How to Find Length of an Array in C++
-
Use
for
Loop to Calculate Array Length in C++ -
Use
sizeof()
Function to Calculate Array Length in C++ -
Use
array.size()
Function to Calculate Array Length in C++ -
Use
begin(arr)
andend(arr)
Functions to Calculate Array Length in C++ -
Use the
std::size
Method to Calculate Array Length in C++ - Conclusion
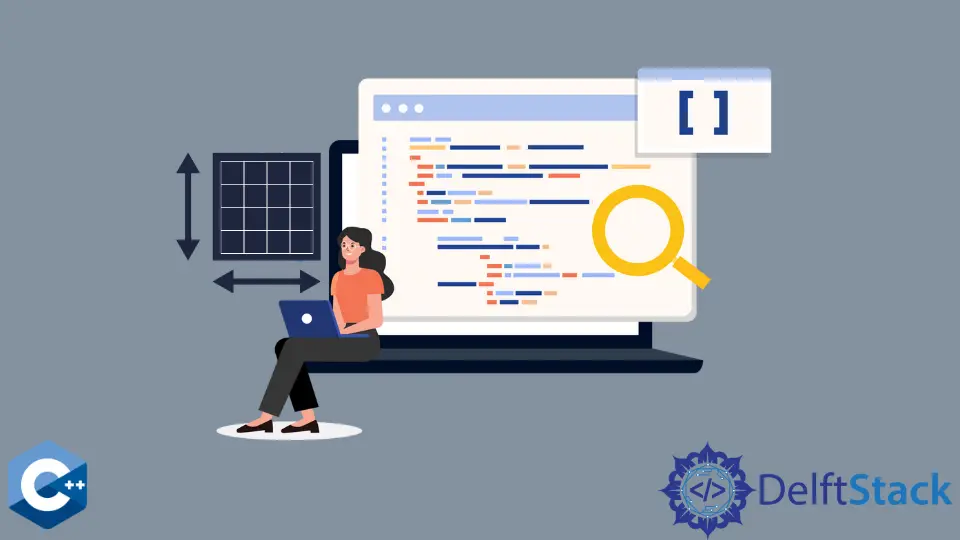
Knowing the size of an array is crucial for efficient memory allocation, accessing elements, and processing data effectively. Determining the size of an array is a fundamental task in C++.
Consider a financial application analyzing stock market data. We face an array sizing challenge to store daily closing prices for a year since the data’s length is unknown.
This article will explore essential techniques to get the array length using different C++ methods.
Use for
Loop to Calculate Array Length in C++
To dynamically calculate the size of an array in C++, we can utilize a for
loop that iterates over the elements until it reaches the end of the array. The following code snippet demonstrates how we can calculate array size using the for
loop in C++.
#include <iostream>
int main() {
int arr[] = {10, 20, 30, 40, 50}; // Example array (can be any data type)
int size = 0; // Variable to store array size
for (auto i : arr) {
size++; // Increment size for each element in the array
}
std::cout << "Array size: " << size << std::endl;
return 0;
}
Output:
The above code calculates the array arr
size using a range-based for
loop.
Initially, an example array arr
is defined with integer values. The variable size
is initialized to 0, storing the array size.
The range-based for
loop iterates through each element i
in the array arr
. The size
variable is incremented for each iteration, effectively counting the number of elements in the array.
Once all elements have been iterated, the loop terminates. Finally, the code prints the calculated array size to the console using std::cout
.
This approach provides a concise and efficient way to determine the size of an array in C++ without relying on explicit array indices or sentinel values.
Use sizeof()
Function to Calculate Array Length in C++
In C++, the sizeof()
function allows us to determine the size of an array at compile time.
Syntax:
sizeof(datatype)
The sizeof()
function takes a data type (e.g., int
, double
, char
, etc.) as its parameter and returns the size of that data type in bytes.
Unlike the for
loop method, which calculates the array size at runtime, the sizeof()
function determines it at compile-time. This provides a more efficient and straightforward approach, especially when dealing with fixed-size arrays.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
int main() {
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "array size: " << sizeof(c_array) / sizeof(c_array[0]) << endl;
return 0;
}
Output:
In the above code, we initialize an example array c_array
. By dividing the total size of the array c_array
(determined by sizeof(c_array)
) by the size of a single element in the array c_array
(determined by sizeof(c_array[0])
), we calculate the number of elements in the array.
As we can see, the output seems correct. Let’s define a separate function that does the same for us.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
int get_array_size(int arr[]) { return sizeof(arr) / sizeof(arr[0]); }
int main() {
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "array size: " << get_array_size(c_array) << endl;
return 0;
}
Output:
We get the wrong number. Using the sizeof
function is not a reliable method to solve this problem.
For the sizeof()
function to work correctly, the array should not be received as a function parameter and not allocated dynamically (on the Heap). To identify incorrect use of sizeof
, IDEs will usually lint the error-prone code sections, but you can also specify compiler flags for the warnings to be displayed during the compilation (e.g., -Wsizeof-pointer-div
or -Wall
along with gcc
).
There’s no universal way of calculating the size of C-style arrays without worrying about many edge cases, and that’s why the std::vector
class exists.
Use array.size()
Function to Calculate Array Length in C++
In C++, the array.size()
function allows us to determine the size of an array at runtime for arrays of the standard library container class std::array
.
Syntax:
std::array<datatype, size> myArray;
int size = myArray.size();
The size()
function is a member function of the std::array
class, and it returns the number of elements in the array myArray
.
Unlike the sizeof()
method, which works with built-in arrays and determines the size at compile-time, array.size()
works specifically with std::array
and calculates the size at runtime. While sizeof()
is more versatile, array.size()
provides a more convenient approach when using std::array
.
Using array.size()
can improve code readability and maintainability, especially when using standard library containers in C++.
The following code snippet demonstrates using the array.size()
function to calculate the array size in C++.
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
int main() {
array<int, 10> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "array size: " << arr.size() << endl;
return 0;
}
Output:
The above code uses the std::array
class in C++ to create an integer array named arr
with a fixed size of 10 elements. The arr.size()
function returns the size of the array, which is then printed to the console.
Use begin(arr)
and end(arr)
Functions to Calculate Array Length in C++
In C++, begin(arr)
and end(arr)
are standard library functions that allow us to calculate the size of an array (including built-in arrays and std::array
) without explicitly using loops or the size()
method.
Syntax:
int* begin(arr); // Returns a pointer to the beginning of the array
int* end(arr); // Returns a pointer to the position after the last element in
// the array
To get the array size, we can subtract the pointer returned by begin(arr)
from the pointer returned by end(arr)
.
Using end(arr) - begin(arr)
for array size calculation offers a concise and efficient alternative to array.size()
. However, it requires a good understanding of pointers and may not be as readable as the array.size()
method, which provides a more straightforward and intuitive approach when using std::array
.
Nonetheless, the end(arr) - begin(arr)
method can be helpful in scenarios where you prefer pointer arithmetic or when working with built-in arrays.
The following code snippet demonstrates using the end(arr) - begin(arr)
method to calculate array size in C++.
#include <iostream>
using std::begin;
using std::end;
int main() {
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; // Example array
int size =
end(arr) - begin(arr); // Calculate array size using pointer arithmetic
std::cout << "Array size: " << size << std::endl;
return 0;
}
Output:
In this code, we define an example integer array arr
. The array size is calculated using pointer arithmetic, where std::end(arr)
returns a pointer to the position after the last element, and std::begin(arr)
returns a pointer to the beginning of the array.
The subtraction of these two pointers yields the total number of elements in the array, which is stored in the variable size
. Finally, the calculated array size is printed to the console using std::cout
.
Use the std::size
Method to Calculate Array Length in C++
Introduced in C++17, the std::size
method is part of the <iterator>
library, and it can operate on std::array
data as well as std::vector
returning the number of elements.
Syntax:
std::size(arr);
The std::size
function takes an array as its parameter and returns the number of elements in the array.
Similar to array.size()
, std::size
provides a convenient way to determine the array size at runtime. However, std::size
offers a more generic approach that can be used with various types of arrays, making it suitable for scenarios involving both built-in arrays and standard library containers.
It also eliminates the need to explicitly use std::begin
and std::end
when calculating the array size, resulting in more concise and readable code. The following code demonstrates the usage of the std::size
function in C++ to calculate the size of both a vector
and an array
.
#include <array>
#include <iostream>
#include <vector>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::size;
using std::vector;
int main() {
vector<int> int_array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
array<int, 10> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "vector size: " << size(int_array) << endl;
cout << "array size: " << size(arr) << endl;
return 0;
}
Output:
In the above code, vector<int> int_array
and array<int, 10> arr
are initialized with ten integer elements. The size()
function, part of the C++17 standard, is then utilized to determine the number of elements in both containers.
Finally, the result is printed to the console using std::cout
.
Conclusion
In conclusion, this tutorial explored various methods to calculate array size in C++. Each approach offers its advantages depending on the specific use case.
- The
for
loop method provides flexibility for dynamically sized arrays but may introduce additional complexity. - The
sizeof()
function works efficiently with fixed-size arrays but lacks versatility for dynamic arrays. - The
array.size()
method is convenient and concise forstd::array
but doesn’t apply to built-in arrays. - The
end(arr) - begin(arr)
method works for both built-in andstd::array
but requires a good understanding of pointers. - The
std::size
function is a generic solution for all array types introduced in C++17, offering simplicity and clarity.
Selecting the appropriate method depends on the array type, performance requirements, and coding preferences. Understanding the strengths and limitations of each method equips us with the knowledge to make informed decisions when calculating array size in C++.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook