How to Convert Int to a String in C++
- Use Stringizing Macro to Convert Int Literal to a String
-
Use
to_string()
Method for Int to String Conversion -
Use
std::stringstream
Class andstr()
Method for Int to String Conversion -
Use
std::to_chars
Method for Int to String Conversion
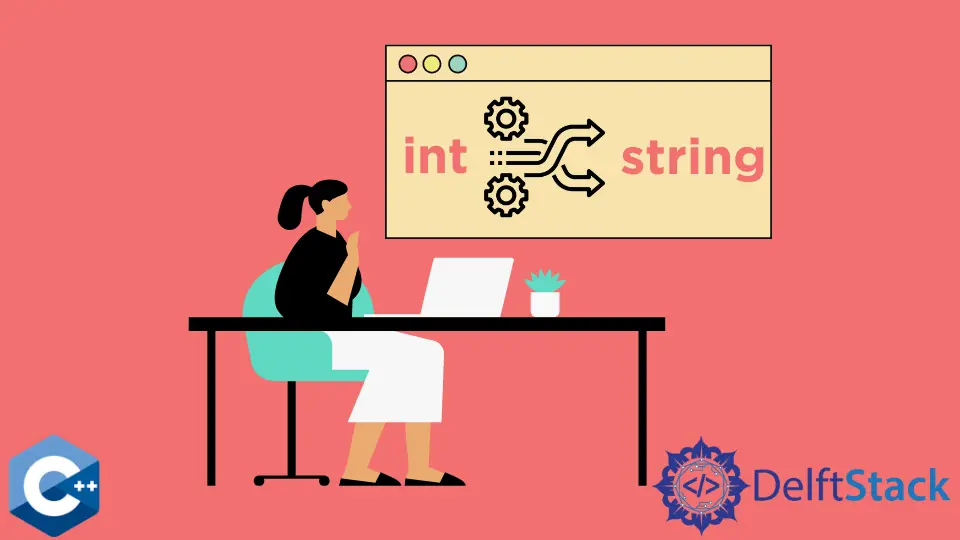
This article will introduce C++ methods to convert an int
to a string
.
Use Stringizing Macro to Convert Int Literal to a String
This method has quite a limited use when it comes to an int to string conversion. Namely, it can only be utilized when so-called hard-coded numeric values need to be converted to a string
type.
Macros are code blocks the programmer assigns the name to, and whenever the name is used, it is replaced with macro expansion(the right part of the macro statement).
This is a C/C++ preprocessor feature, which means you can only use this with literal values.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
#define STRING(num) #num
int main() {
string num_cstr(STRING(1234));
num_cstr.empty() ? cout << "empty\n" : cout << num_cstr << endl;
return EXIT_SUCCESS;
}
Output:
1234
Notice, though, when you want to stringize the result of other macro expansion (in this example NUMBER
which expands to 123123
), you would need to define two-level macro as shown in the following code block:
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
#define STRING(num) STR(num)
#define STR(num) #num
#define NUMBER 123123
int main() {
string num_cstr(STRING(NUMBER));
num_cstr.empty() ? cout << "empty\n" : cout << num_cstr << endl;
return EXIT_SUCCESS;
}
Output:
123123
Use to_string()
Method for Int to String Conversion
to_string
is a built-in <string>
library function, which takes a single numeric value as an argument and returns string
object. This method is the recommended solution for this problem. However, be aware that passing the floating-point values to the to_string
method yields some unexpected results, as demonstrated in the following code example:
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
int n1 = 9876;
double n2 = 0.0000000000000000000001;
double n3 = 2.000000000000123;
string num_str1(std::to_string(n1));
string num_str2(std::to_string(n2));
string num_str3(std::to_string(n3));
num_str1.empty() ? cout << "empty\n" : cout << num_str1 << endl;
num_str1.empty() ? cout << "empty\n" : cout << num_str2 << endl;
num_str1.empty() ? cout << "empty\n" : cout << num_str3 << endl;
return EXIT_SUCCESS;
}
Output:
9876
0.000000
2.000000
Use std::stringstream
Class and str()
Method for Int to String Conversion
Another alternative to solve this problem is to use the stringstream
class, which stores string
instance internally and provides the str()
method to retrieve the string
object from stringstream
contents.
#include <iostream>
#include <sstream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
int n1 = 9876;
std::stringstream sstream;
sstream << n1;
string num_str = sstream.str();
num_str.empty() ? cout << "empty\n" : cout << num_str << endl;
return EXIT_SUCCESS;
}
Output:
9876
Use std::to_chars
Method for Int to String Conversion
The following method is relatively cumbersome compared to others, as it requires a temporary char
array to be initialized for converted result storage. Although, on the plus side, this method is locale-independent, non-allocating, and non-throwing. to_chars
function takes the range of char
array and converts the integer to a character string. Once the characters are stored in arr
variable, a new string
object is initialized with arr.data()
argument.
#include <array>
#include <charconv>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
#define MAX_DIGITS 100
int main() {
int n1 = 9876;
std::array<char, MAX_DIGITS> arr{};
std::to_chars(arr.data(), arr.data() + arr.size(), n1);
string num_str(arr.data());
cout << num_str << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook