How to Use Default Parameters for Functions in C++
- Understanding Default Parameters in C++
- Benefits of Using Default Parameters
- Best Practices for Default Parameters
- Conclusion
- FAQ
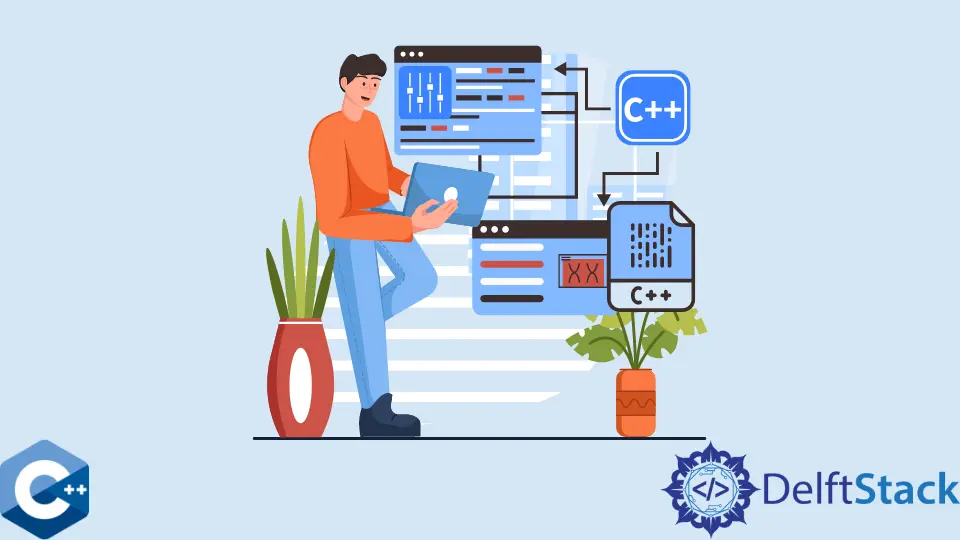
In the world of C++, functions can become quite complex, especially when it comes to handling multiple parameters. Fortunately, C++ offers a powerful feature known as default parameters. This feature allows developers to specify default values for function parameters, making functions more flexible and easier to use.
In this article, we will explore how to effectively use default parameters in C++. We will cover the syntax, benefits, and provide practical examples to illustrate how default parameters can streamline your code. Whether you’re a beginner or an experienced C++ programmer, understanding default parameters can enhance your coding skills and improve the readability of your programs.
Understanding Default Parameters in C++
Default parameters allow you to define a value for a function parameter if an argument is not provided during a function call. This means you can create more versatile functions without overloading them or creating multiple versions. The syntax for defining default parameters is straightforward: you simply assign a value to a parameter in the function declaration.
Here’s a simple example to illustrate this:
#include <iostream>
using namespace std;
void greet(string name, string greeting = "Hello") {
cout << greeting << ", " << name << "!" << endl;
}
int main() {
greet("Alice");
greet("Bob", "Hi");
return 0;
}
Output:
Hello, Alice!
Hi, Bob!
In this example, the function greet
takes two parameters: name
and greeting
. The greeting
parameter has a default value of “Hello”. When we call greet("Alice")
, it uses the default greeting, while greet("Bob", "Hi")
specifies a different greeting. This flexibility simplifies function calls and improves code readability.
Benefits of Using Default Parameters
Using default parameters in C++ comes with several advantages that can significantly enhance your programming experience.
-
Reduced Code Duplication: By using default parameters, you can avoid creating multiple overloaded functions. This not only saves time but also reduces the chance of introducing bugs in your code.
-
Improved Readability: Functions with default parameters can often be called with fewer arguments, making the code cleaner and easier to read. This is particularly helpful when working with functions that have many parameters.
-
Enhanced Flexibility: Default parameters provide the flexibility to call functions with varying numbers of arguments. This is especially useful in scenarios where certain parameters are optional.
Here’s another example that demonstrates these benefits:
#include <iostream>
using namespace std;
void displayInfo(string name, int age = 18, string country = "USA") {
cout << "Name: " << name << ", Age: " << age << ", Country: " << country << endl;
}
int main() {
displayInfo("John");
displayInfo("Jane", 25);
displayInfo("Doe", 30, "Canada");
return 0;
}
Output:
Name: John, Age: 18, Country: USA
Name: Jane, Age: 25, Country: USA
Name: Doe, Age: 30, Country: Canada
In this example, the function displayInfo
has two parameters with default values. This allows for flexibility in how we call the function. The first call uses all default values, the second overrides the age, and the third provides all parameters. This versatility makes it easier to manage function calls in your code.
Best Practices for Default Parameters
While default parameters are a powerful feature, there are some best practices to keep in mind to ensure your code remains clean and maintainable.
-
Place Default Parameters at the End: Always place parameters with default values at the end of the parameter list. This ensures that the function can be called with varying numbers of arguments without confusion.
-
Avoid Using Default Parameters with References: If a parameter is a reference type, be cautious about using default values. This can lead to unexpected behavior if not handled properly.
-
Keep Default Values Consistent: When using default parameters across multiple functions, try to keep default values consistent. This helps maintain a uniform approach and makes your code easier to follow.
Here’s an example that incorporates these best practices:
#include <iostream>
using namespace std;
void calculateArea(double length, double width = 1.0) {
cout << "Area: " << length * width << endl;
}
int main() {
calculateArea(5.0);
calculateArea(5.0, 2.0);
return 0;
}
Output:
Area: 5
Area: 10
In this example, the calculateArea
function has a default width of 1.0. By placing the default parameter at the end, we ensure that the function can be called with just the length or both length and width. This maintains clarity and functionality.
Conclusion
Default parameters in C++ are a valuable tool that can simplify your code by reducing duplication, improving readability, and enhancing flexibility. By understanding how to implement and leverage default parameters, you can write cleaner and more efficient functions. Remember to follow best practices to ensure your code remains maintainable and easy to understand. As you continue to explore C++, incorporating default parameters into your programming toolkit will undoubtedly elevate your coding skills.
FAQ
-
What are default parameters in C++?
Default parameters are values that can be assigned to function parameters, allowing the function to be called with fewer arguments. -
Can default parameters be used with all data types?
Yes, default parameters can be used with any data type, including built-in types and user-defined types. -
What happens if I provide an argument for a parameter with a default value?
If you provide an argument for a parameter with a default value, the provided argument will be used instead of the default.
-
Are default parameters allowed in function overloading?
Yes, default parameters can be used in overloaded functions, but care must be taken to avoid ambiguity in function calls. -
Can I change the default value of a parameter in a derived class?
Yes, you can change the default value of a parameter in a derived class, but it is often better to maintain consistency for clarity.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook