How to Create Vector of Pointers in C++
- Understanding Vectors and Pointers
- Creating a Vector of Pointers
- Managing Memory Efficiently
- Using Smart Pointers
- Conclusion
- FAQ
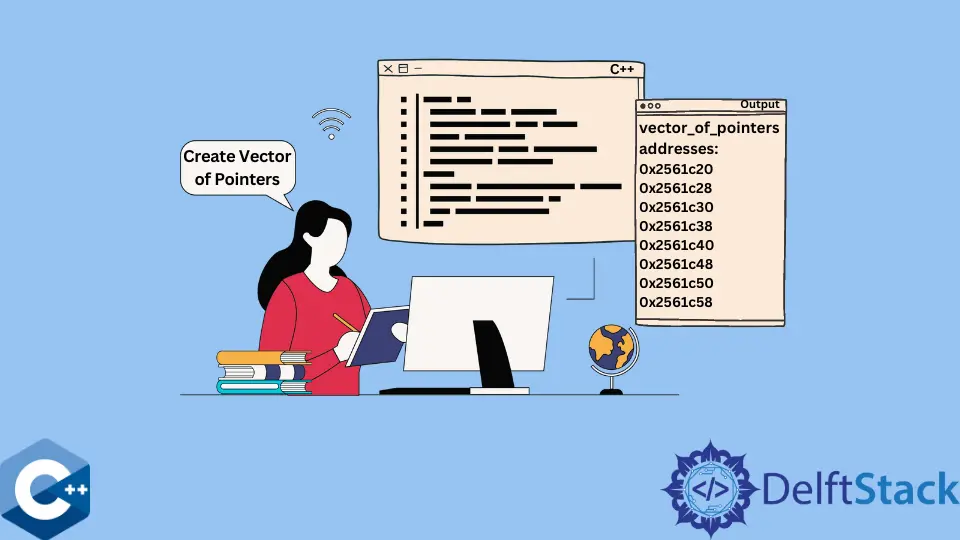
Creating a vector of pointers in C++ can be a powerful technique for managing dynamic memory and handling collections of objects. Whether you’re developing complex applications or simply want to grasp the fundamentals of C++, understanding how to work with vectors and pointers is essential.
In this article, we will explore the step-by-step process of creating a vector of pointers, including code examples and detailed explanations to help you grasp the concept thoroughly. By the end, you will have a solid foundation for utilizing vectors of pointers in your own C++ projects.
Understanding Vectors and Pointers
Before diving into the code, let’s clarify what vectors and pointers are. A vector in C++ is a dynamic array that can grow and shrink in size, making it a flexible choice for storing collections of data. Pointers, on the other hand, are variables that hold memory addresses of other variables. When combined, vectors of pointers allow you to create a dynamic collection of objects while efficiently managing memory.
Creating a Vector of Pointers
To create a vector of pointers, you first need to include the necessary headers and then declare a vector that stores pointers to your desired data type. Here’s a simple example demonstrating how to create a vector of pointers to integers.
#include <iostream>
#include <vector>
int main() {
std::vector<int*> vec;
for (int i = 0; i < 5; ++i) {
int* ptr = new int(i);
vec.push_back(ptr);
}
for (int* ptr : vec) {
std::cout << *ptr << " ";
}
for (int* ptr : vec) {
delete ptr;
}
return 0;
}
Output:
0 1 2 3 4
In this code, we start by including the necessary headers and declaring a vector named vec
that holds pointers to integers. We then use a loop to allocate memory for five integers dynamically. Each pointer is stored in the vector using the push_back
method. After displaying the values pointed to by the pointers, we ensure to free the allocated memory using delete
to prevent memory leaks.
Managing Memory Efficiently
When working with vectors of pointers, managing memory effectively is crucial to avoid leaks and ensure optimal performance. In the previous example, we used new
to allocate memory for integers and delete
to free that memory. However, it is essential to ensure that every allocated pointer is deleted once it is no longer needed.
Here’s another example that demonstrates how to create a vector of pointers to objects of a custom class.
#include <iostream>
#include <vector>
class MyClass {
public:
MyClass(int val) : value(val) {}
void display() const {
std::cout << value << " ";
}
private:
int value;
};
int main() {
std::vector<MyClass*> vec;
for (int i = 0; i < 5; ++i) {
MyClass* obj = new MyClass(i);
vec.push_back(obj);
}
for (MyClass* obj : vec) {
obj->display();
}
for (MyClass* obj : vec) {
delete obj;
}
return 0;
}
Output:
0 1 2 3 4
In this example, we define a class MyClass
that has a constructor and a method to display its value. We create a vector of pointers to MyClass
objects, allocate memory for each object, and store the pointers in the vector. After displaying the values, we again ensure to delete each object to prevent memory leaks.
Using Smart Pointers
While raw pointers are useful, C++ offers smart pointers that automatically manage memory for you, reducing the risk of memory leaks and dangling pointers. The std::unique_ptr
and std::shared_ptr
are two common smart pointers that can be used with vectors. Here’s how to use std::unique_ptr
with a vector.
#include <iostream>
#include <vector>
#include <memory>
class MyClass {
public:
MyClass(int val) : value(val) {}
void display() const {
std::cout << value << " ";
}
private:
int value;
};
int main() {
std::vector<std::unique_ptr<MyClass>> vec;
for (int i = 0; i < 5; ++i) {
vec.push_back(std::make_unique<MyClass>(i));
}
for (const auto& obj : vec) {
obj->display();
}
return 0;
}
Output:
0 1 2 3 4
In this example, we use std::unique_ptr
to manage the memory of MyClass
objects. The std::make_unique
function simplifies object creation and ensures that memory is automatically released when the vector goes out of scope. This approach eliminates the need for manual memory management, making your code safer and cleaner.
Conclusion
Creating a vector of pointers in C++ is a valuable skill that enhances your ability to manage dynamic memory and collections of objects. By understanding the differences between raw pointers and smart pointers, you can write more efficient and safer code. Whether you’re working with simple data types or complex classes, mastering vectors of pointers will significantly improve your programming capabilities. Embrace these techniques, and you’ll be well on your way to writing robust C++ applications.
FAQ
-
What is a vector in C++?
A vector is a dynamic array that can change size during runtime, allowing for flexible storage of elements. -
Why use pointers with vectors?
Using pointers with vectors allows for efficient memory management and the ability to store dynamically allocated objects. -
What are smart pointers in C++?
Smart pointers are objects that manage the memory of dynamically allocated objects, automatically releasing memory when it is no longer needed. -
How do I avoid memory leaks when using vectors of pointers?
Always ensure to delete any dynamically allocated memory after use or consider using smart pointers to manage memory automatically. -
Can I store different types of pointers in a single vector?
No, a vector can only store pointers of a single type. If you need to store different types, consider using a base class pointer or a variant type.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook