C++ のダブルアンパサンド
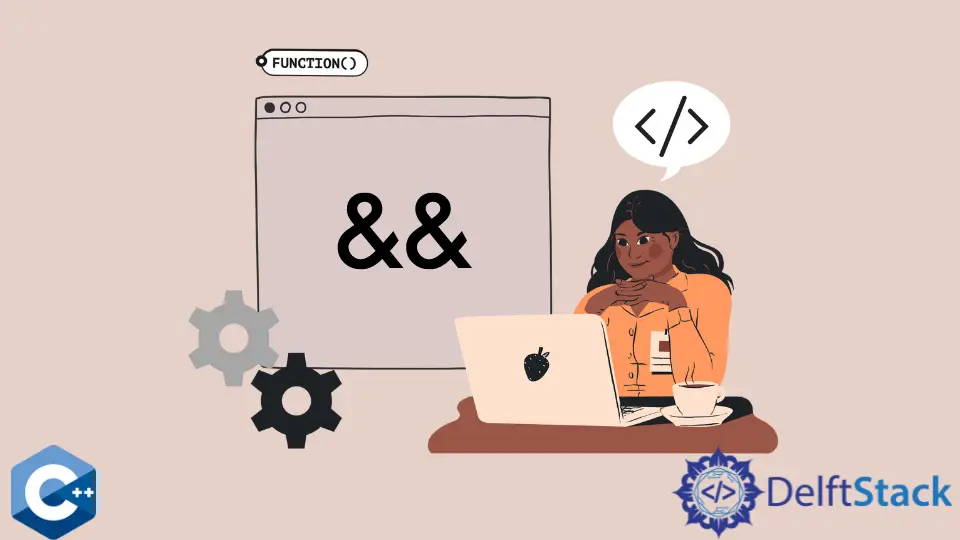
このガイドでは、C++ でのダブルアンパサンド記号について説明します。それは実際には C++11 の概念に含まれています。
変数の宣言でダブルアンパサンド(&&
)を見たことがあるかもしれません。この概念のあらゆる側面を理解するには、C++ の基本のいくつかを理解する必要があります。
C++ のダブルアンパサンド
ダブルアンパサンド(&&
)を理解するには、C++ の lvalues
と rvalues
を知る必要があります。次のサブセクションでこれらの用語を理解しましょう。
C++ の lvalue
lvalue
は、メインメモリまたは RAM 内の識別可能なスペースを占有できるオブジェクトまたは変数と見なされます。たとえば、次のコードを見てください。
int number = 10;
上記のコードでは、number
は lvalue
と見なされます。ポインタにすることで、その場所/アドレスを取得します。
// this is pointer to varible
int *ptr_Number = &number;
// here we'll get the address of variable in memory;
cout << ptr_Number << endl;
// object example
C++ の rvalue
rvalue
は、メモリ内に識別可能なスペースがないオブジェクトまたは変数です。また、一時的な値と見なされます。
次のコードを見てください。
int number = 10;
上記のコードでは、number
は lvalue
ですが、10
は rvalue
です。数字の 10
アドレスを取得しようとすると、エラーが発生します。
// int *ptr_10 = &10;
//(error) expression must be an lvalue
C++ 11 では、二重アンパサンド記号は rvalue
を指します。それでは、2つの方法を考えてみましょう。
1つはシングルアンパサンド記号で、もう 1つはダブルアンパサンド記号です。
lvalue
を関数に渡そうとすると、エラーが発生します。したがって、基本的に、ダブルアンパサンドの主な目的は rvalues
を参照することです。
// single ampersand sign is used to refer lvalue
int add(int &a, int &b) { return a + b; }
// double ampersand sign is used to refer rvalue
int add_(int &&a, int &&b) { return a + b; }
int main() {
// here we pass lvalue to the function
int num1 = 10;
int num2 = 30;
int sum = add(num1, num2);
cout << sum << endl;
// it will give the error if we pass the rvalues to that function
// int sum_1= add(10,30);
//(error) initial value of reference to non-const must be an lvalue
// c++11 provides the facility of passing rvalues by using double ampersand
// sign
int sum_1 = add_(10, 30);
cout << endl;
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn