How to Print a String in C++
-
Use
std::cout
and the<<
Operator to Print a String -
Use the
std::copy
Algorithm to Print a String -
Use the
printf()
Function to Print a String - Conclusion
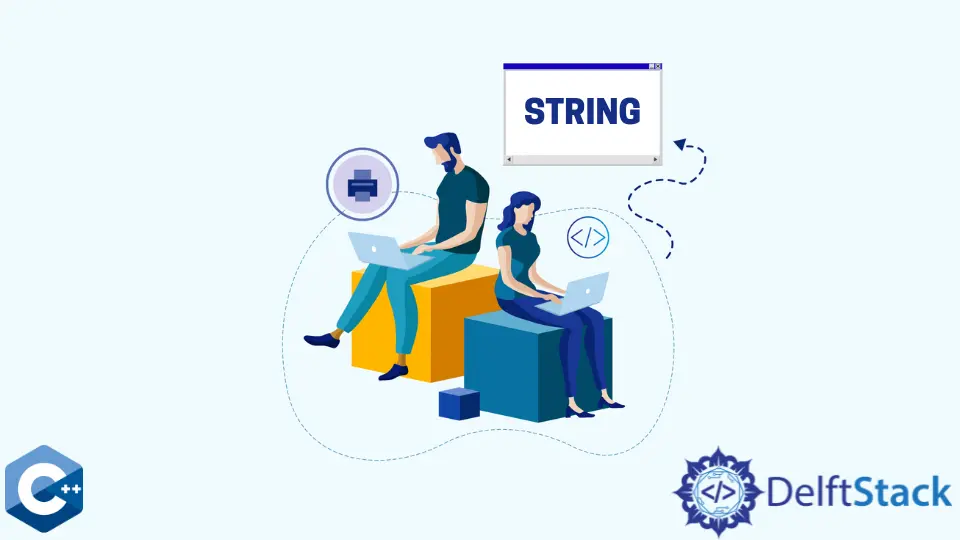
Printing strings is a fundamental task in programming, and C++ offers various methods to accomplish this task efficiently and effectively.
This article will demonstrate multiple methods of how to print a string in C++.
Use std::cout
and the <<
Operator to Print a String
The std::cout
is the global object for controlling the output to a stream buffer. To output the s1
string variable to the buffer, we need to use the operator - <<
called the stream insertion operator.
The following example demonstrates a single-string output operation.
Example Code:
#include <iostream>
#include <iterator>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string s1 = "This string will be printed";
cout << s1;
cout << endl;
return EXIT_SUCCESS;
}
Output:
This string will be printed
In this code, we begin by including essential libraries, such as <iostream>
, <iterator>
, and <string>
. We then declare a string
variable named s1
and assign it the value "This string will be printed"
.
Next, we use the cout
object, part of the Standard Library, to display the content of s1
. The <<
operator is employed to direct the string to the output stream, and following this, we insert endl
to create a newline, ensuring that the text is displayed on a new line.
When we execute this program, the output will be This string will be printed
followed by a newline.
Use the std::copy
Algorithm to Print a String
The copy
method is from the <algorithm>
STL library, and it can manipulate the range elements in multiple ways. Since we can access the string
container itself as a range, we can output each element by adding the std::ostream_iterator<char>
argument to the copy
algorithm.
Notice that this method can also pass a specific delimiter between each character of the string. In the following code, no delimiter (""
) is specified to print the string in its original form.
Example Code:
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string s1 = "This string will be printed";
copy(s1.begin(), s1.end(), std::ostream_iterator<char>(cout, ""));
cout << endl;
return EXIT_SUCCESS;
}
Output:
This string will be printed
In this code, we create a string
variable named s1
and assign it the text "This string will be printed"
. Next, we use the copy
function from the Standard Template Library (STL) to output the characters of the string s1
.
The copy
function is a powerful tool that allows us to manipulate elements within a range. In this case, we specify the range to be from the beginning (s1.begin()
) to the end (s1.end()
) of the string s1
.
To control where the characters are displayed, we use std::ostream_iterator<char>(cout, "")
.This iterator directs the output to the standard output stream(cout
) without any delimiters between characters, thanks to the empty string ""
.
Finally, we insert cout << endl;
to add a newline, ensuring that the printed text appears on a new line in the console. When we run this program, the output will be This string will be printed
.
Use the printf()
Function to Print a String
The printf
is a powerful tool used for formatted output. It is part of the C standard input-output library.
The function can be called from C++ code directly. printf
has the variable number of parameters, and it takes string variable as char *
type, which means we have to call the c_str
method from s1
variable to pass it as an argument.
Note that each type has its format specifier (e.g., string
- %s
), which is listed in the following table.
Specifier | Description |
---|---|
% |
Prints a literal % character (this type doesn’t accept any flags, width, precision, or length fields). |
d, i |
int as a signed integer. %d and %i are similar for output but are different when used with scanf for input (where using %i will interpret a number as hexadecimal if it’s preceded by 0x , and octal if it’s preceded by 0 .) |
u |
Print decimal unsigned int . |
f, F |
double in normal (fixed-point) notation. The printing of the strings for an infinite number or NaN is the only difference between f and F (inf, infinity, and nan for f ; INF, INFINITY, and NAN for F ). |
e, E |
double value in standard form (d.ddde±dd ). An E conversion uses the letter E (rather than e ) to introduce the exponent. |
g, G |
double, expressed in exponential or normal notation, depending on which is more appropriate given its size. Letters G are uppercase, and letters g are lowercase. |
x, X |
unsigned int represented in hexadecimal. Whereas X employs upper-case letters, x utilizes lowercase letters. |
o |
unsigned int in octal. |
s |
null-terminated string. |
c |
char (character). |
p |
void* (pointer to void) in an implementation-defined format. |
a, A |
double in hexadecimal notation, starting with 0x or 0X . a uses lowercase letters, and A uses upper-case letters. |
n |
Nothing is printed, but the number of characters successfully written so far is stored in an integer pointer argument. |
Example Code:
#include <iostream>
#include <iterator>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string s1 = "This string will be printed";
cout << s1;
cout << endl;
printf("%s", s1.c_str());
cout << endl;
return EXIT_SUCCESS;
}
Output:
This string will be printed
This string will be printed
In this code, we declare a string
variable named s1
and assign it the text "This string will be printed"
. Next, we first use cout
, which is the standard output stream, to display the content of s1
, and the line cout << s1;
effectively prints the string to the console.
Next, we use the printf()
function, which is part of the C standard input-output library. It allows us to perform formatted output.
In this case, we use the format specifier %s
to indicate that we want to print a string. To use printf()
with a C++ string, we must call the c_str()
method on the s1
variable to convert it to a C-style string, and this C-style string is then passed as an argument to printf()
.
Finally, we insert cout << endl;
to create a newline, ensuring that the text is displayed on a new line in the console.
When we execute this program, the output will be This string will be printed
twice, each followed by a newline. The first line is printed using cout
, and the second using printf()
.
Conclusion
This article has provided various techniques for printing strings in C++. Whether we choose the straightforward std::cout
and <<
operator, the versatile std::copy
algorithm, or the powerful printf()
function, we have a range of options to suit our specific programming needs and the choice of method ultimately depends on our project’s requirements and personal coding preferences.
By mastering these methods, we’ll be well-equipped to handle string output effectively in our C++ programs.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++