How to Convert Int to ASCII Char in C++
-
Use
int
tochar
Assignment to Convertint
to ASCIIchar
-
Use the
sprintf()
Function to Convertint
to ASCIIchar
-
Use Type Casting to Convert
int
to ASCIIchar
- Conclusion
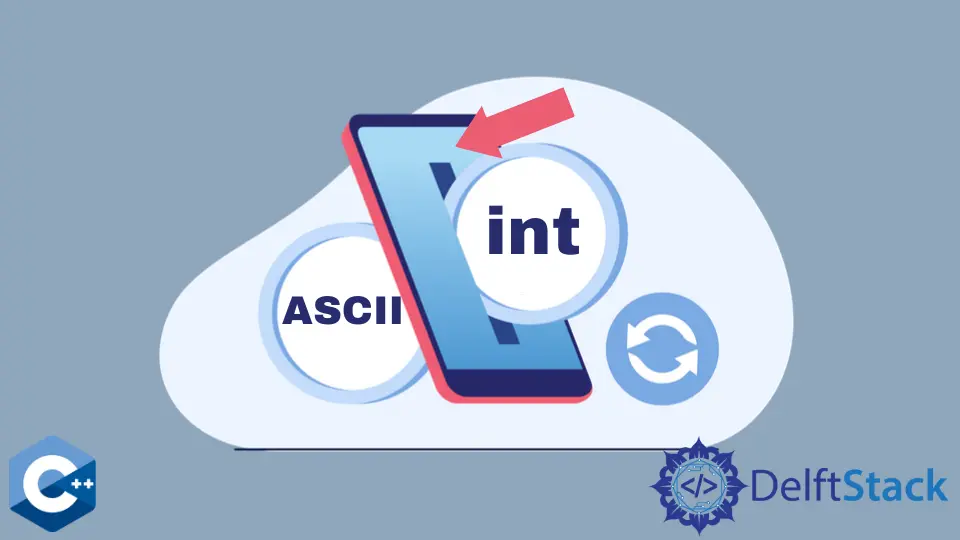
This article will explain several methods of how to convert int
to ASCII char
in C++. From straightforward int
to char
assignment to using printf
, sprintf
, and type casting, each method is examined, offering flexibility to suit various programming needs.
Use int
to char
Assignment to Convert int
to ASCII char
ASCII character encoding is specified in a 7-bit format. Thus, there are 128 unique characters, each mapping to the corresponding numeric value from 0
to 127
.
Since the C programming language implemented char
types as numbers underneath the hood, we can assign an int
variable to a char
type variable and treat that as the corresponding ASCII character. For example, we directly push values from the int
vector to the char
vector and then output them using the std::copy
method, displaying ASCII characters as expected.
Note that assigning to char
type only works when the int
value corresponds to an ASCII code, e.i. is in the range 0
-127
.
#include <array>
#include <iostream>
#include <iterator>
#include <vector>
using std::array;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> numbers{97, 98, 99, 100, 101, 102, 103};
vector<char> chars{};
std::copy(numbers.begin(), numbers.end(),
std::ostream_iterator<int>(cout, "; "));
chars.reserve(numbers.size());
for (auto &number : numbers) {
chars.push_back(number);
}
cout << endl;
std::copy(chars.begin(), chars.end(),
std::ostream_iterator<char>(cout, "; "));
return EXIT_SUCCESS;
}
Output:
97; 98; 99; 100; 101; 102; 103;
a; b; c; d; e; f; g;
In the code above, we start by defining a vector named numbers
, containing integer values corresponding to ASCII codes for characters 'a'
to 'g'
. Using the std::copy
function, we print the original integer values to the console separated by semicolons and spaces.
Following this, we create an empty vector called chars
to store ASCII characters. We then optimize memory usage by reserving space in the chars
vector based on the size of the numbers
vector.
In a subsequent loop, we iterate through each integer in the numbers
vector and use the push_back
method to populate the chars
vector with ASCII characters corresponding to the integers. Finally, we employ another std::copy
function to print the converted ASCII characters to the console, again separated by semicolons and spaces.
The program concludes by printing a newline character for better formatting. This code demonstrates an effective way to convert and display both original integer values and their corresponding ASCII characters concisely.
Alternatively, we can directly output int
values as ASCII characters using the printf
function. printf
takes format specifier for the corresponding type argument, as shown in the following table.
Specifier | Description |
---|---|
% |
Prints a literal % character (this type doesn’t accept any flags, width, precision, or length fields). |
d, i |
The int as a signed integer. The %d and %i are synonymous for output but are different when used with the scanf command for input (where using %i will interpret a number as hexadecimal if it is preceded by 0x and octal if it is preceded by 0 ). |
u |
Prints decimal unsigned int . |
f, F |
Double in normal (fixed-point) notation. The f and F differ in how the strings for an infinite number or NaN are printed (f : inf , infinity , nan and F : INF , INFINITY , and NAN ). |
e, E |
The double value in standard form (d.ddde±dd ). An E conversion introduce the exponent using the letter E (rather than e ). |
g, G |
The double in either normal or exponential notation, whichever is more appropriate for its magnitude. g uses lower-case letters, and G uses upper-case letters. |
x, X |
The unsigned int as a hexadecimal number. The x uses lower-case letters, and X uses upper-case. |
o |
This is the unsigned int in octal. |
s |
This is the null-terminated string. |
c |
This is the char (character). |
p |
This is the void* (pointer to void) in an implementation-defined format. |
a, A |
The double in hexadecimal notation, starting with 0x or 0X . The a uses lower-case letters, and A uses upper-case letters. |
n |
Prints nothing but writes the number of characters written so far into an integer pointer parameter. |
#include <array>
#include <charconv>
#include <iostream>
#include <iterator>
#include <vector>
using std::array;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> numbers{97, 98, 99, 100, 101, 102, 103};
vector<char> chars{};
for (const auto &number : numbers) {
printf("%c; ", number);
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
a; b; c; d; e; f; g;
In this code snippet, we begin by initializing a vector called numbers
containing integer values corresponding to ASCII codes for characters 'a'
to 'g'
. Subsequently, we iterate through each element in the numbers
vector using a for loop.
Within the loop, we use the printf
function to print each integer as its corresponding ASCII character, followed by a semicolon and a space. This concise code efficiently outputs the ASCII characters associated with the given integer values to the console.
Finally, we utilize cout
to print a newline character, enhancing the output’s readability. The code concludes with a successful exit status denoted by EXIT_SUCCESS
.
Use the sprintf()
Function to Convert int
to ASCII char
The sprintf
function is another method to convert int
values to characters. We must declare a char
variable to hold the converted value.
This variable is passed as the first argument to sprintf
, and the third parameter specifies the number to be processed. Finally, you should provide one of the format specifiers, as with printf
, it indicates the type to which the value will be converted.
#include <array>
#include <charconv>
#include <iostream>
#include <iterator>
#include <vector>
using std::array;
using std::copy;
using std::cout;
using std::endl;
using std::to_chars;
using std::vector;
int main() {
vector<int> numbers{97, 98, 99, 100, 101, 102, 103};
array<char, 5> char_arr{};
for (auto &number : numbers) {
sprintf(char_arr.data(), "%c", number);
printf("%s; ", char_arr.data());
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
a; b; c; d; e; f; g;
In this code, we initialize a vector called numbers
containing integers representing ASCII codes for characters 'a'
to 'g'
. Additionally, we declare an array named char_arr
capable of storing up to 5
characters.
Within a for
loop, we iterate through each integer in the numbers
vector. Using the sprintf
function, we convert each integer to its corresponding ASCII character and store it in the char_arr
array.
Subsequently, we utilize printf
to print the converted ASCII character, followed by a semicolon and a space. This process is repeated for each integer in the vector.
Finally, we use cout
to print a newline character, enhancing the output’s readability. The program concludes with a successful exit status denoted by EXIT_SUCCESS
.
Use Type Casting to Convert int
to ASCII char
Using type casting to convert an integer to an ASCII character involves casting the integer value to a char
type.
Keep in mind that this method assumes that the integer value is within the valid ASCII range (0 to 127
). If the integer is outside this range, the result might not represent a valid ASCII character.
Additionally, the behavior is undefined if you try to convert a negative integer to a char
using this method.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers{97, 98, 99, 100, 101, 102, 103};
for (const auto &number : numbers) {
char asciiChar = static_cast<char>(number);
std::cout << asciiChar << "; ";
}
std::cout << "\n\n";
return EXIT_SUCCESS;
}
Output:
a; b; c; d; e; f; g;
In this code snippet, we initialize a vector named numbers
containing integers representing ASCII codes for characters 'a'
to 'g'
. Using a for
loop, we iterate through each integer in the numbers
vector.
Inside the for
loop, we employ type casting (static_cast<char>
) to convert each integer to its corresponding ASCII character, storing the result in a variable named asciiChar
. We then use std::cout
to print the ASCII character followed by a semicolon and a space.
This process is repeated for each integer in the vector, producing a clear and concise output. Finally, we use std::cout
to print two newline characters for better formatting.
The program concludes with a successful exit status denoted by EXIT_SUCCESS
.
Conclusion
The article explores different methods to convert integers to ASCII characters in C++.
The first method employs int
to char
assignment, utilizing the numeric nature of char types. The second method uses the printf
function with %c
format specifiers for direct conversion.
Another approach involves the sprintf
function for integer-to-character conversion. Lastly, type casting is demonstrated, directly converting integers to char
types.
Each method provides distinct strategies for achieving the conversion, catering to different programming scenarios.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook