How to Use the ignore() Function in C++
-
Understanding the
ignore()
Function - Basic Usage of ignore()
- Ignoring Characters in a Loop
- Handling Input Errors with ignore()
- Conclusion
- FAQ
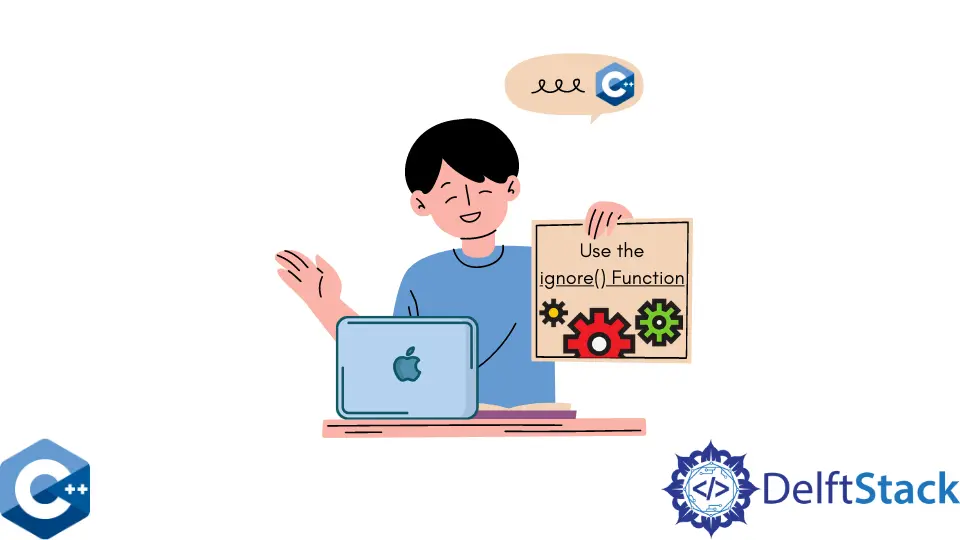
When working with input and output in C++, managing the flow of data can sometimes become tricky. One common issue arises when you need to skip unwanted characters or data from the input stream. This is where the ignore()
function comes into play. It is a powerful tool that allows you to control what gets read from the input stream, ensuring that your program behaves as expected.
In this article, we will explore how to effectively use the ignore()
function in C++, complete with examples and detailed explanations. Whether you’re a beginner or looking to sharpen your skills, understanding this function is essential for efficient input handling in your applications.
Understanding the ignore()
Function
The ignore()
function is part of the <iostream>
library in C++. It is primarily used with input streams, such as cin
, to skip characters in the input buffer. This function can be particularly useful when dealing with formatted input or when you want to discard unwanted characters after reading a certain type of data.
The syntax for the ignore()
function is as follows:
istream& ignore(streamsize n = 1, int delim = EOF);
n
: The number of characters to ignore. The default value is 1.delim
: The delimiter at which to stop ignoring characters. The default isEOF
.
Using ignore()
can help prevent errors caused by leftover characters in the input buffer, which can lead to unexpected behavior in your program.
Basic Usage of ignore()
To demonstrate the basic usage of the ignore()
function, let’s consider a simple example where we read an integer from the user but want to ignore any remaining characters in the input buffer.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
cin.ignore(1000, '\n'); // Ignore the rest of the line
cout << "You entered: " << number << endl;
return 0;
}
Output:
Enter an integer: 42
You entered: 42
In this example, we first prompt the user to enter an integer. After reading the integer using cin
, we call cin.ignore(1000, '\n')
. This command effectively ignores up to 1000 characters or until a newline character is encountered. This is useful in scenarios where the user might input additional characters, such as pressing the Enter key multiple times after entering the number.
By using ignore()
, we ensure that any leftover characters do not interfere with subsequent input operations, maintaining the integrity of our program.
Ignoring Characters in a Loop
Sometimes, you may need to use ignore()
in a loop to process multiple inputs while ensuring that unwanted characters do not disrupt the flow. Below is an example where we read multiple integers until the user decides to stop.
#include <iostream>
using namespace std;
int main() {
int number;
char choice;
do {
cout << "Enter an integer: ";
cin >> number;
cin.ignore(1000, '\n'); // Ignore the rest of the line
cout << "You entered: " << number << endl;
cout << "Do you want to enter another number? (y/n): ";
cin >> choice;
cin.ignore(1000, '\n'); // Ignore the newline character
} while (choice == 'y' || choice == 'Y');
return 0;
}
Output:
Enter an integer: 10
You entered: 10
Do you want to enter another number? (y/n): y
Enter an integer: 20
You entered: 20
Do you want to enter another number? (y/n): n
In this example, we use a do-while
loop to repeatedly prompt the user for an integer. After reading the integer, we call cin.ignore(1000, '\n')
to discard any remaining characters in the input buffer. This is especially important after reading the choice character, as it ensures that the newline character does not affect the next loop iteration.
Using ignore()
in this context allows us to create a smooth user experience, preventing any unintended interruptions in the input process.
Handling Input Errors with ignore()
Another common scenario where ignore()
is useful is when dealing with input errors. If a user inputs a non-integer value when an integer is expected, it can cause the input stream to enter a fail state. We can use ignore()
to clear the erroneous input and reset the state of the input stream.
#include <iostream>
using namespace std;
int main() {
int number;
while (true) {
cout << "Enter an integer: ";
cin >> number;
if (cin.fail()) {
cin.clear(); // Clear the error state
cin.ignore(1000, '\n'); // Ignore the invalid input
cout << "Invalid input. Please enter an integer." << endl;
} else {
cin.ignore(1000, '\n'); // Ignore the rest of the line
cout << "You entered: " << number << endl;
break; // Exit the loop if the input is valid
}
}
return 0;
}
Output:
Enter an integer: abc
Invalid input. Please enter an integer.
Enter an integer: 30
You entered: 30
In this example, we use a loop to repeatedly prompt the user to enter an integer. If the user enters a non-integer value, cin.fail()
will return true. We then clear the error state with cin.clear()
and use cin.ignore(1000, '\n')
to discard the invalid input. This allows the program to continue running smoothly, prompting the user for valid input without crashing.
Using ignore()
in combination with error handling techniques is a powerful way to ensure that your program remains robust and user-friendly.
Conclusion
The ignore()
function in C++ is a versatile tool for managing input streams, allowing you to skip unwanted characters and handle user input gracefully. Whether you’re reading a single value, processing multiple inputs, or handling errors, mastering the use of ignore()
can significantly enhance the robustness of your applications. By incorporating this function into your input handling routines, you can create a smoother and more efficient user experience.
FAQ
-
What is the purpose of the
ignore()
function in C++?
theignore()
function is used to skip characters in the input stream, allowing you to manage unwanted data effectively. -
Can ignore() be used with other input types besides integers?
Yes, ignore() can be used with any input type, including strings and characters, to manage the input buffer. -
How does ignore() affect the input stream’s state?
theignore()
function does not change the state of the input stream; however, it can help clear unwanted characters that may cause errors. -
Is there a default value for the parameters in ignore()?
Yes, the default value for the number of characters to ignore is 1, and the default delimiter is EOF. -
Can I use ignore() to skip specific characters?
Yes, you can specify a delimiter in theignore()
function to skip characters until that delimiter is encountered.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook