How to Determine if a String Is a Number in C++
-
Use the
std::isdigit
Method to Determine if a String Is a Number -
Use
std::isdigit
Withstd::all_of
to Determine if a String Is a Number -
Use the
find_first_not_of
Method to Determine if a String Is a Number -
Use
std::stoi
,std::stof
, orstd::stod
to Determine if a String Is a Number -
Use
std::regex
to Determine if a String Is a Number - Conclusion
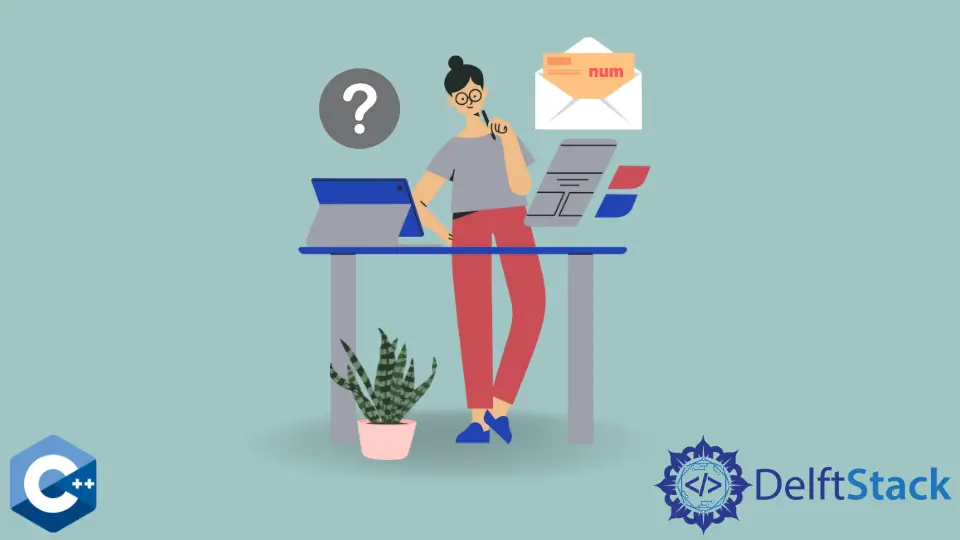
Determining whether a given string is a number is a common task in C++ programming.
This article explores various methods to achieve this, ranging from simple approaches like checking for digits using std::isdigit
to more advanced techniques involving regular expressions and string-to-number conversion functions.
Use the std::isdigit
Method to Determine if a String Is a Number
One simple and effective approach to determine whether a given string represents a numeric value involves utilizing the std::isdigit
function. This function is part of the C++ Standard Library and is particularly handy for checking if individual characters are digits.
The std::isdigit
function is defined in the <cctype>
header, and it takes an integer or character as an argument. It returns a non-zero value if the character is a digit (0-9
), and 0
otherwise.
int isdigit(int c);
Here’s a complete working example demonstrating how to use std::isdigit
to determine if a given string is a number:
#include <cctype>
#include <iostream>
bool isNumber(const std::string& str) {
for (char c : str) {
if (!std::isdigit(c)) {
return false;
}
}
return true;
}
int main() {
std::string input;
std::cout << "Enter a string: ";
std::cin >> input;
if (isNumber(input)) {
std::cout << "The entered string is a number.\n";
} else {
std::cout << "The entered string is not a number.\n";
}
return 0;
}
As you can see in the isNumber
function, we iterate through each character of the input string using a range-based for
loop. For each character, we use std::isdigit
to check if it is a digit.
If any character is found not to be a digit, the function returns false
, indicating that the string is not a number. If all characters are digits, the function returns true
.
In the main
function, we take user input and call the isNumber
function to determine if the entered string is a number. The result is then printed to the console.
Output:
Enter a string: 1
The entered string is a number.
This approach is straightforward and suitable for cases where a string is considered a number only if all its characters are digits. Keep in mind that it won’t handle cases involving decimals, negative signs, or exponentials.
If more complex numeric validation is required, alternative methods like using std::stoi
, std::stof
, or regular expressions may be more appropriate.
Use std::isdigit
With std::all_of
to Determine if a String Is a Number
Expanding on our exploration of checking if a string is a number in C++, let’s delve into another approach, this time incorporating std::isdigit
with std::all_of
.
std::all_of
is an algorithm introduced in the <algorithm>
header of the C++ Standard Library. It checks whether a given predicate is true for all elements in a specified range.
In our case, the range is the sequence of characters in the string, and the predicate is provided by std::isdigit
.
The syntax of std::all_of
is defined as follows:
template <class InputIt, class UnaryPredicate>
bool all_of(InputIt first, InputIt last, UnaryPredicate pred);
Where:
first
,last
: The range of elements to examine.pred
: Unary predicate function that returnstrue
for the required condition.
The function returns true
if the predicate p
is true
for all elements in the range [first, last)
, and false
otherwise.
Here’s a complete working code example:
#include <algorithm>
#include <cctype>
#include <iostream>
bool isNumber(const std::string& str) {
return std::all_of(str.begin(), str.end(),
[](unsigned char c) { return std::isdigit(c); });
}
int main() {
std::string testString =
"42a7"; // Note: This string contains a non-digit character.
if (isNumber(testString)) {
std::cout << "The string is a number." << std::endl;
} else {
std::cout << "The string is not a number." << std::endl;
}
return 0;
}
In this example, the isNumber
function employs std::all_of
to determine if all characters in the given string satisfy the condition specified by the lambda function within the algorithm. The lambda function checks if each character is a digit using std::isdigit
.
The std::all_of
algorithm iterates through the entire range of characters, and if the predicate is true
for all elements, it returns true
; otherwise, it returns false
.
In the main
function, a test string (42a7
) is passed to the isNumber
function. This string intentionally contains a non-digit character (a
).
Output:
The string is not a number.
By combining the power of std::isdigit
with the expressive simplicity of std::all_of
, this approach offers a concise and effective solution for determining if a given string is a number in C++.
Use the find_first_not_of
Method to Determine if a String Is a Number
find_first_not_of
is a member function of the std::string
class, residing in the <string>
header. It searches the string for the first occurrence of a character not included in a specified set and returns its position.
Syntax:
size_t find_first_not_of(const std::string& str, const std::string& chars,
size_t pos = 0);
Where:
str
: The string to search.chars
: The set of characters to consider as “not wanted”.pos
: The position in the string to start the search from (default is0
).
Let’s see a complete working example:
#include <iostream>
#include <string>
bool isNumber(const std::string& str) {
size_t found = str.find_first_not_of("0123456789");
return (found == std::string::npos);
}
int main() {
std::string testString = "314159";
if (isNumber(testString)) {
std::cout << "The string is a number." << std::endl;
} else {
std::cout << "The string is not a number." << std::endl;
}
return 0;
}
In this example, the isNumber
function utilizes find_first_not_of
to search for the first character in the given string (testString
) that is not a digit. The set of characters considered as “not wanted” is defined by the string 0123456789
.
If no such character is found (i.e., find_first_not_of
returns std::string::npos
), the function concludes that all characters in the string are digits and, therefore, returns true
.
In the main
function, a test string (314159
) is passed to the isNumber
function. Since all characters in this string are digits, the program outputs:
The string is a number.
This approach provides a concise and efficient means of determining if a given string represents a numeric value in C++ by checking for the absence of non-digit characters using find_first_not_of
.
Use std::stoi
, std::stof
, or std::stod
to Determine if a String Is a Number
When it comes to parsing and identifying numbers within strings in C++, the trio of functions - std::stoi
, std::stof
, and std::stod
- emerges as another powerful toolset.
The std::stoi
, std::stof
, and std::stod
functions serve as integral components of the C++ Standard Library for string-to-number conversions.
std::stoi
: Converts strings to integers. It takes a string as input and, optionally, pointers to asize_t
object to store the position of the first unconverted character and an integer specifying the numeric base.std::stof
: Converts strings to floats. Similar tostd::stoi
, it takes a string and an optional pointer to store the position of the first unconverted character.std::stod
: Converts strings to doubles. Like the other two, it takes a string and an optional pointer to store the position of the first unconverted character.
Syntax:
int stoi(const std::string& str, size_t* pos = 0, int base = 10);
float stof(const std::string& str, size_t* pos = 0);
double stod(const std::string& str, size_t* pos = 0);
Where:
str
: The string to be converted to an integer.pos
(optional): A pointer to asize_t
object where the position of the first unconverted character will be stored. If specified, after the function call, this pointer will point to the first character that is not part of the integral representation in the string.base
(optional): Specifies the numeric base for the conversion. It can be any value between2
and36
. If not provided, the default base is10
.
Take a look at the complete code example below:
#include <iostream>
#include <string>
bool isNumber(const std::string& str) {
try {
size_t pos;
std::stoi(str, &pos);
return pos == str.length();
} catch (...) {
return false;
}
}
int main() {
std::string testString = "12345";
if (isNumber(testString)) {
std::cout << "The string is a number." << std::endl;
} else {
std::cout << "The string is not a number." << std::endl;
}
return 0;
}
Here, the isNumber
function attempts to convert the given string (testString
) to an integer using std::stoi
. The function uses a try-catch
block to handle potential exceptions that might arise during the conversion, such as an invalid format.
If the conversion is successful, it retrieves the position of the first unconverted character (stored in the pos
variable) and checks if it matches the length of the original string. If it does, the function concludes that the entire string has been converted, indicating that it is a valid number.
If an exception is caught during the conversion, the function returns false
.
In the main
function, a test string is passed to the isNumber
function. As this string represents a valid integer, the program outputs:
The string is a number.
The utilization of std::stoi
in combination with exception handling provides a robust and concise solution for determining if a given string is a number in C++.
Use std::regex
to Determine if a String Is a Number
Another tool at our disposal for validating whether a given string represents a number is the <regex>
library, which provides facilities for regular expressions.
Syntax:
bool std::regex_match(const std::string& str, const std::regex& rgx);
Where:
str
: The string to be matched against the regular expression.rgx
: The regular expression object representing the pattern to match.
To employ std::regex
for checking if a string is a number, we can create a regular expression pattern that matches numeric values - \d+(\.\d+)?
.
Breaking it down:
\d+
matches one or more digits.(\.\d+)?
is an optional group that allows for a decimal point followed by one or more digits.
With this regular expression, we can define a std::regex
object and use std::regex_match
to check if the entire string adheres to the pattern, indicating it is a number.
Code Example:
#include <iostream>
#include <regex>
bool isNumber(const std::string& str) {
std::regex pattern("\\d+(\\.\\d+)?");
return std::regex_match(str, pattern);
}
int main() {
std::cout << "Is '123' a number? " << (isNumber("123") ? "Yes" : "No")
<< std::endl;
std::cout << "Is '3.14' a number? " << (isNumber("3.14") ? "Yes" : "No")
<< std::endl;
std::cout << "Is 'abc' a number? " << (isNumber("abc") ? "Yes" : "No")
<< std::endl;
return 0;
}
In the code example above, we define a function isNumber
that takes a string as an argument and returns a boolean value. Inside this function, we create a std::regex
object named pattern
initialized with the regular expression \d+(\.\d+)?
.
The std::regex_match
function is then used to check if the entire input string matches the defined pattern.
In the main
function, we provide test cases to demonstrate the functionality. The program outputs whether each input string is a number or not based on the defined regular expression.
Output:
Is '123' a number? Yes
Is '3.14' a number? Yes
Is 'abc' a number? No
Here, 123
is recognized as a number, 3.14
is a valid floating-point number, and abc
does not match the numeric pattern, thus confirming that the implementation effectively determines whether a given string is a number using std::regex
.
Conclusion
Choosing the appropriate method for determining if a given string is a number in C++ depends on the specific requirements of your application. Whether opting for a simple digit-checking loop, utilizing algorithms like std::all_of
, employing regular expressions, or leveraging string-to-number conversion functions, each approach has its strengths and can be tailored to suit different scenarios.
Selecting the most suitable method involves considering factors such as performance, readability, and the specific constraints of the problem at hand.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++