Circular Doubly Linked List in C++
- Understanding Circular Doubly Linked Lists
- Implementation of Circular Doubly Linked List in C++
- Adding More Functionality
- Conclusion
- FAQ
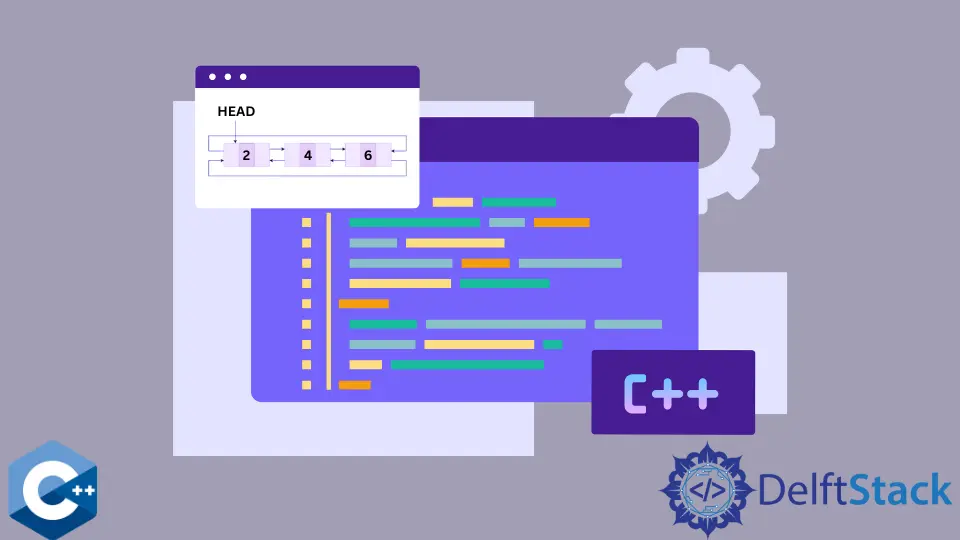
Circular doubly linked lists are a fascinating data structure that allows for efficient traversal and manipulation of data. Unlike traditional linked lists, where the last node points to null, a circular doubly linked list connects the last node back to the first node, creating a circular structure. This unique feature enables seamless navigation in both directions, making it ideal for applications such as round-robin scheduling, music playlists, and more.
In this article, we will explore how to implement a circular doubly linked list in C++. We will walk through the core concepts, provide clear code examples, and discuss the advantages of using this data structure. So, let’s dive in and unravel the world of circular doubly linked lists!
Understanding Circular Doubly Linked Lists
Before we jump into the implementation, it’s essential to understand the structure of a circular doubly linked list. Each node in this list contains three components: a pointer to the previous node, a pointer to the next node, and the data itself. The circular nature means that the last node’s next pointer points back to the first node, and the first node’s previous pointer points back to the last node. This creates a loop, allowing for continuous traversal.
Key Features:
Bidirectional Traversal
: You can traverse the list in both forward and backward directions.Circular Structure
: The last node points back to the first node, making it easy to loop through the list.Dynamic Size
: Nodes can be added or removed without the need for resizing, which is a significant advantage over arrays.
Implementation of Circular Doubly Linked List in C++
Let’s go through the implementation of a circular doubly linked list in C++. We will create a class to represent the list and define methods to insert and delete nodes.
Code Example
#include <iostream>
class Node {
public:
int data;
Node* next;
Node* prev;
Node(int val) : data(val), next(nullptr), prev(nullptr) {}
};
class CircularDoublyLinkedList {
public:
Node* head;
CircularDoublyLinkedList() : head(nullptr) {}
void insert(int val) {
Node* newNode = new Node(val);
if (!head) {
head = newNode;
head->next = head;
head->prev = head;
} else {
Node* tail = head->prev;
tail->next = newNode;
newNode->prev = tail;
newNode->next = head;
head->prev = newNode;
}
}
void display() {
if (!head) return;
Node* temp = head;
do {
std::cout << temp->data << " ";
temp = temp->next;
} while (temp != head);
std::cout << std::endl;
}
};
In this code, we first define a Node
class that holds the data and pointers to the next and previous nodes. The CircularDoublyLinkedList
class manages the list. The insert
method is responsible for adding new nodes. If the list is empty, it initializes the head node. Otherwise, it updates the pointers accordingly to maintain the circular structure.
To display the list, we use the display
method, which traverses the list starting from the head and prints each node’s data until it loops back to the head.
Output:
Adding More Functionality
Now that we have a basic implementation, let’s add more methods to enhance our circular doubly linked list. We will implement methods to delete a node and search for a value in the list.
Code Example
void deleteNode(int val) {
if (!head) return;
Node* current = head;
do {
if (current->data == val) {
if (current == head && current->next == head) {
delete current;
head = nullptr;
return;
} else {
current->prev->next = current->next;
current->next->prev = current->prev;
if (current == head) head = current->next;
delete current;
return;
}
}
current = current->next;
} while (current != head);
}
bool search(int val) {
if (!head) return false;
Node* current = head;
do {
if (current->data == val) return true;
current = current->next;
} while (current != head);
return false;
}
The deleteNode
function searches for a node with the specified value. If found, it updates the pointers of the adjacent nodes to bypass the node being deleted. If the node to be deleted is the head, it updates the head pointer accordingly.
The search
function checks if a value exists in the list, returning true if found and false otherwise. This functionality is crucial for many applications, allowing for efficient data retrieval.
Output:
Conclusion
In conclusion, a circular doubly linked list is a versatile and efficient data structure that offers numerous advantages over traditional linked lists. Its ability to traverse in both directions and maintain a circular structure makes it ideal for various applications. In this article, we explored how to implement a circular doubly linked list in C++, covering essential methods for insertion, deletion, and searching. Understanding this data structure can significantly enhance your programming skills and open up new possibilities for your projects. So why not give it a try in your next coding endeavor?
FAQ
-
What is a circular doubly linked list?
A circular doubly linked list is a data structure where each node contains pointers to both the next and previous nodes, and the last node points back to the first node, forming a circle. -
What are the advantages of using a circular doubly linked list?
The advantages include bidirectional traversal, dynamic size, and efficient insertion and deletion operations. -
How do you insert a node into a circular doubly linked list?
To insert a node, create a new node, adjust the pointers of the surrounding nodes, and update the head if necessary. -
Can you delete a node from a circular doubly linked list?
Yes, you can delete a node by updating the pointers of the adjacent nodes to bypass the node being removed. -
How do you search for a value in a circular doubly linked list?
You can search by traversing the list from the head and checking each node’s data until you find the value or loop back to the head.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook