How to Generate a Random Float Number in C++
-
Use C++11
<random>
Library to Generate a Random Float -
Use the
rand
Function to Generate a Random Float -
Use
<random>
Library With<chrono>
Seed to Generate a Random Float - Conclusion
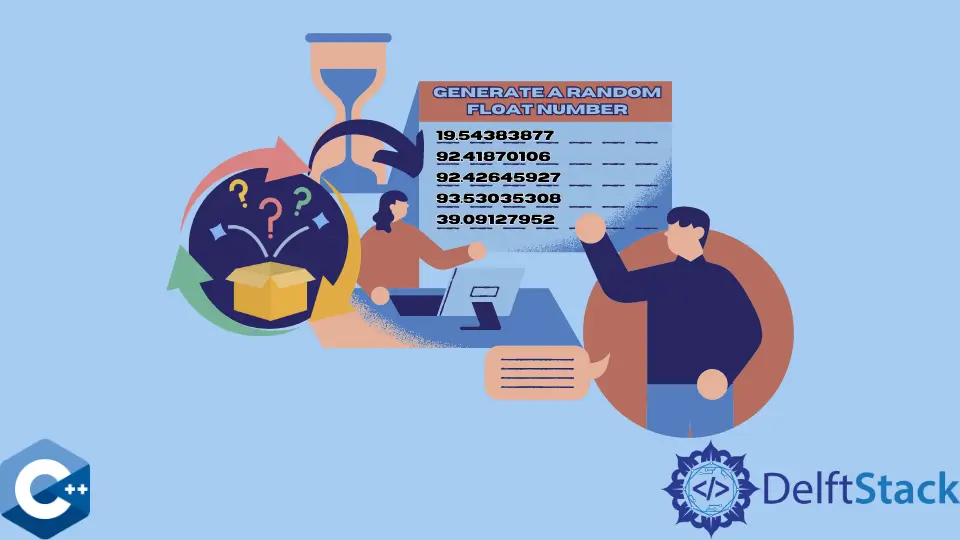
Generating random floating-point numbers is a common requirement in diverse applications, ranging from simulations to game development, where randomness is essential for creating realistic and unpredictable scenarios. In this comprehensive guide, we discuss various methods in C++ to accomplish this task.
Use C++11 <random>
Library to Generate a Random Float
The C++11 <random>
library provides a recommended way to generate high-quality random numbers in contemporary C++. In this method, the std::random_device
object is initialized to produce non-deterministic random bits for seeding the random engine and this is crucial to avoid producing the same number sequences.
We use std::default_random_engine
to generate pseudo-random values, and a uniform distribution is initialized with min/max values as optional arguments.
Code:
#include <chrono>
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
constexpr int FLOAT_MIN = 10;
constexpr int FLOAT_MAX = 100;
int main() {
std::random_device rd1;
std::default_random_engine eng1(rd1());
std::uniform_real_distribution<float> distr1(FLOAT_MIN, FLOAT_MAX);
for (int n = 0; n < 5; ++n) {
cout << setprecision(10) << distr1(eng1) << "\n";
}
cout << endl;
return EXIT_SUCCESS;
}
In this code, we utilize the <random>
library to generate five random floating-point numbers within the specified range of 10 to 100. We start by initializing a random device (rd1
) to obtain non-deterministic random bits and then use it to seed a default random engine (eng1
).
A uniform real distribution (distr1
) is set up with the given minimum and maximum values. Inside the loop, we generate and output these random float numbers to the console with a precision of 10 decimal places.
The use of setprecision(10)
ensures an accurate representation of the floating-point values.
Output:
90.40170288
61.41258621
11.38827324
32.71624756
21.94068146
The output displays five random float numbers within the specified range, each with 10 decimal places of precision.
Use the rand
Function to Generate a Random Float
The rand
function, coming from the C library, is an alternative method for generating random float values. However, it is not recommended for high-quality randomness due to limitations in its implementation.
This function generates a pseudo-random integer between 0
and RAND_MAX
(both included). The RAND_MAX
value is implementation-dependent, limiting the randomness.
Code:
#include <chrono>
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
constexpr int FLOAT_MIN = 10;
constexpr int FLOAT_MAX = 100;
int main() {
std::srand(std::time(nullptr));
for (int i = 0; i < 5; i++) {
cout << setprecision(10)
<< FLOAT_MIN +
(float)(rand()) / ((float)(RAND_MAX / (FLOAT_MAX - FLOAT_MIN)))
<< endl;
}
return EXIT_SUCCESS;
}
In this code, we generate five random floating-point numbers within the specified range of 10 to 100 using the traditional rand
function. To ensure varied sequences, we seed the random number generator with the current time.
Inside the loop, we calculate each random float value by combining the minimum value with the scaled result of the rand()
function divided by the range between RAND_MAX
and the specified minimum and maximum values.
Output:
50.13548279
81.41028595
27.23748207
31.59845161
80.02357483
The output displays five random float numbers within the specified range, and each number is presented with 10 decimal places of precision.
Use <random>
Library With <chrono>
Seed to Generate a Random Float
You can seed the random engine using the <chrono>
library to make the seed more dynamic and less predictable. This is particularly useful when you need to generate different random sequences in a short time interval.
Code:
#include <chrono>
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
constexpr int FLOAT_MIN = 10;
constexpr int FLOAT_MAX = 100;
int main() {
auto seed =
std::chrono::high_resolution_clock::now().time_since_epoch().count();
std::default_random_engine eng(seed);
std::uniform_real_distribution<> distr(FLOAT_MIN, FLOAT_MAX);
for (int n = 0; n < 5; ++n) {
cout << setprecision(10) << distr(eng) << "\n";
}
return EXIT_SUCCESS;
}
In this code, we employ the <random>
library to generate five random floating-point numbers within the specified range of 10 to 100. To achieve dynamic and less predictable seeds for the random engine, we use the current time as obtained from std::chrono::high_resolution_clock
.
The random engine (eng
) is then initialized with this seed, and a uniform real distribution (distr
) is set up with the given minimum and maximum values. Within the loop, we generate and output each random float number to the console, ensuring a precision of 10 decimal places.
Output:
36.07038562
19.54420663
48.06368897
53.92516954
75.96040923
The output displays five random float numbers within the specified range, with each number presented accurately with 10 decimal places of precision.
Conclusion
In summary, this guide has explored three distinct methods for generating random floating-point numbers in C++, each catering to specific requirements. The C++11 <random>
library provides a robust and modern solution, ensuring high-quality randomness.
The rand
function, while simple, is not recommended for applications demanding precision. Lastly, the <random>
library with the <chrono>
seed offers dynamic and less predictable randomness, particularly useful for scenarios requiring varied sequences in a short time interval.
The method chosen is determined by the application’s requirements, balancing factors such as simplicity, precision, and unpredictability.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook