How to Extract a Subvector From a Vector in C++
-
Extract a Subvector From a Vector in C++ Using
std::copy
and Iterators - Extract a Subvector from a Vector in C++ Using Iterators
- Extract a Subvector from a Vector in C++ Using std::vector Constructor
- Conclusion
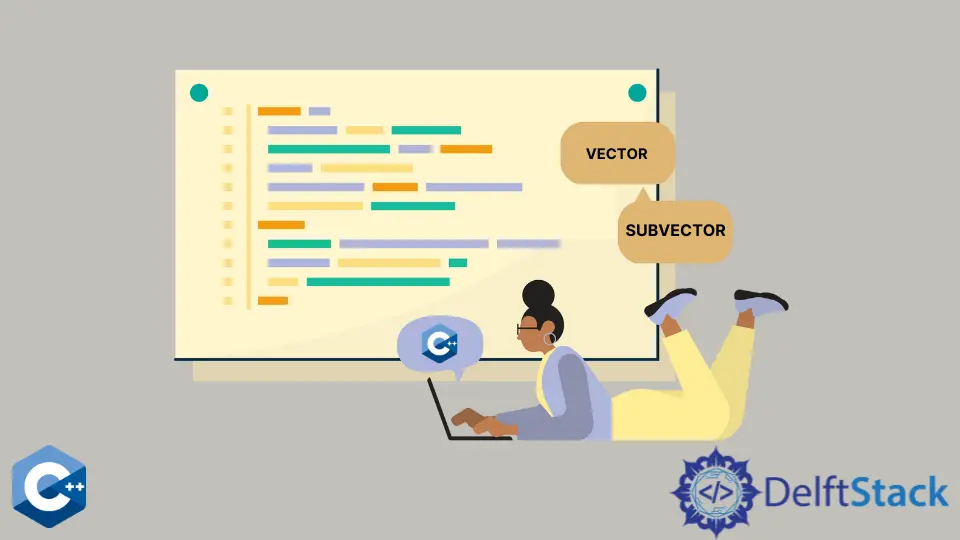
Vectors are a fundamental data structure in C++, offering dynamic arrays that can be resized efficiently. Extracting subvectors from a larger vector is a common task, and C++ provides several approaches to achieve this.
In this comprehensive guide, we’ll explore different methods using iterators and the std::copy
algorithm to extract subvectors.
Extract a Subvector From a Vector in C++ Using std::copy
and Iterators
The std::copy
algorithm in C++ is part of the <algorithm>
header and is designed to copy elements from one range to another. When combined with iterators, it becomes a powerful tool for extracting subvectors.
This method has been available since C++98 and remains relevant in modern C++ programming.
The general syntax for std::copy
when extracting a subvector looks like this:
#include <algorithm>
// Assuming originalVector is the source vector
std::copy(originalVector.begin() + start,
originalVector.begin() + start + length, destinationVector.begin());
Where:
originalVector.begin() + start
: Iterator pointing to the starting position of the subvector within the original vector.originalVector.begin() + start + length
: Iterator pointing to the position one past the end of the desired subvector.destinationVector.begin()
: Iterator pointing to the beginning of the destination vector where the subvector will be copied.
In the context of extracting a subvector, we identify a starting point and an endpoint within the original vector and use iterators to define the source range.
Let’s consider a practical example to illustrate the extraction of a subvector using std::copy
and iterators:
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> originalVector = {1, 2, 3, 4, 5};
size_t start = 1;
size_t length = 3;
std::vector<int> subVector(length);
std::copy(originalVector.begin() + start,
originalVector.begin() + start + length, subVector.begin());
std::cout << "Subvector: ";
for (int num : subVector) {
std::cout << num << " ";
}
return 0;
}
Output:
Subvector: 2 3 4
In this example, we start by defining our original vector (originalVector
) containing integers. We then specify the parameters for our subvector: start
represents the starting index of the subvector, and length
is the number of elements we want in the subvector.
Next, we create a destination vector (subVector
) with a size equal to the desired subvector length. The std::copy
algorithm is employed to copy the elements from the original vector’s specified range (determined by iterators) to the destination vector.
The iterators are calculated based on the start index and length of the subvector.
Finally, we display the extracted subvector using a simple loop.
Extract a Subvector from a Vector in C++ Using Iterators
Iterators provide a flexible means of traversing and manipulating the elements of a container. Iterators are available in C++98 and later versions.
The core idea behind extracting a subvector using iterators involves defining two iterators that mark the beginning and end of the desired subvector within the original vector. The syntax for achieving this looks like the following:
#include <iostream>
#include <vector>
// Assuming originalVector is the source vector
std::vector<int>::iterator first = originalVector.begin() + start;
std::vector<int>::iterator last = first + length;
// Create a new vector using iterators
std::vector<int> subVector(first, last);
Where:
originalVector.begin() + start
: Iterator pointing to the starting position of the subvector within the original vector.first + length
: Iterator pointing to position one past the end of the desired subvector.std::vector<int> subVector(first, last)
: Constructor for a new vector created using the iteratorsfirst
andlast
, effectively forming the subvector.
Let’s dive into a practical example to illustrate the process of extracting a subvector using iterators:
#include <iostream>
#include <vector>
int main() {
std::vector<int> originalVector = {1, 2, 3, 4, 5};
size_t start = 1;
size_t length = 3;
std::vector<int>::iterator first = originalVector.begin() + start;
std::vector<int>::iterator last = first + length;
std::vector<int> subVector(first, last);
std::cout << "Subvector: ";
for (int num : subVector) {
std::cout << num << " ";
}
return 0;
}
Output:
Subvector: 2 3 4
In this example, we start with the definition of our original vector (originalVector
) containing integers.
We then specify the parameters for our subvector: start
, indicating the starting index of the subvector, and length
, representing the number of elements we want in the subvector.
Next, we create two iterators, first
and last
, to mark the beginning and end of the subvector within the original vector. The subvector is then constructed using these iterators, effectively extracting the desired portion of the original vector.
Finally, we display the extracted subvector using a simple loop. This approach using iterators provides a clear and efficient way to extract a subvector from a larger vector in C++, offering both simplicity and versatility in code.
Extract a Subvector from a Vector in C++ Using std::vector Constructor
Another way we can extract a subvector from a larger vector is by using the std::vector
constructor. It allows us to create a new vector by specifying a range defined by two iterators.
To extract a subvector, we can use iterators to mark the beginning and end positions of the desired portion in the original vector.
The syntax for this operation is as follows:
#include <iostream>
#include <vector>
// Assuming originalVector is the source vector
std::vector<int> subVector(originalVector.begin() + start,
originalVector.begin() + start + length);
Where:
originalVector.begin() + start
: Iterator pointing to the starting position of the subvector within the original vector.originalVector.begin() + start + length
: Iterator pointing to the position one past the end of the desired subvector.std::vector<int> subVector(...)
: Constructor for a new vector created using the specified iterators, effectively forming the subvector.
Let’s walk through a practical example to illustrate the process of extracting a subvector using the std::vector
constructor:
#include <iostream>
#include <vector>
int main() {
std::vector<int> originalVector = {1, 2, 3, 4, 5};
size_t start = 1;
size_t length = 3;
std::vector<int> subVector(originalVector.begin() + start,
originalVector.begin() + start + length);
std::cout << "Subvector: ";
for (int num : subVector) {
std::cout << num << " ";
}
return 0;
}
Output:
Subvector: 2 3 4
In this example, we start with the definition of our original vector (originalVector
) containing integers. The parameters for our subvector are specified: start
, indicating the starting index of the subvector, and length
, representing the number of elements we want in the subvector.
Using the std::vector
constructor, we create a new vector (subVector
) by providing the iterators that mark the beginning and end positions of the desired subvector within the original vector. This concise approach simplifies the extraction process.
Finally, we display the extracted subvector using a simple loop. The use of the std::vector
constructor in C++11 and later versions offers a clean and efficient way to create a subvector from a larger vector, promoting readability and ease of use in your code.
Conclusion
Extracting subvectors from vectors in C++ is a common operation with multiple approaches. Whether using the std::copy
algorithm with iterators, explicit iterators, or the std::vector
constructor, each method provides a clean and efficient way to achieve the desired result.
Choose the approach that best fits your specific use case and coding style.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook