Binary Search Tree Destructor in C++
- C++ Binary Search Tree
-
Use the
delete
Keyword to Create a Destructor for Binary Search Tree in C++ - Use Recursive Post-order Traversal to Create a Binary Search Tree Destructor in C++
- Use Iterative Post-order Traversal to Create a Binary Search Tree Destructor in C++
- Use Level-order Traversal to Create a Binary Search Tree Destructor in C++
- Conclusion
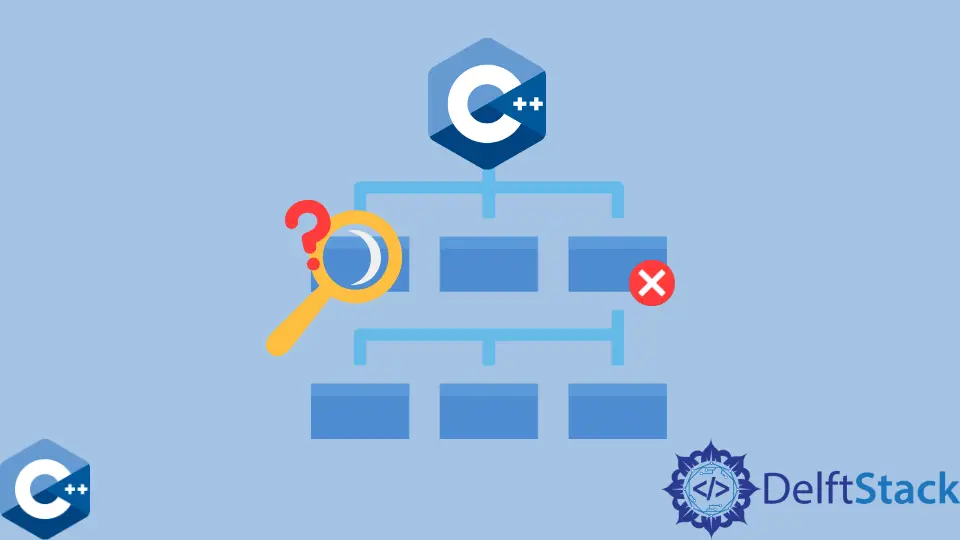
An integral aspect of managing data structures in C++ involves proper memory management, especially when dealing with dynamic data structures like a Binary Search Tree (BST). Creating an effective destructor for a BST is paramount to prevent memory leaks and ensure efficient memory utilization.
A destructor’s role in a BST is to systematically deallocate memory by freeing the dynamically allocated nodes in the tree.
In this article, we’ll explore multiple methodologies to craft a destructor for a BST in C++. Each method will be meticulously explained, accompanied by illustrative code examples, demonstrating various traversal techniques and memory deallocation strategies.
By delving into recursive and iterative traversal methods such as post-order traversal and level-order traversal, we aim to provide a thorough understanding of how to implement a destructor tailored for different BST scenarios in C++. These approaches ensure proper memory cleanup and prevent memory leaks when destroying a BST in C++.
C++ Binary Search Tree
A binary search tree (BST) is a data structure that stores sorted data that can be searched. It is used in data centers and software.
It consists of nodes, and each node has two children. When a binary tree is built, the first value represents the root node, and the next value will be placed to its right if it is larger than the root node; otherwise, it will be placed to its left.
When the next value comes, we have to compare it with the root node first, and then we will compare it with other children’s if there are any.
Each node of the binary search tree consists of a key and value. The binary search tree is easy to search because it is already sorted.
Use the delete
Keyword to Create a Destructor for Binary Search Tree in C++
To build a binary search tree in C++, we can use the int
data type for the value and two pointer variables for the left and right nodes along with the this
keyword, which is used to refer the current variables to the instance of the class. To create the destructor that deletes the entire binary tree, we can use the delete
keyword to deallocate the variables’ memory.
We need to deallocate the memory of the left and right nodes to delete the binary search tree. For example, let’s create a public class of binary search tree, which will contain two methods: one to build the tree and one to delete it.
#include <iostream>
using namespace std;
class BTreeNode {
public:
int Treedata;
BTreeNode* leftNode;
BTreeNode* rightNode;
BTreeNode(int Treedata) {
this->Treedata = Treedata;
this->leftNode = NULL;
this->rightNode = NULL;
}
~BTreeNode() {
delete leftNode;
delete rightNode;
cout << "Deleting " << this->Treedata << endl;
}
};
int main() {
BTreeNode* root = new BTreeNode(1);
BTreeNode* node1 = new BTreeNode(2);
BTreeNode* node2 = new BTreeNode(3);
root->leftNode = node1;
root->rightNode = node2;
delete root;
return 0;
}
Output:
Deleting 2
Deleting 3
Deleting 1
In the above code, we created a tree with a root and two nodes and deleted it using the delete
keyword. We also used the cout()
function to display the values of the nodes that are being deleted one by one.
In the above output, we can see that node2
is deleted first because it is the left node, and we deleted the left node first inside the destructor of the binary search tree.
Use Recursive Post-order Traversal to Create a Binary Search Tree Destructor in C++
Post-order traversal follows the sequence: left, right, root. This traversal strategy ensures that child nodes are visited and deleted before their parents.
Implementing this approach recursively enables a straightforward way to create a destructor for a BST.
Let’s begin by defining the structure for a BST node and the BST class in C++:
class TreeNode {
public:
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int value) : val(value), left(nullptr), right(nullptr) {}
~TreeNode() {
delete left;
delete right;
}
};
class BinarySearchTree {
public:
TreeNode* root;
BinarySearchTree() : root(nullptr) {}
// Other BST methods (insert, search, etc.)...
~BinarySearchTree() { deleteTree(root); }
private:
void deleteTree(TreeNode* root) {
if (root == nullptr) return;
deleteTree(root->left);
deleteTree(root->right);
delete root;
}
};
The TreeNode
class represents each node in the BST. Its destructor is responsible for recursively deleting the left and right child nodes, ensuring the deletion of the entire subtree rooted at that node.
The BinarySearchTree
class contains the root node of the BST. Its destructor triggers the deletion of the entire BST by calling the deleteTree
function, which initiates the recursive post-order traversal.
Let’s implement the recursive post-order traversal approach within the deleteTree
function to create the BST destructor:
class BinarySearchTree {
// ... (previously defined code)
private:
void deleteTree(TreeNode* root) {
if (root == nullptr) return;
deleteTree(root->left);
deleteTree(root->right);
delete root;
}
public:
// ... (other BST methods)
~BinarySearchTree() { deleteTree(root); }
};
The deleteTree
function initiates the post-order traversal from the root node, deleting child nodes before their parents. This recursive process ensures proper memory deallocation by systematically deleting all nodes in the BST.
Recursive post-order traversal simplifies the destructor creation process, providing an intuitive way to release memory in a BST. It ensures memory cleanup by deleting nodes in a bottom-up manner, preventing memory leaks.
However, for exceptionally deep or unbalanced trees, recursive methods might consume more call stack space, potentially leading to stack overflow. In such scenarios, iterative approaches or tree-balancing techniques can be considered for efficient memory management.
Use Iterative Post-order Traversal to Create a Binary Search Tree Destructor in C++
Post-order traversal visits nodes in the order of left, right, and root. Implementing this strategy iteratively allows for sequential node deletion, ensuring that child nodes are removed before their parents.
This alternative approach uses an iterative post-order traversal to delete nodes without recursion for efficient memory cleanup in a BST.
Let’s start by defining the structure for a BST node and the BST class in C++:
class TreeNode {
public:
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int value) : val(value), left(nullptr), right(nullptr) {}
};
class BinarySearchTree {
public:
TreeNode* root;
BinarySearchTree() : root(nullptr) {}
// Other BST methods (insert, search, etc.)...
~BinarySearchTree() { destroyTree(root); }
private:
void destroyTree(TreeNode* root) {
if (root == nullptr) return;
std::stack<TreeNode*> stack;
TreeNode* prev = nullptr;
while (root || !stack.empty()) {
while (root) {
stack.push(root);
root = root->left;
}
root = stack.top();
if (root->right == nullptr || root->right == prev) {
delete root;
stack.pop();
prev = root;
root = nullptr;
} else {
root = root->right;
}
}
}
};
The TreeNode
class represents each node in the BST. The BinarySearchTree
class contains the root node of the BST.
Let’s implement the iterative post-order traversal approach within the destroyTree
function to create the BST destructor:
class BinarySearchTree {
// ... (previously defined code)
private:
void destroyTree(TreeNode* root) {
if (root == nullptr) return;
std::stack<TreeNode*> stack;
TreeNode* prev = nullptr;
while (root || !stack.empty()) {
while (root) {
stack.push(root);
root = root->left;
}
root = stack.top();
if (root->right == nullptr || root->right == prev) {
delete root;
stack.pop();
prev = root;
root = nullptr;
} else {
root = root->right;
}
}
}
public:
// ... (other BST methods)
~BinarySearchTree() { destroyTree(root); }
};
The destroyTree
function performs iterative post-order traversal, emulating the post-order sequence of left, right, root. It uses a stack-based iterative approach to delete nodes in a bottom-up manner.
Iterative post-order traversal simplifies the destructor creation process by efficiently deallocating memory without recursion. This method ensures proper memory cleanup by deleting nodes in a bottom-up manner, preventing memory leaks.
However, compared to recursive approaches, iterative methods might require more code complexity due to manual stack management. In large or complex BSTs, this iterative approach might offer better scalability and reduced call stack usage compared to recursive methods.
Use Level-order Traversal to Create a Binary Search Tree Destructor in C++
Level-order traversal, also known as Breadth-First Search (BFS), explores nodes level by level, starting from the root. This traversal strategy ensures that nodes are visited in a breadth-first manner, facilitating a sequential approach to node deletion.
Utilizing this strategy provides an alternative to recursive or iterative methods for efficient memory cleanup in a BST.
Let’s begin by defining the structure for a BST node and the BST class in C++:
class TreeNode {
public:
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int value) : val(value), left(nullptr), right(nullptr) {}
};
class BinarySearchTree {
public:
TreeNode* root;
BinarySearchTree() : root(nullptr) {}
// Other BST methods (insert, search, etc.)...
~BinarySearchTree() { destroyTree(root); }
private:
void destroyTree(TreeNode* root) {
if (root == nullptr) return;
std::queue<TreeNode*> queue;
queue.push(root);
while (!queue.empty()) {
TreeNode* current = queue.front();
queue.pop();
if (current->left) queue.push(current->left);
if (current->right) queue.push(current->right);
delete current;
}
}
};
The TreeNode
class represents each node in the BST. The BinarySearchTree
class contains the root node of the BST.
Let’s implement the level-order traversal approach within the destroyTree
function to create the BST destructor:
class BinarySearchTree {
// ... (previously defined code)
private:
void destroyTree(TreeNode* root) {
if (root == nullptr) return;
std::queue<TreeNode*> queue;
queue.push(root);
while (!queue.empty()) {
TreeNode* current = queue.front();
queue.pop();
if (current->left) queue.push(current->left);
if (current->right) queue.push(current->right);
delete current;
}
}
public:
// ... (other BST methods)
~BinarySearchTree() { destroyTree(root); }
};
The destroyTree
function initiates the level-order traversal starting from the root node, deleting nodes in a breadth-first manner. This sequential deletion ensures proper memory deallocation by systematically deleting all nodes in the BST.
Level-order traversal simplifies the destructor creation process by efficiently deallocating memory in a breadth-first manner. This method ensures proper memory cleanup by deleting nodes level by level, preventing memory leaks.
However, for extremely deep or unbalanced trees, level-order traversal might require more memory usage due to queue-based traversal, potentially impacting performance in large BSTs.
Conclusion
In exploring BST destructor creation in C++, we investigated four methods: using the delete
keyword, recursive post-order traversal, iterative post-order traversal, and level-order traversal. Each technique aims to efficiently deallocate memory in BSTs, emphasizing systematic node deletion to prevent memory leaks.
- Using
delete
Keyword: Demonstrated straightforward node deletion with explicitdelete
calls, displaying a clear deletion sequence. - Recursive Post-order Traversal: Employed a recursive bottom-up approach, deleting child nodes before their parents, ensuring thorough memory deallocation.
- Iterative Post-order Traversal: Offered an iterative alternative to recursion, deleting nodes bottom-up without using recursive calls, minimizing stack usage.
- Level-order Traversal: Implemented a breadth-first approach for node deletion, efficiently deallocating memory but possibly consuming more memory due to queue-based traversal.
Each method showcased the significance of systematic memory cleanup and varied approaches to constructing BST destructors in C++. Understanding these strategies enables efficient memory management and prevents memory-related issues in C++ programs. Choosing an appropriate method depends on BST characteristics, such as depth, balance, and memory constraints.