Double Ampersand in C++
-
lvalue
in C++ -
rvalue
in C++ -
Use the Double Ampersand (
&&
) forrvalue
References in C++11 - Move Semantics in C++11
- Conclusion
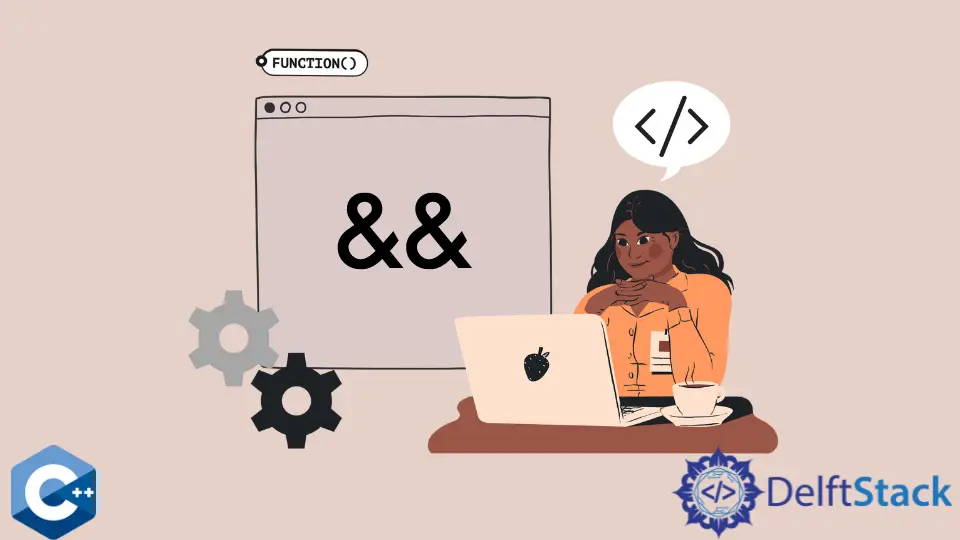
C++ 11, the version of the C++ programming language released in 2011, brought about significant improvements and additions to the language’s features and capabilities.
Among these enhancements was the introduction of the double ampersand (&&
) sign, also known as the rvalue
reference or move semantics.
This addition has had a profound impact on how developers handle memory management and optimize their code for better performance and efficiency.
To understand the double ampersand (&&
), we need to know lvalues
and rvalues
in C++. Let’s understand these terms in the following subsections.
lvalue
in C++
An lvalue
is essentially an object or variable that can occupy an identifiable space within the computer’s main memory or RAM. Consider the following code snippet as an example:
int number = 10;
In this code, number
is an lvalue
as it represents a variable with a memory location. We can obtain its address by creating a pointer like so:
// this is a pointer to a variable
int *ptr_Number = &number;
// Here, we'll get the address of a variable in memory;
cout << ptr_Number << endl;
// object example
Here, ptr_Number
now holds the address of the variable number
, showcasing the characteristics of an lvalue
.
rvalue
in C++
On the other hand, an rvalue
represents an object or variable that doesn’t possess an identifiable space in memory; it’s considered a temporary value.
Let’s revisit the previous example:
int number = 10;
In this case, number
is an lvalue
, but 10 is an rvalue
. Attempting to obtain the address of the value 10 directly would result in an error, as shown below:
// int *ptr_10 = &10;
//(error) expression must be an lvalue
Use the Double Ampersand (&&
) for rvalue
References in C++11
As we have discussed above, variables typically fall into one of two categories: lvalues
and rvalues
. An lvalue
represents an object that has a specific memory location, meaning you can take its address, while an rvalue
represents a temporary value that does not have a memory location.
C++ 11 introduced the concept of rvalue
references, denoted by the double ampersand (&&
), to allow programmers to differentiate between these two types of expressions.
An rvalue
reference can bind to an rvalue
, providing a powerful mechanism to move resources and improve performance. It allows for efficient resource management by enabling the transfer of ownership or the “move” of resources from one object to another without creating unnecessary copies.
This concept becomes evident when comparing functions that utilize a single ampersand and those that use a double ampersand. Let’s consider two methods: one employing a single ampersand and another utilizing a double ampersand.
When attempting to pass an lvalue
to a function using a single ampersand, an error occurs. The primary purpose of the double ampersand is to cater to rvalues, allowing their successful use within functions.
int add(int &a, int &b) { return a + b; }
int add_(int &&a, int &&b) { return a + b; }
int main() {
int num1 = 10;
int num2 = 30;
int sum = add(num1, num2);
cout << sum << endl;
// It will give an error if we pass rvalues to that function
// int sum_1= add(10,30);
// (error) initial value of reference to non-const must be an lvalue
int sum_1 = add_(10, 30);
cout << endl;
}
In this example code, the add
function uses single ampersands to accept lvalue
references, taking two integer references as parameters and returning their sum.
On the other hand, the add_
function uses double ampersands to accept rvalue
references, also taking two integers, but in this case, they are rvalue
references.
The main
function showcases these concepts in action. It begins by initializing two variables, num1
and num2
, which are lvalues
.
The add
function is then called with these lvalues
, successfully computing their sum. However, attempting to call add
with literals (rvalues
) like 10 and 30 results in a compilation error, as the function expects lvalues
.
In contrast, the add_
function is designed to accept rvalues
, and it is called with the literals 10 and 30, demonstrating the utilization of double ampersands to handle rvalues
appropriately.
Move Semantics in C++11
The move semantics in C++ 11 are closely related to rvalue
references and play a crucial role in optimizing memory management and improving performance.
When an object is an rvalue
, it’s often temporary or about to go out of scope. In such cases, instead of creating a new copy when assigning the value to another variable, C++ 11 allows us to “move” the resources associated with the rvalue
to the new variable.
Using move semantics can significantly reduce the overhead associated with creating unnecessary copies and allocations, ultimately leading to faster code execution and better memory management.
Let’s have an example that leverages rvalue
references and move semantics.
#include <iostream>
#include <utility>
class MyVector {
public:
MyVector(size_t size) : size_(size), data_(new int[size]) {}
MyVector(MyVector&& other) noexcept {
size_ = other.size_;
data_ = other.data_;
other.size_ = 0;
other.data_ = nullptr;
}
MyVector& operator=(MyVector&& other) noexcept {
if (this != &other) {
delete[] data_;
size_ = other.size_;
data_ = other.data_;
other.size_ = 0;
other.data_ = nullptr;
}
return *this;
}
~MyVector() { delete[] data_; }
private:
size_t size_;
int* data_;
};
int main() {
MyVector vec1(10);
MyVector vec2 = std::move(vec1);
MyVector vec3(5);
vec3 = std::move(vec2);
return 0;
}
Let’s break down each essential part of the code.
First, we introduced a custom class called MyVector
, resembling a simplified vector-like container that holds integers. In this class, there’s a constructor named MyVector(size_t size)
responsible for creating instances of MyVector
by allocating memory for a specific number of integers defined by the size
parameter.
Here, the move constructor MyVector(MyVector&& other)
is a special function designed to take ownership of resources from another MyVector
object, efficiently transferring its size and data. Similarly, the move assignment operator MyVector& operator=(MyVector&& other)
allows transferring ownership when assigning an rvalue
to an existing MyVector
.
Properly deallocating memory is ensured through the destructor ~MyVector()
, which is called when a MyVector
object goes out of scope, preventing memory leaks.
The main
function demonstrates the use of move semantics, creating instances of MyVector
and showcasing how to move resources from one object to another, enhancing resource management and overall performance in C++ programs.
The introduction of rvalue
references and move semantics in C++11 has brought several benefits to the language and its users, including:
- Efficient Resource Management: Move semantics allow for the efficient management of resources, avoiding unnecessary copies and allocations.
- Improved Performance: By reducing unnecessary copying, move semantics contribute to faster code execution and improved program performance.
- Reduced Memory Overhead: Minimizing memory allocations and deallocations leads to lower memory overhead, enhancing overall system efficiency.
Conclusion
The double ampersand (&&
) sign in C++11 represents rvalue
references and introduces move semantics to the language. This feature enhances memory management and performance by allowing the efficient transfer of resources from temporary or soon-to-be-discarded objects to new variables.
By embracing move semantics, C++ programmers can significantly improve the efficiency of their code while ensuring optimal resource utilization.
Understanding and utilizing rvalue
references and move semantics is a crucial skill for any C++ developer seeking to write high-performance, resource-efficient code.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn