C++의 이중 앰퍼샌드
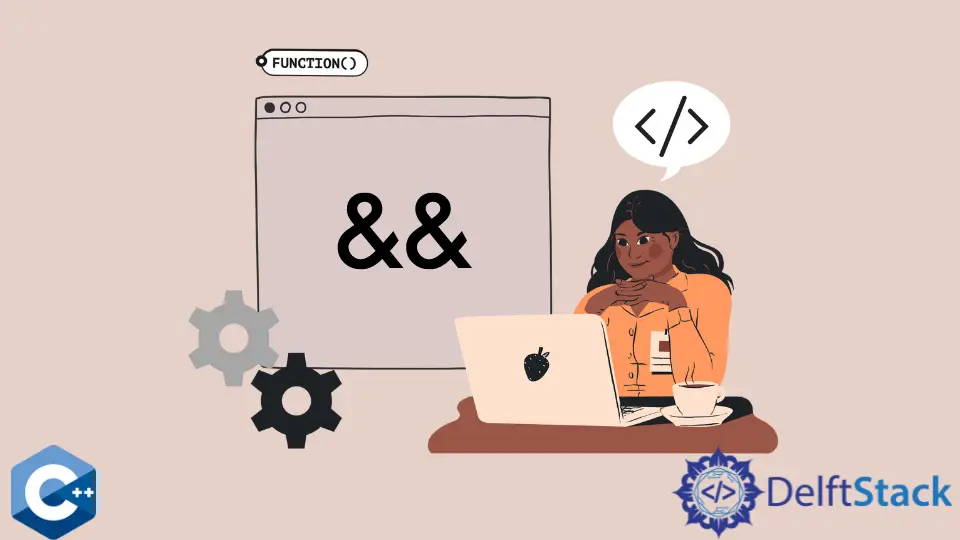
이 가이드에서는 C++의 이중 앰퍼샌드 기호에 대해 설명합니다. 실제로 C++11의 개념에 있습니다.
변수를 선언할 때 이중 앰퍼샌드(&&
)를 본 적이 있을 것입니다. 이 개념의 모든 측면을 이해하려면 C++의 몇 가지 기본 사항을 이해해야 합니다.
C++의 이중 앰퍼샌드
이중 앰퍼샌드(&&
)를 이해하려면 C++에서 lvalues
와 rvalues
를 알아야 합니다. 다음 하위 섹션에서 이러한 용어를 이해합시다.
C++의 lvalue
lvalue
는 주 메모리 또는 RAM에서 식별 가능한 공간을 차지할 수 있는 개체 또는 변수로 간주됩니다. 예를 들어 다음 코드를 살펴보십시오.
int number = 10;
number
는 위의 코드에서 lvalue
로 간주됩니다. 포인터를 만들어 위치/주소를 얻습니다.
// this is pointer to varible
int *ptr_Number = &number;
// here we'll get the address of variable in memory;
cout << ptr_Number << endl;
// object example
C++의 rvalue
rvalue
는 메모리에 식별 가능한 공간이 없는 개체 또는 변수입니다. 또한 일시적인 가치로 간주됩니다.
다음 코드를 살펴보십시오.
int number = 10;
위 코드에서 ‘숫자’는 ’lvalue’이지만 ‘10’은 ‘rvalue’입니다. 숫자 ‘10’ 주소를 얻으려고 하면 오류가 발생합니다.
// int *ptr_10 = &10;
//(error) expression must be an lvalue
C++11에서 이중 앰퍼샌드 기호는 rvalue
를 나타냅니다. 이제 두 가지 방법을 살펴보겠습니다.
하나는 단일 앰퍼샌드 기호이고 다른 하나는 이중 앰퍼샌드 기호입니다.
lvalue
를 함수에 전달하려고 하면 오류가 발생합니다. 따라서 기본적으로 이중 앰퍼샌드의 주요 목적은 rvalues
를 참조하는 것입니다.
// single ampersand sign is used to refer lvalue
int add(int &a, int &b) { return a + b; }
// double ampersand sign is used to refer rvalue
int add_(int &&a, int &&b) { return a + b; }
int main() {
// here we pass lvalue to the function
int num1 = 10;
int num2 = 30;
int sum = add(num1, num2);
cout << sum << endl;
// it will give the error if we pass the rvalues to that function
// int sum_1= add(10,30);
//(error) initial value of reference to non-const must be an lvalue
// c++11 provides the facility of passing rvalues by using double ampersand
// sign
int sum_1 = add_(10, 30);
cout << endl;
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn