C++ 中的雙與號
Haider Ali
2023年10月12日
C++
C++ Reference
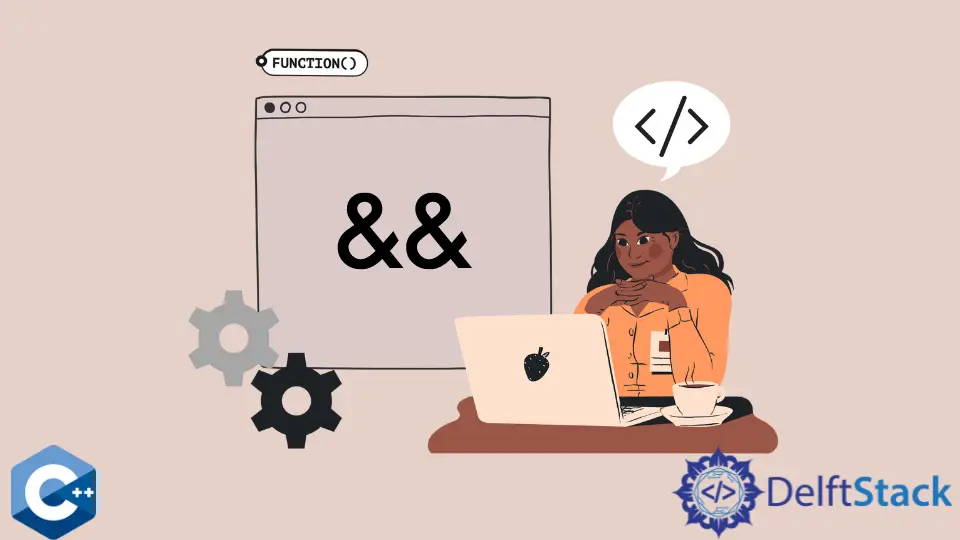
本指南將討論 C++ 中的雙 & 符號。它實際上是在 C++11 的概念中。
你可能已經在宣告變數時看到過雙與號 (&&
)。要理解這個概念的各個方面,我們需要了解 C++ 的一些基礎知識。
C++ 中的雙與號
要理解雙與號 (&&
),我們需要了解 C++ 中的 lvalues
和 rvalues
。讓我們在以下小節中理解這些術語。
C++ 中的左值
lvalue
被認為是一個物件或變數,可以佔用主儲存器或 RAM 中的可識別空間。例如,看看下面的程式碼。
int number = 10;
number
在上面的程式碼中被認為是一個 lvalue
。我們通過將其設為指標來獲取其位置/地址。
// this is pointer to varible
int *ptr_Number = &number;
// here we'll get the address of variable in memory;
cout << ptr_Number << endl;
// object example
C++ 中的 rvalue
rvalue
是在記憶體中沒有任何可識別空間的物件或變數。它也被認為是一個臨時值。
看看下面的程式碼。
int number = 10;
number
是上面程式碼中的 lvalue
,但 10
是 rvalue
。如果我們嘗試獲取數字 10
地址,我們會得到一個錯誤。
// int *ptr_10 = &10;
//(error) expression must be an lvalue
在 C++11 中,雙 & 符號指的是 rvalue
。現在,讓我們考慮兩種方法。
一個帶有單&符號,另一個帶有雙&符號。
如果你嘗試將 lvalue
傳遞給函式,你將收到錯誤訊息。所以基本上,雙&符號的主要目的是引用 rvalues
。
// single ampersand sign is used to refer lvalue
int add(int &a, int &b) { return a + b; }
// double ampersand sign is used to refer rvalue
int add_(int &&a, int &&b) { return a + b; }
int main() {
// here we pass lvalue to the function
int num1 = 10;
int num2 = 30;
int sum = add(num1, num2);
cout << sum << endl;
// it will give the error if we pass the rvalues to that function
// int sum_1= add(10,30);
//(error) initial value of reference to non-const must be an lvalue
// c++11 provides the facility of passing rvalues by using double ampersand
// sign
int sum_1 = add_(10, 30);
cout << endl;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn