Binary Search Tree Insertion in C++
- Understanding Binary Search Trees
- Insertion Method for Binary Search Tree
- Recursive vs. Iterative Insertion
- Conclusion
- FAQ
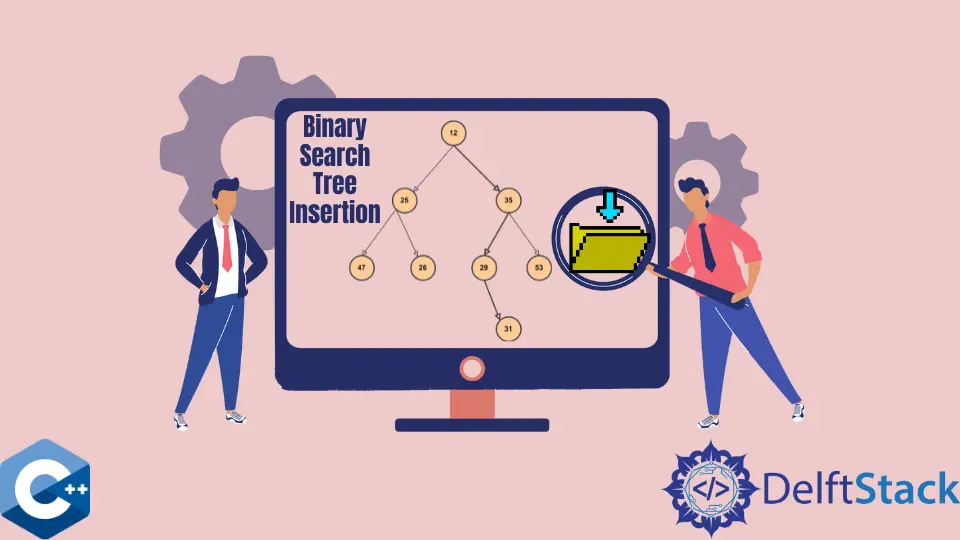
Binary Search Trees (BST) are a fundamental data structure that allows for efficient searching, insertion, and deletion of data.
In this article, we will delve into how to implement the insertion function for a binary search tree in C++. Understanding this concept is essential for anyone looking to improve their programming skills or work with data structures effectively. We’ll walk through the key components of the insertion process, providing clear code examples and detailed explanations to ensure you grasp the concepts fully. By the end of this article, you will be equipped to implement your own insertion function in C++ and understand how it operates within the context of a binary search tree.
Understanding Binary Search Trees
Before we dive into the insertion method, let’s briefly discuss what a binary search tree is. A binary search tree is a tree data structure in which each node has at most two children. The left child contains values less than the parent node, while the right child contains values greater than the parent node. This property makes searching for values efficient, as you can eliminate half of the tree at each step.
When inserting a new value, the algorithm starts at the root and traverses the tree, comparing the value to be inserted with the current node’s value. If the new value is less, it moves to the left child; if it’s greater, it moves to the right child. This process continues until it finds an appropriate null position to insert the new node.
Insertion Method for Binary Search Tree
Now, let’s look at how to implement the insertion function in C++. The insertion function will take a pointer to the root of the tree and the value to be inserted as parameters. If the tree is empty, the new value will become the root. Otherwise, we will recursively find the correct position for the new value.
Here’s a simple implementation:
#include <iostream>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
Node* insert(Node* root, int value) {
if (root == nullptr) {
Node* newNode = new Node();
newNode->data = value;
newNode->left = nullptr;
newNode->right = nullptr;
return newNode;
}
if (value < root->data) {
root->left = insert(root->left, value);
} else {
root->right = insert(root->right, value);
}
return root;
}
void inorder(Node* root) {
if (root != nullptr) {
inorder(root->left);
cout << root->data << " ";
inorder(root->right);
}
}
int main() {
Node* root = nullptr;
root = insert(root, 50);
insert(root, 30);
insert(root, 70);
insert(root, 20);
insert(root, 40);
insert(root, 60);
insert(root, 80);
cout << "Inorder traversal: ";
inorder(root);
return 0;
}
Output:
Inorder traversal: 20 30 40 50 60 70 80
In this code, we define a structure for the nodes of the tree. The insert
function checks if the root is null, indicating that we have found a position for the new node. If the root is not null, it compares the value to be inserted with the current node’s data. Depending on the comparison result, it either moves left or right to find the appropriate position. The inorder
function is used to display the values in sorted order, confirming that the insertion was successful.
Recursive vs. Iterative Insertion
While the recursive method is elegant, some may prefer an iterative approach for inserting nodes into a binary search tree. The iterative method uses a loop instead of recursion, which can be more efficient in terms of memory usage. Here’s how you can implement the iterative insertion method:
#include <iostream>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
Node* insert(Node* root, int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->left = nullptr;
newNode->right = nullptr;
if (root == nullptr) {
return newNode;
}
Node* current = root;
Node* parent = nullptr;
while (true) {
parent = current;
if (value < current->data) {
current = current->left;
if (current == nullptr) {
parent->left = newNode;
return root;
}
} else {
current = current->right;
if (current == nullptr) {
parent->right = newNode;
return root;
}
}
}
}
void inorder(Node* root) {
if (root != nullptr) {
inorder(root->left);
cout << root->data << " ";
inorder(root->right);
}
}
int main() {
Node* root = nullptr;
root = insert(root, 50);
insert(root, 30);
insert(root, 70);
insert(root, 20);
insert(root, 40);
insert(root, 60);
insert(root, 80);
cout << "Inorder traversal: ";
inorder(root);
return 0;
}
Output:
Inorder traversal: 20 30 40 50 60 70 80
In this iterative version of the insertion function, we create a new node and check if the tree is empty. If it is not, we use a while
loop to find the correct parent node where the new node should be attached. The loop continues until it finds a null position, at which point it assigns the new node to the left or right child of the identified parent node.
Conclusion
Inserting nodes into a binary search tree is a fundamental operation that lays the groundwork for understanding more complex data structures and algorithms. Whether you choose a recursive or iterative approach, both methods offer efficient ways to maintain the properties of the binary search tree. With these examples and explanations, you now have the tools to implement insertion functionality in your own C++ projects. Mastering binary search trees will enhance your programming skills and prepare you for more advanced topics in data structures and algorithms.
FAQ
-
What is a binary search tree?
A binary search tree is a data structure where each node has at most two children, with the left child containing values less than the parent and the right child containing values greater than the parent. -
How does the insertion process work in a binary search tree?
The insertion process involves comparing the value to be inserted with the current node’s value and recursively or iteratively finding the appropriate position in the tree. -
What are the advantages of using a binary search tree?
Binary search trees allow for efficient searching, insertion, and deletion operations, making them suitable for various applications, including databases and memory management. -
Can I use a binary search tree to store duplicate values?
Typically, binary search trees do not allow duplicate values. However, variations such as multi-way trees can accommodate duplicates. -
How do I traverse a binary search tree?
Common traversal methods include inorder, preorder, and postorder traversal, each providing different ways to visit all the nodes in the tree.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook