在 C++ 中实现二叉搜索树的中序遍历
Jinku Hu
2023年10月12日
C++
C++ Data Structure
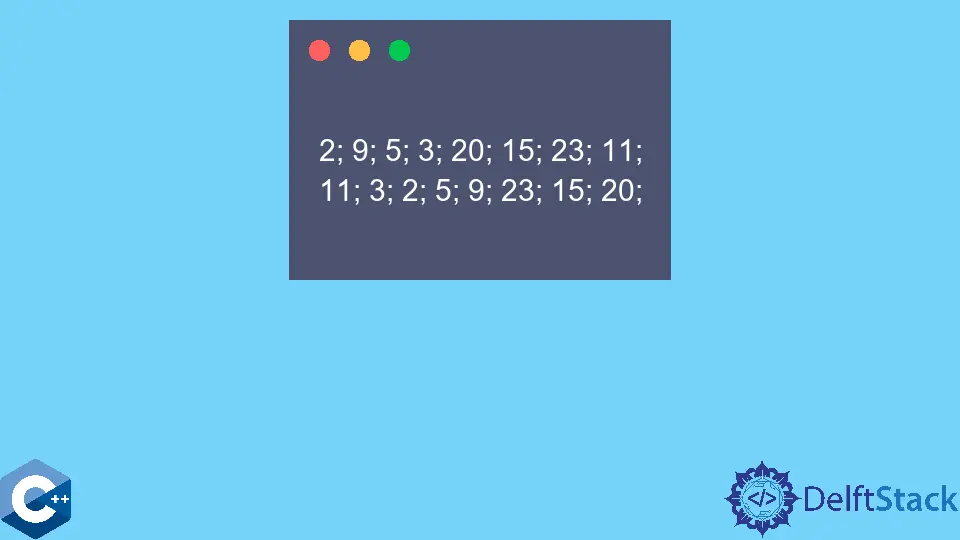
本文将讲解如何在 C++ 中实现二叉搜索树的中序遍历。
使用中序遍历打印二叉搜索树的内容
二叉搜索树的构造使得每个节点的键必须大于其左子树中的所有键,并且小于右子树中的所有键。
为了简单起见,我们在这里只考虑不平衡树,但在实际场景中,二叉搜索树的效率来自平衡性质,其中根的每个子树具有大致相同的高度。
可以使用三种不同的方法遍历二叉树:中序、前序和后序。二叉搜索树的中序遍历产生按非递减顺序排序的元素。这个版本通常从最左边的节点开始,按照先遍历左子树的顺序,然后是根节点,最后是右子树。
请注意以下代码片段输出和整数插入树时的顺序。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct TreeNode {
int key;
struct TreeNode *left{};
struct TreeNode *right{};
};
void insertNode(TreeNode *&root, const int k) {
if (root == nullptr) {
root = new TreeNode;
root->key = k;
root->left = nullptr;
root->right = nullptr;
} else {
if (k < root->key)
insertNode(root->left, k);
else
insertNode(root->right, k);
}
}
void printTreeInorder(TreeNode *n) {
if (n != nullptr) {
printTreeInorder(n->left);
cout << n->key << "; ";
printTreeInorder(n->right);
}
}
int main() {
std::vector<int> v1{11, 23, 3, 5, 9, 15, 2, 20};
TreeNode *root = nullptr;
for (const auto &item : v1) {
insertNode(root, item);
}
printTreeInorder(root);
cout << endl;
return EXIT_SUCCESS;
}
2; 3; 5; 9; 11; 15; 20; 23;
或者,我们可能需要利用前序或后序遍历来访问二叉搜索树中的节点。我们只需要在 printTree*
函数中移动 cout << n->key << "; "
行来修改遍历算法。
前序遍历从根节点开始打印,然后分别进入左右子树,而后序遍历最后访问根节点。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct TreeNode {
int key;
struct TreeNode *left{};
struct TreeNode *right{};
};
void insertNode(TreeNode *&root, const int k) {
if (root == nullptr) {
root = new TreeNode;
root->key = k;
root->left = nullptr;
root->right = nullptr;
} else {
if (k < root->key)
insertNode(root->left, k);
else
insertNode(root->right, k);
}
}
void printTreePreorder(TreeNode *n) {
if (n != nullptr) {
cout << n->key << "; ";
printTreePreorder(n->left);
printTreePreorder(n->right);
}
}
void printTreePostorder(TreeNode *n) {
if (n != nullptr) {
printTreePostorder(n->left);
printTreePostorder(n->right);
cout << n->key << "; ";
}
}
int main() {
std::vector<int> v1{11, 23, 3, 5, 9, 15, 2, 20};
TreeNode *root = nullptr;
for (const auto &item : v1) {
insertNode(root, item);
}
printTreePostorder(root);
cout << endl;
printTreePreorder(root);
cout << endl;
return EXIT_SUCCESS;
}
2; 9; 5; 3; 20; 15; 23; 11;
11; 3; 2; 5; 9; 23; 15; 20;
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu