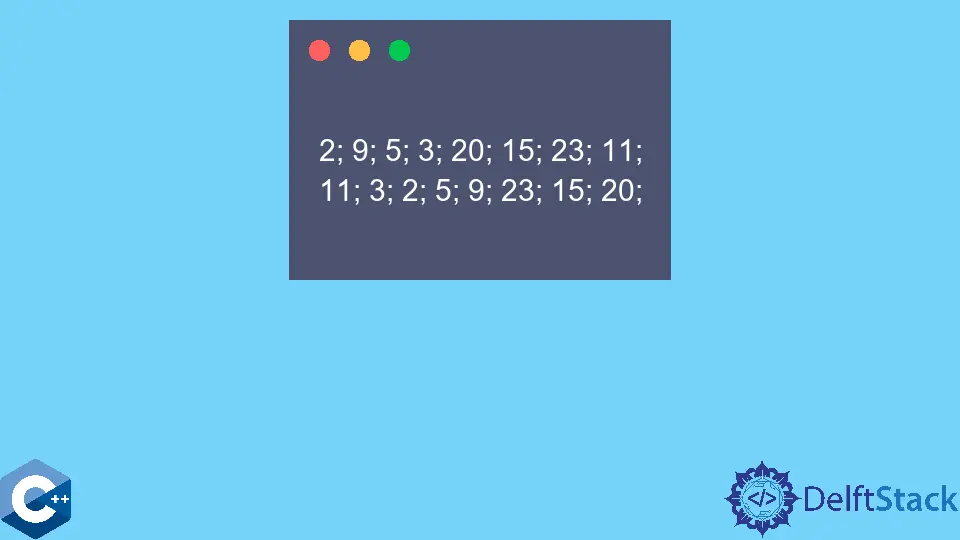
Inorder traversal is a fundamental technique for navigating through binary search trees (BSTs). It allows you to visit nodes in a specific order: left child, root, and then right child. This method is essential for various applications, such as retrieving data in a sorted manner.
If you’re looking to implement inorder traversal in C++, this article will guide you through the process with clear explanations and code examples. Whether you’re a beginner or an experienced programmer, you’ll find valuable insights here. Let’s dive into how to implement inorder traversal for a binary search tree in C++.
Understanding Binary Search Trees
Before we jump into the implementation, it’s important to understand what a binary search tree is. A binary search tree is a data structure that maintains sorted data in a hierarchical manner. Each node contains a key, and every node’s left subtree contains keys less than the node’s key, while the right subtree contains keys greater than the node’s key. This property allows for efficient searching, insertion, and deletion operations.
The inorder traversal of a binary search tree will yield the keys in ascending order. This characteristic is what makes it particularly useful for tasks that require sorted data.
Implementing Inorder Traversal in C++
Now that we have a basic understanding of binary search trees, let’s look at how to implement inorder traversal in C++. We’ll explore both recursive and iterative methods.
Recursive Method
The recursive approach is often the simplest way to implement inorder traversal. Here’s how you can do it:
#include <iostream>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
void inorderTraversal(Node* root) {
if (root == nullptr) return;
inorderTraversal(root->left);
cout << root->data << " ";
inorderTraversal(root->right);
}
Node* newNode(int data) {
Node* node = new Node();
node->data = data;
node->left = node->right = nullptr;
return node;
}
int main() {
Node* root = newNode(4);
root->left = newNode(2);
root->right = newNode(6);
root->left->left = newNode(1);
root->left->right = newNode(3);
root->right->left = newNode(5);
root->right->right = newNode(7);
cout << "Inorder Traversal: ";
inorderTraversal(root);
return 0;
}
Output:
Inorder Traversal: 1 2 3 4 5 6 7
The recursive function inorderTraversal
takes a pointer to the root node as its parameter. If the root is nullptr
, the function simply returns. Otherwise, it first recursively traverses the left subtree, prints the current node’s data, and then recursively traverses the right subtree. This method is elegant and easy to understand, making it a popular choice for implementing inorder traversal.
Iterative Method
While the recursive method is straightforward, the iterative approach can be more efficient in terms of stack space. Here’s how to implement it:
#include <iostream>
#include <stack>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
void inorderTraversal(Node* root) {
stack<Node*> s;
Node* current = root;
while (current != nullptr || !s.empty()) {
while (current != nullptr) {
s.push(current);
current = current->left;
}
current = s.top();
s.pop();
cout << current->data << " ";
current = current->right;
}
}
Node* newNode(int data) {
Node* node = new Node();
node->data = data;
node->left = node->right = nullptr;
return node;
}
int main() {
Node* root = newNode(4);
root->left = newNode(2);
root->right = newNode(6);
root->left->left = newNode(1);
root->left->right = newNode(3);
root->right->left = newNode(5);
root->right->right = newNode(7);
cout << "Inorder Traversal: ";
inorderTraversal(root);
return 0;
}
Output:
Inorder Traversal: 1 2 3 4 5 6 7
In this iterative method, we utilize a stack to keep track of nodes. We start with the current node set to the root. While there are still nodes to process (either the current node is not nullptr
or the stack is not empty), we push the current node onto the stack and move to its left child. When we can no longer go left, we pop a node from the stack, print its data, and then move to its right child. This method avoids the potential overhead of recursive calls, making it suitable for larger trees.
Conclusion
Inorder traversal is a powerful technique that allows you to navigate through binary search trees efficiently. Whether you choose the recursive or iterative method, both approaches have their advantages. The recursive method is clean and easy to implement, while the iterative method is more space-efficient. By understanding these methods, you can enhance your skills in manipulating binary search trees in C++. Experiment with both techniques to see which one fits your programming style best.
FAQ
-
What is inorder traversal?
Inorder traversal is a method of visiting nodes in a binary search tree in the order of left child, root, and then right child. -
Why is inorder traversal important?
It allows you to retrieve data from a binary search tree in sorted order, which is useful for various applications. -
Can I use inorder traversal on other types of trees?
Yes, inorder traversal can be applied to any binary tree, but it is particularly meaningful for binary search trees. -
What are the advantages of the iterative method?
The iterative method uses less stack space compared to the recursive method, making it more efficient for large trees. -
Are there any other traversal methods for binary trees?
Yes, besides inorder traversal, there are also preorder and postorder traversals, each with its own use cases.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook